I want to build a strategy that buys at the Market Open and then sells the following Market Open (but also then rebuys X number of symbols at the same Market Open). I've tried playing with pos sizer and boosting margin to 2x to account for this but it always skips a day in between trades. Is there any other way of getting around this?
Rename
There's nothing really that needs to be gotten around, you just need to ensure the strategy is coded correctly to do what you want. Here's a simple proof of concept that buys sometimes at market open, sells at market open, but also sometimes buys again at market open on that same bar that's sold.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //Initialize public override void Initialize(BarHistory bars) { } //Execute public override void Execute(BarHistory bars, int idx) { //sell at market open foreach(Position pos in OpenPositions) ClosePosition(pos, OrderType.Market); //don't buy always if (rnd.NextDouble() > 0.2) return; //buy at market open PlaceTrade(bars, TransactionType.Buy, OrderType.Market); //and sometimes buy again at market open if (rnd.NextDouble() > 0.8) PlaceTrade(bars, TransactionType.Buy, OrderType.Market); } //private members private Random rnd = new Random(); } }
Thanks glitch. What if I want to guarantee say 10 trades to buy/sell at each market open?
Buy 10 stocks market open, sell them next market open and buy 10 additional that same open? The symbols are all picked from weighting a select group of symbols.
Buy 10 stocks market open, sell them next market open and buy 10 additional that same open? The symbols are all picked from weighting a select group of symbols.
Of course, you could do that. it sounds like the selections could be done in PreExecute or you could assign a transaction weight and let position sizing drive the limit of 10.
I currently am using position sizing (max 10) and am still having this issue. Can you post a brief snip of code to make sure I am doing it correctly? Thanks.
The snippet is above in Post #1. If yours doesn't use that pattern, maybe you should post what you're doing so we can tell what's happening. It sounds to me like you're using the basic single position logic that processes either entries or exits on a given bar. You need to be doing both on the same bar (like in the snippet above).
Thanks Cone, I am using single position logic, I will recode to the first post. I will update you if I have any issues. Thank you both!
The logic works with Market orders but seems to fail with MOC orders. Do MOC orders act differently?
No difference, I changed by code above to all MarketClose, and here's an example of a sell and buy on the same bar ...
This appears to only work when backtesting one symbol, and not a portfolio (buying and selling on same MarketClose). I used the sample code, changed to MarketClose and do not get any repeat buy/sells at MarketClose, it always skips a day.
Position sizing too high? Really it works.
Give this a try.. how many trades do you get on the second to last bar?
Give this a try.. how many trades do you get on the second to last bar?
CODE:
//Execute public override void Execute(BarHistory bars, int idx) { if (idx == bars.Count - 3) { for (int n = 0; n < 10; n++) { PlaceTrade(bars, TransactionType.Buy, OrderType.MarketClose); } } }
Using 20% Equity.
Max Open Post: 5
The rest are set to 0.
Margin 1.2
SP 100 dataset (wealthdata)
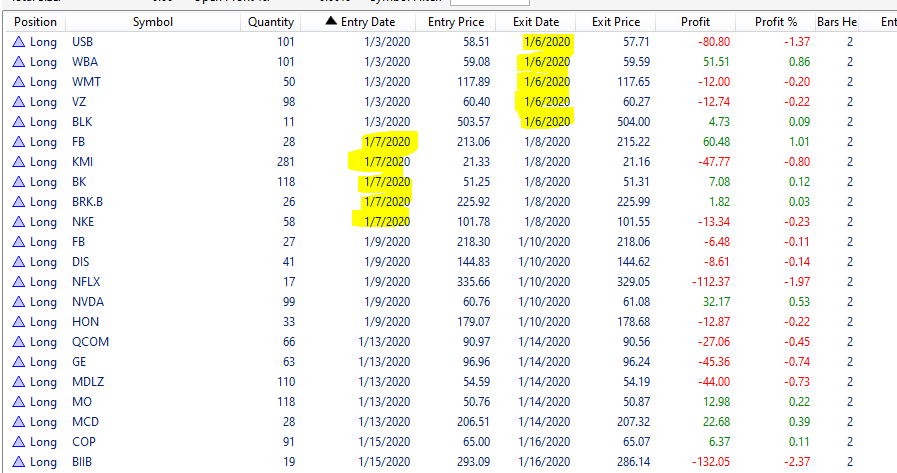
Max Open Post: 5
The rest are set to 0.
Margin 1.2
SP 100 dataset (wealthdata)
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //Initialize public override void Initialize(BarHistory bars) { } //Execute public override void Execute(BarHistory bars, int idx) { foreach(Position pos in OpenPositions) ClosePosition(pos, OrderType.MarketClose); PlaceTrade(bars, TransactionType.Buy, OrderType.MarketClose); } //private members private Random rnd = new Random(); } }
Re: Post #11. Yes, this seems to be the case here too with MarketClose orders - as opposed to Market orders which do not skip a day. Looks like an issue to me.
On a DataSet, yes, I see that the results are inconsistent. With margin applied, this should work!
I don't see any issue, besides maybe a misunderstanding. You cannot buy and sell at MarketClose on the same bar, it doesn't make sense.
You can sell on MarketClose same-bar in a DataSet backtest, but you have to place the sell orders after the buy orders in the Strategy. Using a loop going over OpenPositions like in the example code will not work because the Positions aren't even created yet.
Here is a minimal example:
You can sell on MarketClose same-bar in a DataSet backtest, but you have to place the sell orders after the buy orders in the Strategy. Using a loop going over OpenPositions like in the example code will not work because the Positions aren't even created yet.
Here is a minimal example:
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //Initialize public override void Initialize(BarHistory bars) { } //Execute public override void Execute(BarHistory bars, int idx) { //don't buy always if (rnd.NextDouble() > 0.2) return; //buy at market open PlaceTrade(bars, TransactionType.Buy, OrderType.Market); //and sometimes buy again at market open if (rnd.NextDouble() > 0.8) PlaceTrade(bars, TransactionType.Buy, OrderType.Market); //sell at market close PlaceTrade(bars, TransactionType.Sell, OrderType.MarketClose); PlaceTrade(bars, TransactionType.Sell, OrderType.MarketClose); } //private members private Random rnd = new Random(); } }
QUOTE:
I don't see any issue, besides maybe a misunderstanding. You cannot buy and sell at MarketClose on the same bar, it doesn't make sense.
It was the lack of morning coffee on my part 😂🤦♂️ Of course you're right.
So if I wanted to create a rotational strategy that sells positions at market close (based on previous day data) and then buy additional symbols that same market close (based on yesterdays data), it cannot be done? Similar to a rotational strategy that sells/buys same market open.
Sure it can be done, but the same position cannot be bought and sold on the same bar using marketclose for both.
I don't look at the computer before sipping a cafe'. I have no excuse.
The example provided above #11 is using a dataset, not the same symbol. It skips a day in between.
Yep. It will skip a day because it's using ClosePosition. And then, later on in the code, it's using PlaceTrade to enter an order.
A Position is not created until the next bar, so it makes sense that it will skip a day.
A Position is not created until the next bar, so it makes sense that it will skip a day.
Just use the rotation script I wrote for you and change the orders to MarkeClose.
https://www.wealth-lab.com/Discussion/Rotational-Strategy-Stocks-by-Sector-7316
https://www.wealth-lab.com/Discussion/Rotational-Strategy-Stocks-by-Sector-7316
So, bottom line, is there "currently" any method to Buy and Sell the same position on the same day with WL7? I'm gathering after reading this thread that there isn't. But I could be missing something.
QUOTE:
is there "currently" any method to Buy and Sell the same position on the same day with WL7?
That certainly exceeds any existing backtesting software's capability as of today! 😄
CODE:
if (idx == bars.Count-2) { PlaceTrade(bars, TransactionType.Buy, OrderType.Market); PlaceTrade(bars, TransactionType.Sell, OrderType.MarketClose); }
It is possible to do this (Buy and Sell on the same day) with WL6 because I'm using "pseudo Buys" to buy positions the real portfolio missed selling the day before, so WL6 could have another opportunity to sell these orphaned positions again to resynchronize the real portfolio with the WL6 simulation. And this has been working for 4 years on WL6 very nicely. And it has saved me a lot of money.
This is a continuation of an earlier thread. https://www.wealth-lab.com/Discussion/Selling-a-quot-ghost-quot-position-and-resync-39-ing-a-portfolio-with-the-WL7-simulation-5541 I have a solution to that problem, but I need to post a Feature Request to solve it. I just don't want to clutter this Feature Request with all the others awaiting to be serviced right now.
Unless everyone is anxious to see what this Feature Request looks like.... I've been thinking about it for awhile.
CODE:But that code above is a day late. How do I make the same code work for (bars.Count-1) like it does now for WL6?
if (idx == bars.Count-2) { PlaceTrade(bars, TransactionType.Buy, OrderType.Market); PlaceTrade(bars, TransactionType.Sell, OrderType.MarketClose); }
This is a continuation of an earlier thread. https://www.wealth-lab.com/Discussion/Selling-a-quot-ghost-quot-position-and-resync-39-ing-a-portfolio-with-the-WL7-simulation-5541 I have a solution to that problem, but I need to post a Feature Request to solve it. I just don't want to clutter this Feature Request with all the others awaiting to be serviced right now.
Unless everyone is anxious to see what this Feature Request looks like.... I've been thinking about it for awhile.
It's certainly possible to buy and sell on the same bar, the same position, as demonstrated above in my sample code.
What doesn't make sense, and isn't supported, is buying at MarketClose, and then selling that position on the same bar.
What doesn't make sense, and isn't supported, is buying at MarketClose, and then selling that position on the same bar.
QUOTE:
It's certainly possible to buy and sell on the same bar,
That seems to work, but for the off-the-Chart bar there's a problem. Let's take this problem up in the other thread cited above where it's more appropriate. https://www.wealth-lab.com/Discussion/Selling-a-quot-ghost-quot-position-and-resync-39-ing-a-portfolio-with-the-WL7-simulation-5541 See Reply# 16.
QUOTE:Really, it's not a problem. We solved it 2 ways (and when I say "we" I mean Glitch), but the preferred way is UserStrategyBase > AssignAutoStopTargetPrices.
the off-the-Chart bar there's a problem.
Edit: Maybe we're not talking about the same issue. This thread is about buying and selling on the same date. AssignAutoStopTargetPrices allows you to enter a trade and sell it on the same bar - in backtesting and live.
QUOTE:
Edit: Maybe we're not talking about the same issue. This thread is about buying and selling on the same date. AssignAutoStopTargetPrices allows you to enter a trade and sell it on the same bar
No, it's not the same issue. ResyncPort() is about exception handling of a missed sell on an earlier bar, so ResyncPort() is trying to sell this orphaned position after the fact. The problem is the WL backtester doesn't let you sell a "real" portfolio position the WL simulation doesn't currently own.
One "wacky" workaround to this predicament is to Buy the orphaned position back, then Sell it all on the same bar. But that trick only works with WL6, not WL7. Details are discussed in the ResyncPort() thread. ResyncPort() is not about normal program flow. It's about correcting a portfolio synchronization problem after the fact. Let's keep this thread about "normal" program flow.
WL7 does let you do that. But you have to give the Transaciton a volume. Here is a minimal working example that will issue a sell signal.
CODE:
using WealthLab.Backtest; using WealthLab.Core; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //Initialize public override void Initialize(BarHistory bars) { } //Execute public override void Execute(BarHistory bars, int idx) { if (idx == bars.Count - 1) { Transaction t = PlaceTrade(bars, TransactionType.Sell, OrderType.Market); t.Quantity = 123; } } } }
Your Response
Post
Edit Post
Login is required