Can the Trend Line Block be applied to an indicator? It would be a nice feature.
Rename
Certainly possible, but need a feature request for that.
It'll take some re-arranging, but I think I can knock this out pretty quickly. Look for it in Build 31.
Cone Thank you. There is one Strategy in the community called Trendline Break CCI but only works for CCI.
Okay, but I've been given some higher priorities, so let's call it off for Build 31 for now.
Ok. No problem in the meantime I'm going to look at the Trendline Break CCI C# code and figure out maybe I can doctor it for other indicators like RSI etc.
I can't find the Trendline Break CCI strategy. I looked in the WL.com Published Strategies and Sample Strategies. I also filtered by name (e.g. Trend) and can't find it. Is it on Github? Thanks.
This was just a demo that I created after modifying the code a bit from the blocks.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Drawing; using System.Collections.Generic; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { public MyStrategy() : base() { } public override void Initialize(BarHistory bars) { //WAS: //pt = new PeakTroughCalculator(bars, 40.00, PeakTroughReversalType.Percent); //create the indicator, and add it to the pt calculator and plot it _cci = CCI.Series(bars, 20); pt = new PeakTroughCalculator(_cci, 40.00, PeakTroughReversalType.Percent); PlotIndicatorLine(_cci); ChartDisplaySettings cds = new ChartDisplaySettings(); cds.LogScale = false; SetChartDrawingOptions(cds); StartIndex = 20; } public override void Execute(BarHistory bars, int idx) { int index = idx; Position foundPosition0 = FindOpenPosition(0); bool condition0; if (foundPosition0 == null) { condition0 = false; { tlFlag = false; TrendLine tl = pt.GetUpperTrendLine(index, 3, false); // 3 is the number of consecutive peaks required if (tl != null && tl.IsFalling) { double val = tl.ExtendTo(index, false); if (tl.Deviation <= 2.00) { if (_cci[index] > val) tlFlag = _cci[index - 1] < tl.ExtendTo(index - 1, false); if (tlFlag || index == bars.Count - 1) { int toIdx = index + Math.Min(bars.Count - 1 - index, 5); DrawLine(tl.Index1, tl.Value1, toIdx, tl.ExtendTo(toIdx, false), WLColor.Green, 2, LineStyle.Solid, "CCI", false, false); } } } if (tlFlag) { condition0 = true; } } if (condition0) { _transaction = PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0, 0, "Buy At Market (1)"); } } else { ClosePosition(foundPosition0, OrderType.Market, 0, "Sell At Market (1)"); } } private PeakTroughCalculator pt; private bool tlFlag; private Transaction _transaction; //Add the indicator reference CCI _cci; } }
It is under the Community folder.
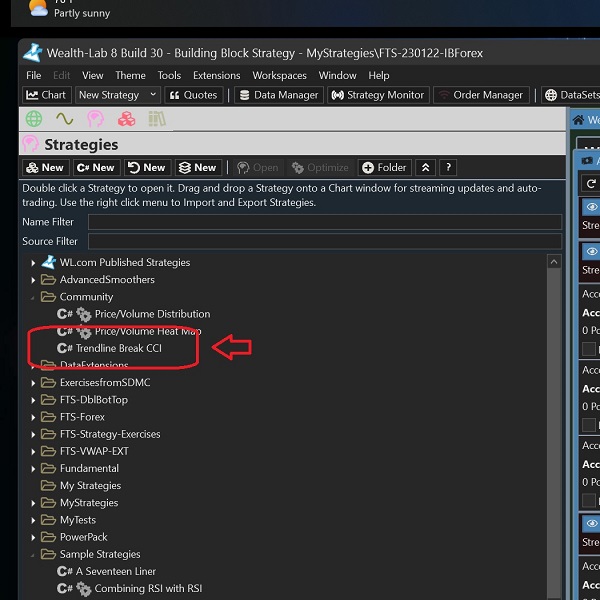
Trendline Break RSI code below with sample image. I added a filter to only enter when current close is larger than close 2 bars ago. Works pretty good. Alls I have to do is replace CCI for RSI in all the instances in Cone's code.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Drawing; using System.Collections.Generic; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { public MyStrategy() : base() { } public override void Initialize(BarHistory bars) { //WAS: //pt = new PeakTroughCalculator(bars, 40.00, PeakTroughReversalType.Percent); //create the indicator, and add it to the pt calculator and plot it _rsi = new RSI(bars.Close,20); pt = new PeakTroughCalculator(_rsi, 40.00, PeakTroughReversalType.Percent); PlotIndicatorLine(_rsi); ChartDisplaySettings cds = new ChartDisplaySettings(); cds.LogScale = false; SetChartDrawingOptions(cds); StartIndex = 0; } public override void Execute(BarHistory bars, int idx) { int index = idx; Position foundPosition0 = FindOpenPosition(0); bool condition0; if (foundPosition0 == null) { condition0 = false; { tlFlag = false; TrendLine tl = pt.GetUpperTrendLine(index, 3, false); // 3 is the number of consecutive peaks required if (tl != null && tl.IsFalling) { double val = tl.ExtendTo(index, false); if (tl.Deviation <= 2.00) { // if (bars.Close[index] > val) // tlFlag = bars.Close[index - 1] < tl.ExtendTo(index - 1, false); // if (tlFlag || index == bars.Count - 1) // { // int toIdx = index + Math.Min(bars.Count - 1 - index, 5); // DrawLine(tl.Index1, tl.Value1, toIdx, tl.ExtendTo(toIdx, false), WLColor.Green, 2, LineStyle.Solid, "Price", false, false); // } if (_rsi[index] > val) tlFlag = _rsi[index - 1] < tl.ExtendTo(index - 1, false); if (tlFlag || index == bars.Count - 1) { int toIdx = index + Math.Min(bars.Count - 1 - index, 5); DrawLine(tl.Index1, tl.Value1, toIdx, tl.ExtendTo(toIdx, false), WLColor.Green, 2, LineStyle.Solid, "RSI", false, false); } } } if (tlFlag && _rsi[index] > _rsi[index - 2]) { condition0 = true; } } if (condition0) { _transaction = PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0, 0, "Buy At Market (1)"); } } else { condition0 = false; { condition0 = true; } if (condition0) //{ //ClosePosition(foundPosition0, OrderType.Market, 0, "Sell At Market (5)"); //} Backtester.CancelationCode = 123; if (idx - foundPosition0.EntryBar + 1 >= 20) { ClosePosition(foundPosition0, OrderType.Market, 0, "Sell after 20 bars"); } } } private PeakTroughCalculator pt; private bool tlFlag; private Transaction _transaction; //Add the indicator reference RSI _rsi; } }
Impressive!
Thanks, I find the WL8 script very logical and easy to understand.
Your Response
Post
Edit Post
Login is required