I found an old thread where SymbolData indicator wasn't able to draw symbol as candlechart. That was fixed (in build 27 if I remember correctly), but it seems problem has reoccurred.
Problem1: Trying to draw two symbols on same chart with candles. SymbolInd or SymbolData can not draw candles. But I was able to plot them as blocks, so candles should be possible to plot also.
Problem2: I have VIX on actual chart, and I set VIX on lower pane with both SymbolInd and SymbolData, they align and are plotted in similar way. (Pic below)
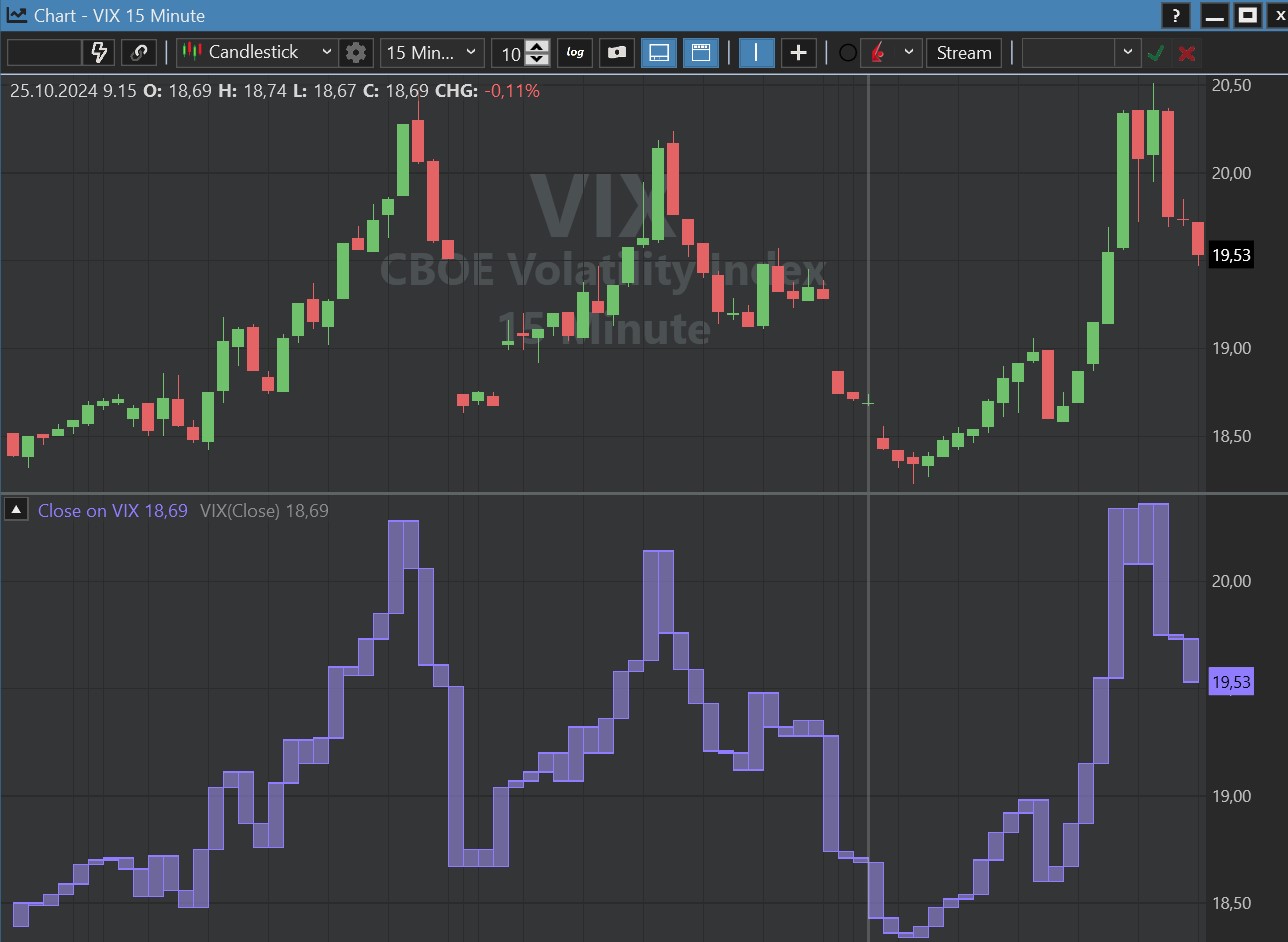
But when VIX at the actual chart is changed to something else, for example SPY, it seems VIX drawn with SymbolData jumps 4 bars into the future, while SymbolInd drawn stays the same. (pic below) Both indicators have the same settings.
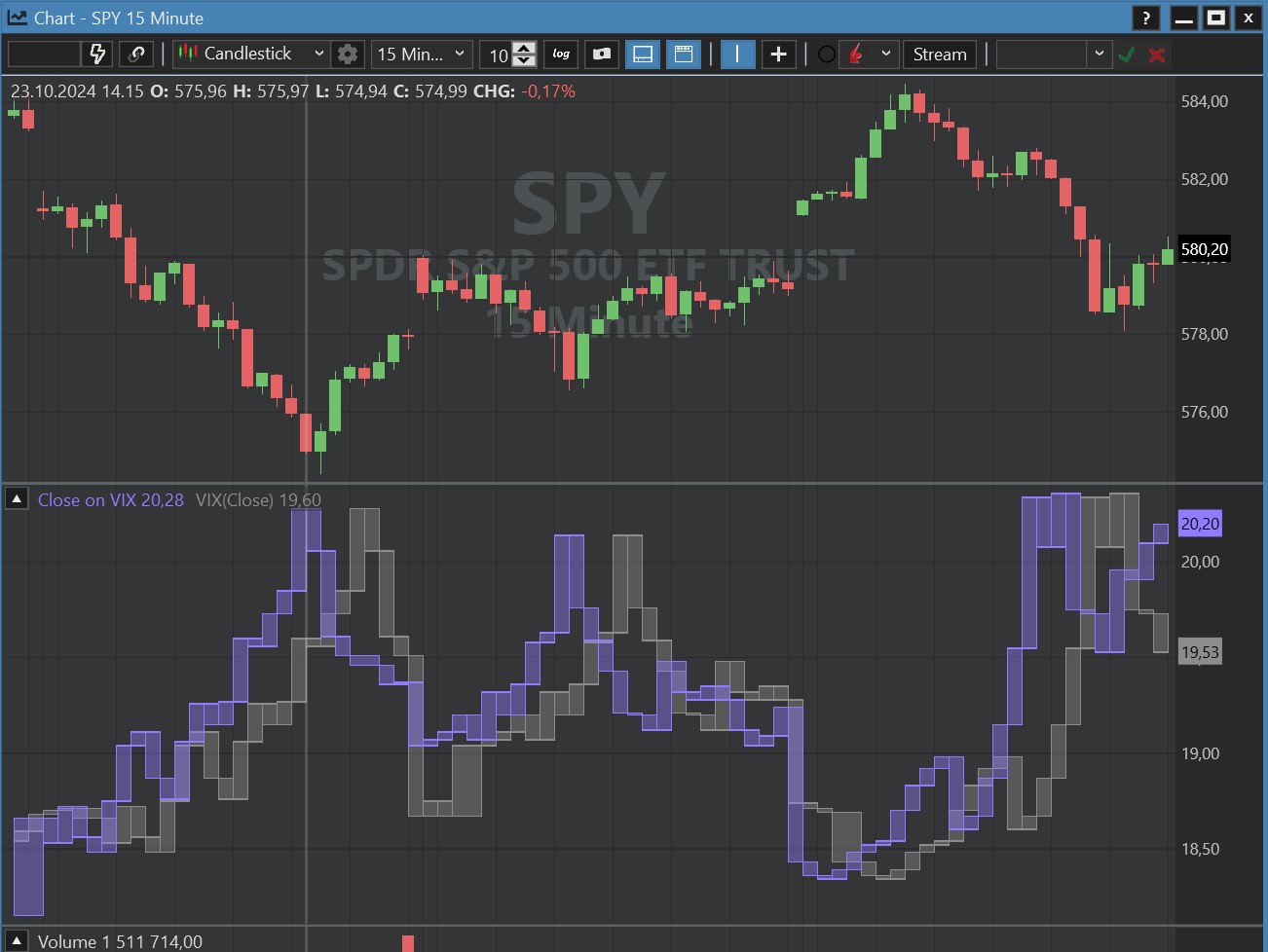
Edit: Problem3: Blocks drawn with indicators are misaligned with chart data. Using vertical chart cursor it is easy to notice that cursor is in the middle of chart bar but before/after blocks
Problem1: Trying to draw two symbols on same chart with candles. SymbolInd or SymbolData can not draw candles. But I was able to plot them as blocks, so candles should be possible to plot also.
Problem2: I have VIX on actual chart, and I set VIX on lower pane with both SymbolInd and SymbolData, they align and are plotted in similar way. (Pic below)
But when VIX at the actual chart is changed to something else, for example SPY, it seems VIX drawn with SymbolData jumps 4 bars into the future, while SymbolInd drawn stays the same. (pic below) Both indicators have the same settings.
Edit: Problem3: Blocks drawn with indicators are misaligned with chart data. Using vertical chart cursor it is easy to notice that cursor is in the middle of chart bar but before/after blocks
Rename
QUOTE:SymbolInd and SymbolData are indicators, which return a TimeSeries. You can't "candelize" (a word I just invented) a TimeSeries - you need a BarHistory.
Problem1: Trying to draw two symbols on same chart with candles.
Do it like this -
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; namespace WealthScript42 { public class MyStrategy : UserStrategyBase { public override void Initialize(BarHistory bars) { BarHistory bh = GetHistory(bars, "VIX", "Volatility Indices"); // CBOE Provider // different pane PlotBarHistory(bh, "VPane", null, false); // in the same Price pane // PlotBarHistory(bh, "Price", null, true); } public override void Execute(BarHistory bars, int idx) { } } }
Re: other problems
Show your code.
Yes, line chart can't be changed to candles without more info even though blocks can be made. But from user point of view, it kind of looks like those indicators
should be able to "candelize". Are those selections just empty templates without functionality?

SynmbolInd and SymbolData draws only straight line at daily chart. Lower timeframes work only. This might be a bug also.
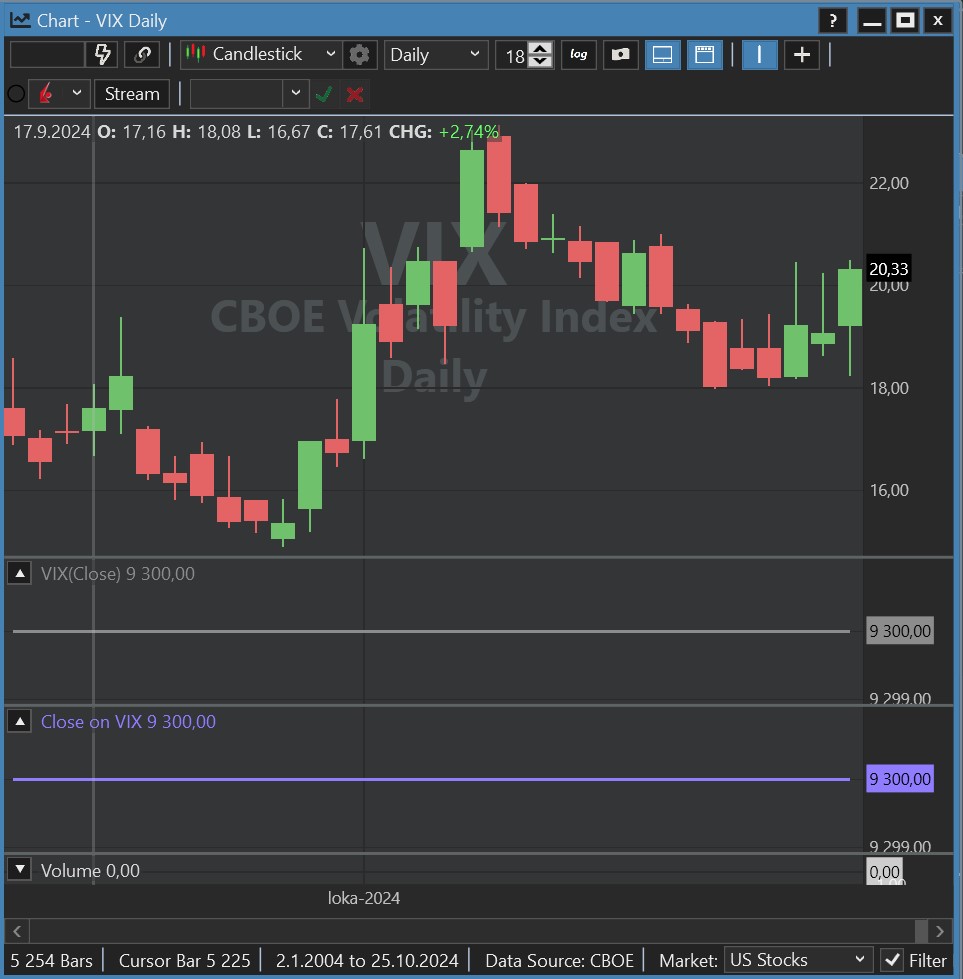
Difference between indicators can be reproduced just by setting both on chart without strategy. But here is code from some random test strategy with both indicators.
And with picture also: (It seems that other indicator is few bars in future, but also when looking at the lowest point of line between peaks, those indicators draw completeley different lines.)

should be able to "candelize". Are those selections just empty templates without functionality?
SynmbolInd and SymbolData draws only straight line at daily chart. Lower timeframes work only. This might be a bug also.
Difference between indicators can be reproduced just by setting both on chart without strategy. But here is code from some random test strategy with both indicators.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; namespace WealthScript2 { public class MyStrategy : UserStrategyBase { public MyStrategy() : base() { StartIndex = 20; } public override void Initialize(BarHistory bars) { indicator1 = new SymbolData(bars,"VIX",PriceComponent.Close); PlotIndicator(indicator1,new WLColor(0,0,0)); _startIndexList.Add(indicator1); indicator2 = new SMA(bars.Close,20); PlotIndicator(indicator2,new WLColor(0,0,255)); _startIndexList.Add(indicator2); indicator12 = new SymbolInd(bars,new TimeSeriesIndicatorWrapper(bars.Close),"VIX"); PlotIndicator(indicator12,new WLColor(255,0,0)); _startIndexList.Add(indicator12); indicator22 = new SMA(bars.Close,20); _startIndexList.Add(indicator22); foreach(IndicatorBase ib in _startIndexList) if (ib.FirstValidIndex > StartIndex) StartIndex = ib.FirstValidIndex; } public override void Execute(BarHistory bars, int idx) { int index = idx; Position foundPosition0 = FindOpenPosition(0); bool condition0; if (foundPosition0 == null) { condition0 = false; { if (index - 0 >= 0 && indicator1[index] < indicator2[index - 0]) { if (index - 0 >= 0 && indicator12[index] < indicator22[index - 0]) { condition0 = true; } } } if (condition0) { Backtester.CancelationCode = 1; _transaction = PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0, 0, "Buy At Market (1)"); } } else { { Backtester.CancelationCode = 1; if (idx - foundPosition0.EntryBar + 1 >= 5) { ClosePosition(foundPosition0, OrderType.Market, 0,"Sell after 5 bars"); } } } } public override void NewWFOInterval(BarHistory bars) { indicator1 = new SymbolData(bars,"VIX",PriceComponent.Close); indicator2 = new SMA(bars.Close,20); indicator12 = new SymbolInd(bars,new TimeSeriesIndicatorWrapper(bars.Close),"VIX"); indicator22 = new SMA(bars.Close,20); } private IndicatorBase indicator1; private IndicatorBase indicator2; private IndicatorBase indicator12; private IndicatorBase indicator22; private Transaction _transaction; private List<IndicatorBase> _startIndexList = new List<IndicatorBase>(); } }
And with picture also: (It seems that other indicator is few bars in future, but also when looking at the lowest point of line between peaks, those indicators draw completeley different lines.)
>> Are those selections just empty templates without functionality?<<
The BarChart Plot Style supports very specific indicators like VChart that include additional OHLC data.
The BarChart Plot Style supports very specific indicators like VChart that include additional OHLC data.
QUOTE:No, they are enumerations for PlotStyles available for strategy code. We could probably filter the choices for indicators, but it's not too hard to ignore the choices that clearly don't apply - but you can still select them without error if you want.
Are those selections just empty templates without functionality?
Re: the delay
You're getting VIX intraday from IB, which is CME, CST timestamps. The SymbolData indicator - the one with the VIX(Close) label - is correctly synchronized to the charted symbol's EST time.
We'll have to see if there a way for SymbolInd to recognize different markets. Until then, you know to use SymbolData for VIX instead.
QUOTE:
The SymbolData indicator - the one with the VIX(Close) label - is correctly synchronized to the charted symbol's EST time.
I am a bit confused now. It looks like VIX (SymbolInd) is displaying the correct value at the correct time when drawn on chart. Highest value (candle close) of VIX should be 14:15 according to timestamp on picture (see pic below). This time and value have been checked from other sources too.
But when I set buying to happen when close of VIX is greater than 20,26, using symbol VIX" (highest point being 20.28), buying is executed using SymbolData value, which peaks way too late.
Unable to add picture by editing message (would be useful feature).
Here is correct picture.
Here is correct picture.
Now I understand, and know how to get expected results. It is so that definately SymbolInd is correct and SymbolData not.
Below is how I get buy to happen as it should using SymbolInd
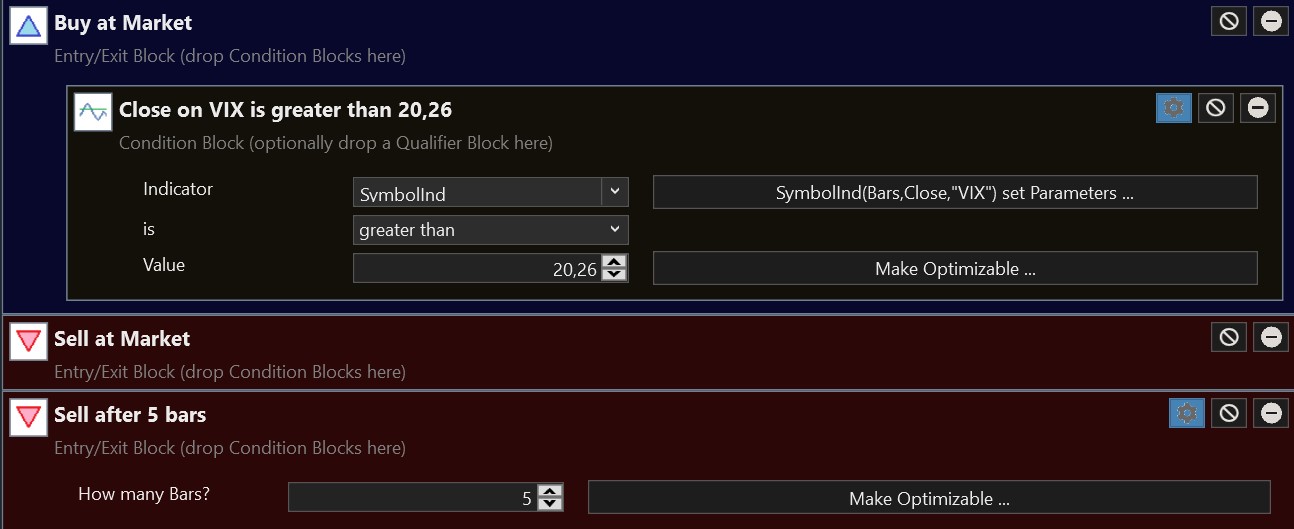
And here is the way that causes buy to happen too late. I believe "using symbol" building block uses SymbolData and causes buying to happen too late. (value here is 20 instead of 20.26, but no effect on the outcome)
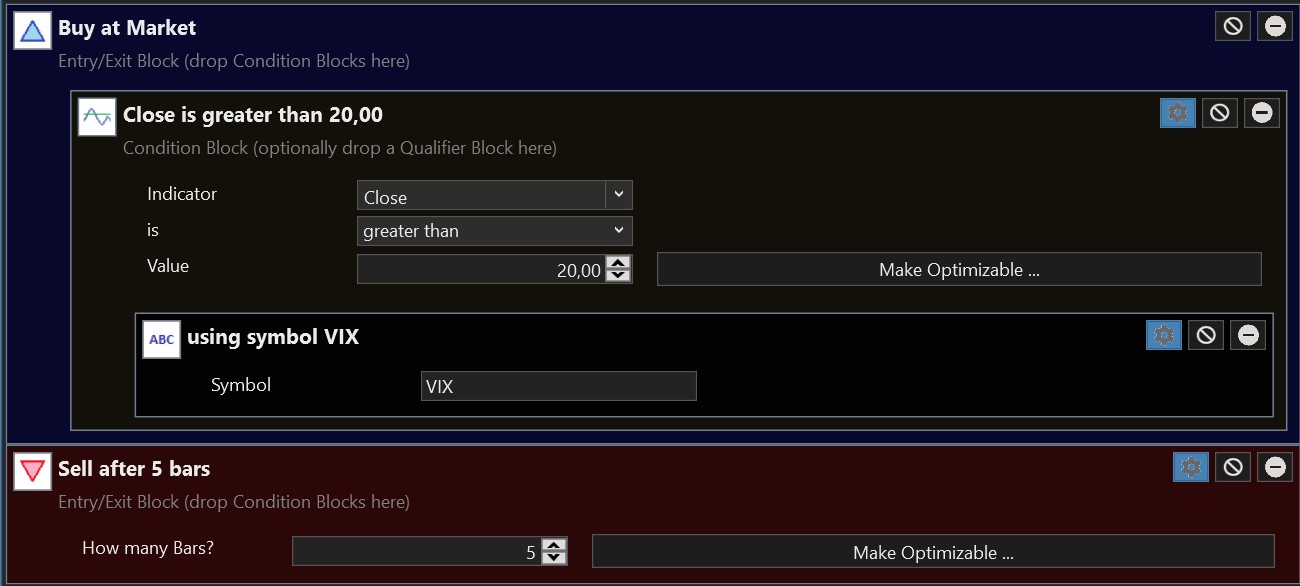
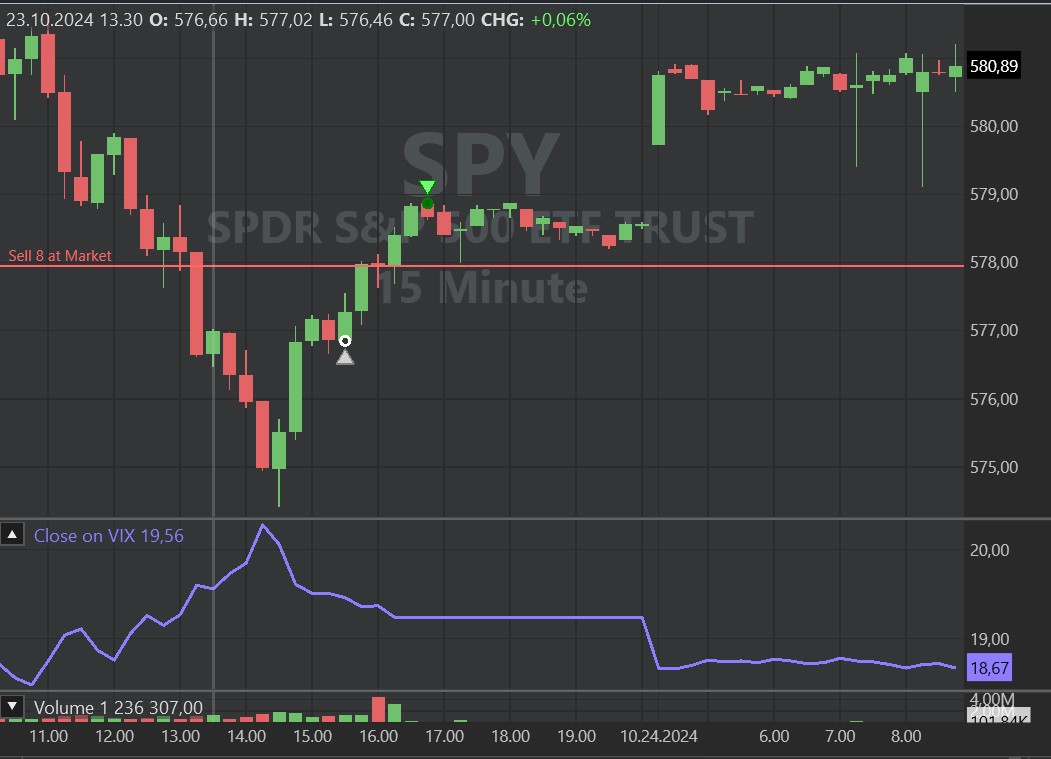
Below is how I get buy to happen as it should using SymbolInd
And here is the way that causes buy to happen too late. I believe "using symbol" building block uses SymbolData and causes buying to happen too late. (value here is 20 instead of 20.26, but no effect on the outcome)
If you put your IB-sourced VIX on a VIX chart, then everything is in CST. There's no sync problem there because it's not synchronizing to a different market.
The problem is putting a [intraday] VIX in CST on a chart like SPY in EST. SymbolInd is WRONG because it's not in the same time zone. It's peeking by one hour in a backtest or when reviewing it in a chart like the one right above this post ^^^
SymbolData correctly aligns the data between the two zones.
The problem is putting a [intraday] VIX in CST on a chart like SPY in EST. SymbolInd is WRONG because it's not in the same time zone. It's peeking by one hour in a backtest or when reviewing it in a chart like the one right above this post ^^^
SymbolData correctly aligns the data between the two zones.
You must have added a record for that in Markets & Symbols, did you not?
Hang on.. let me step back and look at it more carefully. I might be experiencing a DST issue right now since Europe just "fell back" one hour.
It appears that due to the DST transition in Europe (occurs next week in the U.S.), the IB Provider's data before today (i.e., last week) is incorrectly advanced by 1 hour. In this current scenario, when looking at IB data before today, an IB timestamp at 4pm actually represents the market at 3pm EST. This [probably] only affects those of us in Europe trading U.S. markets.
IB's data for "today" is timestamped correctly.
So this is all another complicating factor to something that was already complicated. We'll work on it.
IB's data for "today" is timestamped correctly.
So this is all another complicating factor to something that was already complicated. We'll work on it.
Just for clarification purposes:
SymbolInd here is wrong (lower), and SymbolData (upper) is correct. But, because DST transition is causing 1 hour timejump in SymbolData, it causes that SymbolInd is atm correct even though it is in principle wrong.
Complicated indeed.

But when using ESz24 instead of SPY, it seems both are "correct" but having the same DST transition error?
SymbolInd here is wrong (lower), and SymbolData (upper) is correct. But, because DST transition is causing 1 hour timejump in SymbolData, it causes that SymbolInd is atm correct even though it is in principle wrong.
Complicated indeed.
But when using ESz24 instead of SPY, it seems both are "correct" but having the same DST transition error?
The only reason SymbolInd is lining up today on a CME market is because DST ended in Europe but hasn't ended in the US.
It will change again next week.
It will change again next week.
In summary, there are a couple issues here.
1. SymbolInd doesn't necessarily have a way to synchronize to an arbitrary TimeSeries because TimeSeries don't need to be associated with a Market. Instead use SymbolData in these edge cases.
2. IB Provider bug
The provider calculates and applies a single Market Time Zone correction to bars retrieved in data requests from IB. The correction will be correct for "today". But if the data covers a DST time zone change, the data previous to the change won't be timestamped correctly.
To correct this, we have to calculate the correction for every bar timestamp individually. We'll get it done, but it probably won't be ready this week. :(
1. SymbolInd doesn't necessarily have a way to synchronize to an arbitrary TimeSeries because TimeSeries don't need to be associated with a Market. Instead use SymbolData in these edge cases.
2. IB Provider bug
The provider calculates and applies a single Market Time Zone correction to bars retrieved in data requests from IB. The correction will be correct for "today". But if the data covers a DST time zone change, the data previous to the change won't be timestamped correctly.
To correct this, we have to calculate the correction for every bar timestamp individually. We'll get it done, but it probably won't be ready this week. :(
IB Provider is ready with the DST fix.
1. Do what the Important New Guidance! says in the Change Log for Build 57.
2. To fix the data, you'll need to either 1) refresh each chart that was affected, or, 2) use the Data Tool's Data Truncation feature in the Data Manager (Delete Data after 2024-10-25)
1. Do what the Important New Guidance! says in the Change Log for Build 57.
2. To fix the data, you'll need to either 1) refresh each chart that was affected, or, 2) use the Data Tool's Data Truncation feature in the Data Manager (Delete Data after 2024-10-25)
Cool! It seems all data is in sync now. (And btw impressed for a such fast fix.)
Only thing worrying me for now regarding this matter, is that symbol "VIX" only draws straight line on daily chart, when using SymbolData or SymbolInd. I tested also with many other symbols, and everything else works like a charm: AAPL, ESZ24, SPY, even VXN, and VVIX work, but not VIX
Only thing worrying me for now regarding this matter, is that symbol "VIX" only draws straight line on daily chart, when using SymbolData or SymbolInd. I tested also with many other symbols, and everything else works like a charm: AAPL, ESZ24, SPY, even VXN, and VVIX work, but not VIX
Open a new chart of VIX only and look at the provider at the bottom.
Which Provider is the source?
For me, if VIX is source by CBOE or IB, it works fine - (whoops, just noticed RSI(VIX) for the SymbolInd version below)
Which Provider is the source?
For me, if VIX is source by CBOE or IB, it works fine - (whoops, just noticed RSI(VIX) for the SymbolInd version below)
On daily timeframe (and it seems all TFs above), if I just type "VIX" and press enter, I get a scrambled chart of Bitcoin volatility from Yahoo. Of course when I type VIX and select IB or CBOE as data providers, I get the correct chart.
On lower timeframes I get VIX automaticly from IB. So VIX loading bitcoin chart seems it might be the problem
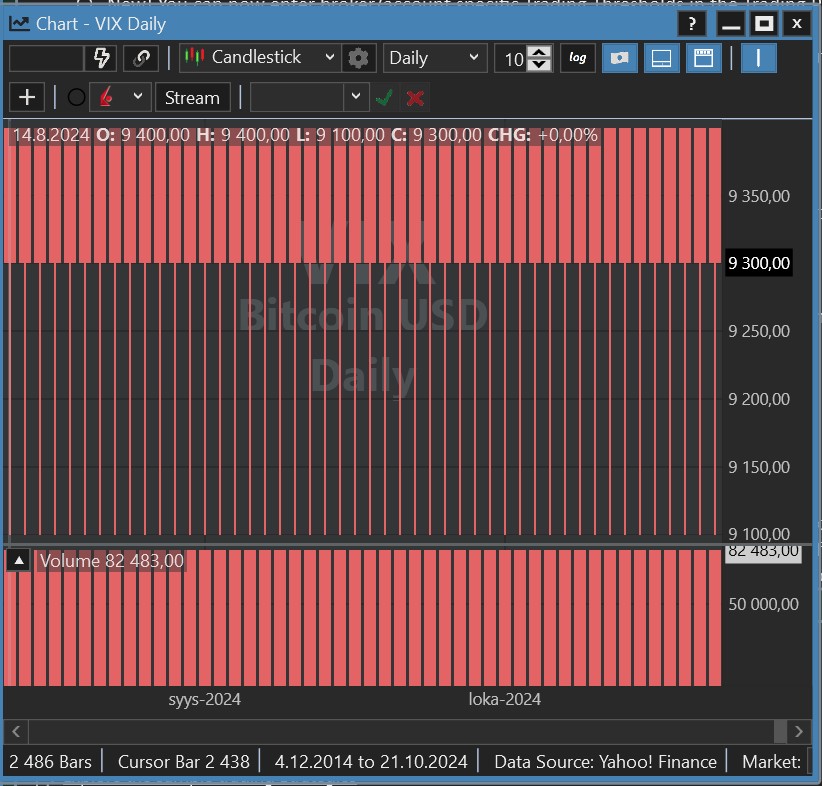
On lower timeframes I get VIX automaticly from IB. So VIX loading bitcoin chart seems it might be the problem
Turn off Yahoo! or move it below IB (or CBOE) in the Data Manager > Historical Provider list.
Works 100% now. Thanks!
Your Response
Post
Edit Post
Login is required