Good Day,
Could you please assist me on how to create a strategy (RSI Cross 20 MA) on a 15 Minute Heikn Ashi Chart (and not standard chart)?
Thank you
Wes
Could you please assist me on how to create a strategy (RSI Cross 20 MA) on a 15 Minute Heikn Ashi Chart (and not standard chart)?
Thank you
Wes
Rename
Hi Wes,
Trades are created on the real bars, not virtual ones like Heikin Ashi. But I think it's also possible to backtest the idea:
Trades are created on the real bars, not virtual ones like Heikin Ashi. But I think it's also possible to backtest the idea:
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Collections.Generic; namespace WealthScript2 { public class MyStrategy : UserStrategyBase { public override void Initialize(BarHistory bars) { price = bars.Close; sma = new SMA(bars.Close,20); ha = new BarHistory(bars); StartIndex = 20; // Create Heikin-Ashi series TimeSeries HO = bars.Open *0; TimeSeries HH = bars.High *0; TimeSeries HL = bars.Low *0; TimeSeries HC = bars.AveragePriceOHLC; // Build the Bars object ha.Add(bars.DateTimes[0], HO[0], HH[0], HL[0], HC[0], bars.Volume[0]); for (int bar = 1; bar < bars.Count; bar++) { double o1 = HO[bar - 1]; double c1 = HC[bar - 1]; HO[bar] = (o1 + c1) / 2; HH[bar] = Math.Max(HO[bar], bars.High[bar]); HL[bar] = Math.Min(HO[bar], bars.Low[bar]); ha.Add(bars.DateTimes[bar], HO[bar], HH[bar], HL[bar], HC[bar], bars.Volume[bar]); } PlotBarHistoryStyle(ha, "HAPane", "Candlestick", WLColor.Navy); PlotIndicator(sma, new WLColor(0, 0,0)); } public override void Execute(BarHistory bars, int idx) { if (!HasOpenPosition(ha, PositionType.Long)) { if (price.CrossesOver(sma, idx)) _transaction = PlaceTrade(ha, TransactionType.Buy, OrderType.Market, 0, 0, "Buy At Market (1)"); } else { if (price.CrossesUnder(sma, idx)) ClosePosition(LastPosition, OrderType.Market, 0, "Sell At Market (1)"); } } private TimeSeries price; private IndicatorBase sma; private Transaction _transaction; BarHistory ha; } }
Thank You
This can be simplified using our framework's HeikinAshi helper class,
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Collections.Generic; namespace WealthScript2 { public class MyStrategy : UserStrategyBase { public override void Initialize(BarHistory bars) { price = bars.Close; sma = new SMA(bars.Close, 20); ha = new BarHistory(bars); StartIndex = 20; // Create Heikin-Ashi series ha = HeikinAshi.Convert(bars); PlotBarHistoryStyle(ha, "HAPane", "Candlestick", WLColor.Navy); PlotIndicator(sma, new WLColor(0, 0, 0)); } public override void Execute(BarHistory bars, int idx) { if (!HasOpenPosition(ha, PositionType.Long)) { if (price.CrossesOver(sma, idx)) _transaction = PlaceTrade(ha, TransactionType.Buy, OrderType.Market, 0, 0, "Buy At Market (1)"); } else { if (price.CrossesUnder(sma, idx)) ClosePosition(LastPosition, OrderType.Market, 0, "Sell At Market (1)"); } } private TimeSeries price; private IndicatorBase sma; private Transaction _transaction; private BarHistory ha; } }
It's not really valid, is it? You can create trades on the HA series, but these may be trades at prices that don't actually exist on the trade bar -
Thank You.
On the chart & on the Backtesting it gives you the right numbers on the Order Manager it gives you funny numbers
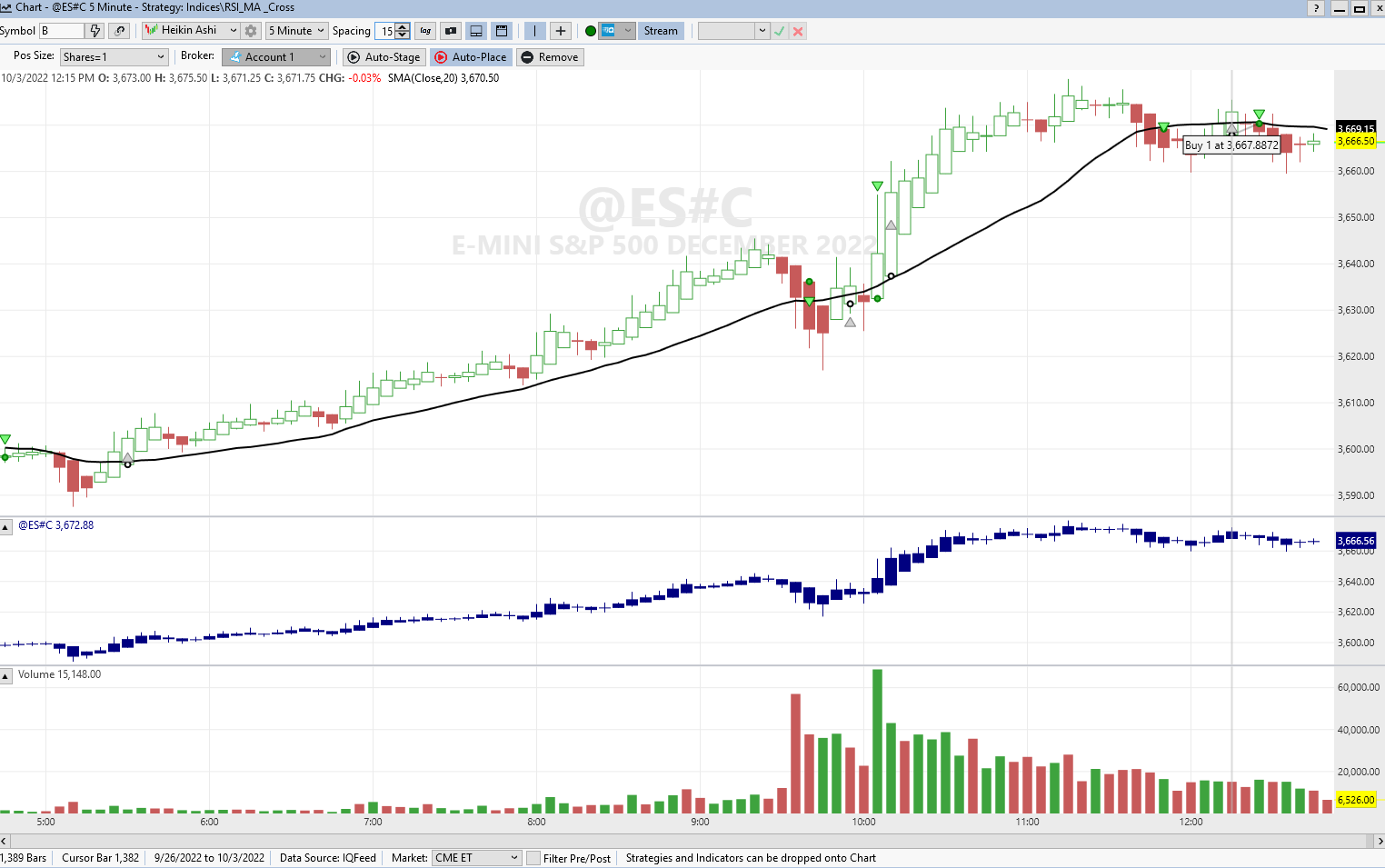
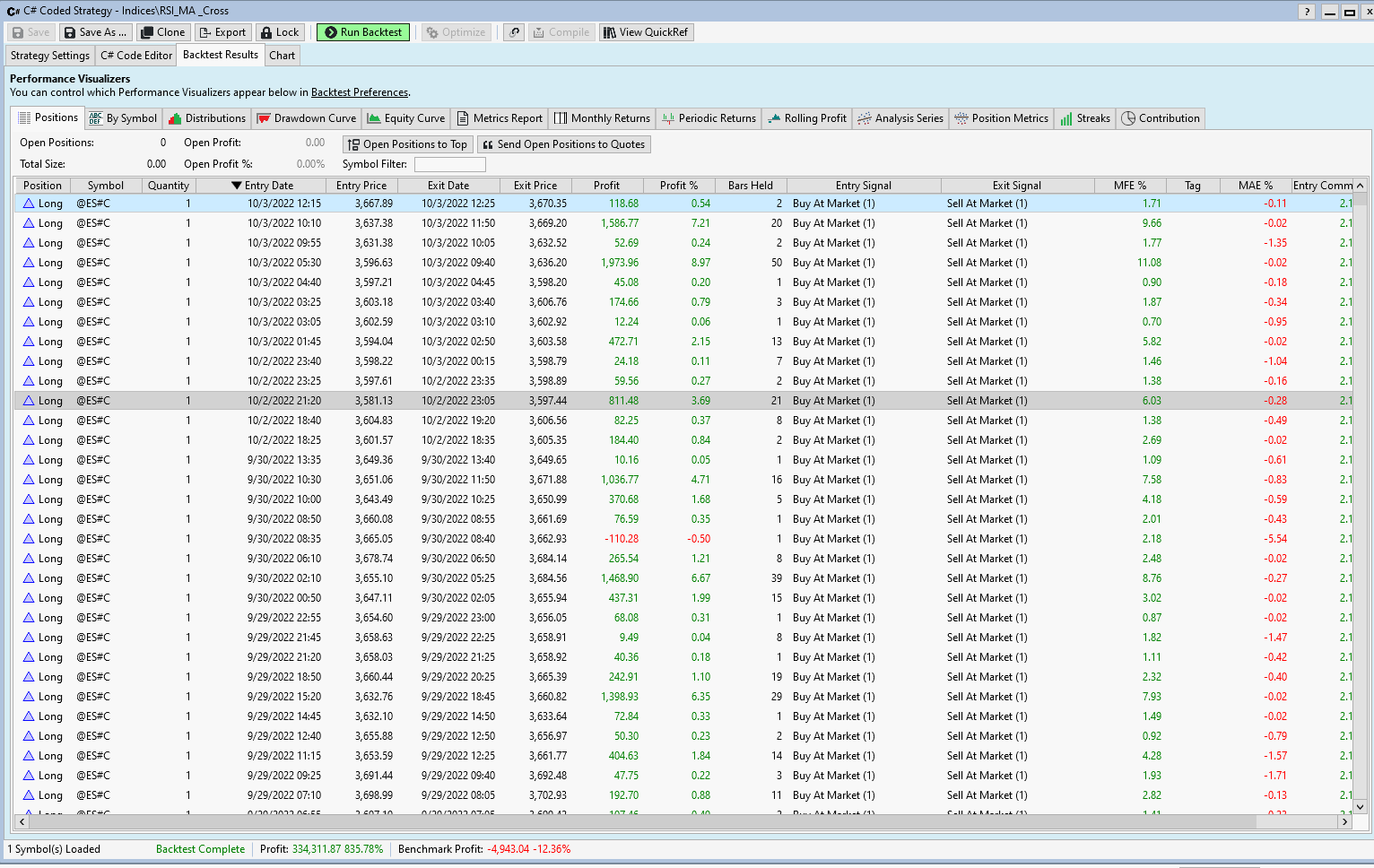
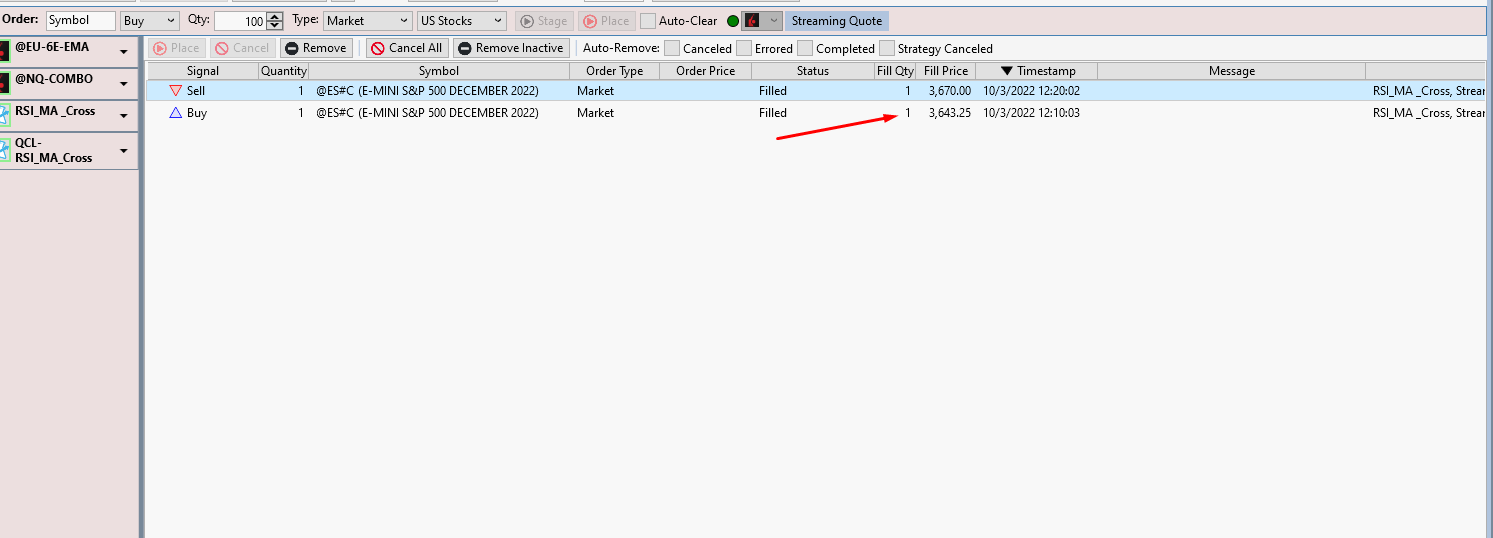
Re: Cone, yes I just converted Eugene's code but didn't examine it closely. Of course, you cannot PlaceTrade on the Heikin-Ashi BarHistory, here is a corrected version. It applies the SMA to the Heikin-Ashi and signals based off it, but places the trades on the underlying BarHistory.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Collections.Generic; namespace WealthScript2 { public class MyStrategy : UserStrategyBase { public override void Initialize(BarHistory bars) { StartIndex = 20; // Create Heikin-Ashi series ha = HeikinAshi.Convert(bars); sma = new SMA(ha.Close, 20); PlotBarHistoryStyle(ha, "HA", "Candlestick", WLColor.Aqua); PlotIndicator(sma, WLColor.Fuchsia, PlotStyle.Line, false, "HA"); } public override void Execute(BarHistory bars, int idx) { if (!HasOpenPosition(ha, PositionType.Long)) { if (ha.Close.CrossesOver(sma, idx)) PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0, 0, "Buy At Market (1)"); } else { if (ha.Close.CrossesUnder(sma, idx)) ClosePosition(LastPosition, OrderType.Market, 0, "Sell At Market (1)"); } } private IndicatorBase sma; private BarHistory ha; } }
Your Response
Post
Edit Post
Login is required