I am developing an idea for a system with multiple positions, and I wanted to sell only 100 shares of the 300 that I buy, how can I do that?
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; using Steema.TeeChart.Functions; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { public override void Initialize(BarHistory bars) { } public override void Execute(BarHistory bars, int idx) { Backtester.CancelationCode = 1; tBuy = PlaceTrade(bars, TransactionType.Buy, OrderType.Market); tBuy.Quantity = 300; foreach (Position foundPosition0 in OpenPositions) { if(foundPosition0.ProfitPctAsOf(idx) >= 10) { Backtester.CancelationCode = 1; ClosePosition(foundPosition0, OrderType.Market); } } } //declare private variables below Transaction tBuy, tSell; } }
Rename
Instead of using ClosePosition, use PlaceTrade and assign 100 to the Quantity of the resulting Transaction.
But if I do it this way, it will close the last position that was opened, not the position that has at least 10% profit:
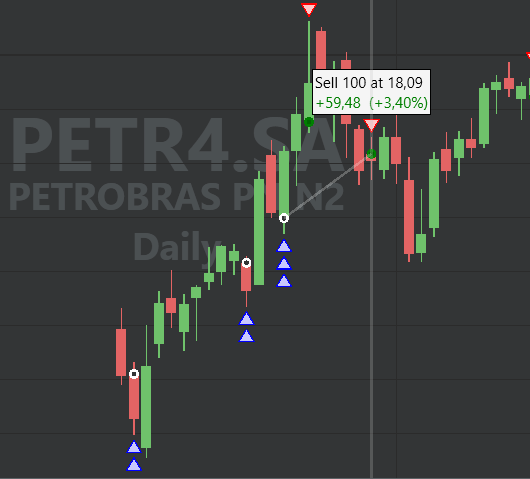
CODE:
if (sell == 1) { foreach (Position foundPosition0 in OpenPositions) { if(foundPosition0.ProfitPctAsOf(idx) >= 10) { Backtester.CancelationCode = 1; tSell = PlaceTrade(bars, TransactionType.Sell, OrderType.Market); tSell.Quantity = 100; break; } } }
Then you'll need to issue two buys, one for 200 shares and one for 100 shares. Exit the one with 100 shares that has 10% profit.
And if you don't specifically identify the position you want to exit, then WealthLab uses this option - try FIFO here, for example.
Even with this change in configuration and buying separately, he still sells the shares in the wrong position.
There would have to be a way to sell shares in a specific quantity from a specific position, right?
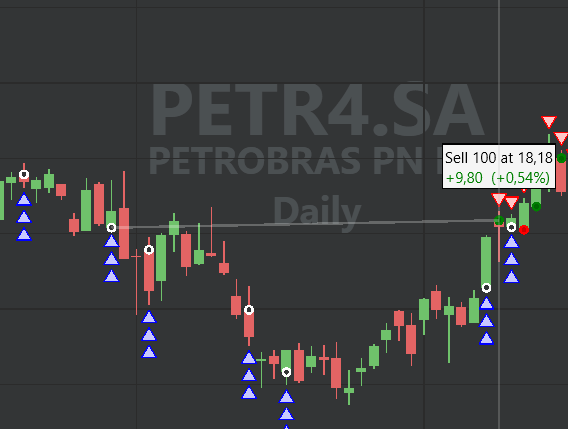
There would have to be a way to sell shares in a specific quantity from a specific position, right?
If you selected FIFO, then it exited the oldest active position, right?
QUOTE:Of course. Find the reference to the position that you want to exit and pass it to ClosePosition(). The "problem" with ClosePosition() is that it doesn't return a Transaction object and exits the full position.
There would have to be a way to sell shares in a specific quantity from a specific position, right?
Try this variation, it issues 2 buys, like I suggested above, and sells the one of 100 shares when it reaches 10%. Since you didn't specify any exit criteria for the remaining positions I coded it to sell them when they reach 20% profit. Adjust accordingly.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; using Steema.TeeChart.Functions; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { public override void Initialize(BarHistory bars) { } public override void Execute(BarHistory bars, int idx) { Backtester.CancelationCode = 1; tBuy = PlaceTrade(bars, TransactionType.Buy, OrderType.Market); tBuy.Quantity = 100; tBuy.SignalName = "1"; tBuy = PlaceTrade(bars, TransactionType.Buy, OrderType.Market); tBuy.Quantity = 200; tBuy.SignalName = "2"; foreach (Position foundPosition0 in OpenPositions) { if (foundPosition0.ProfitPctAsOf(idx) >= 10 && foundPosition0.EntrySignalName == "1") { Backtester.CancelationCode = 1; ClosePosition(foundPosition0, OrderType.Market); } if (foundPosition0.ProfitPctAsOf(idx) >= 20 && foundPosition0.EntrySignalName == "2") { Backtester.CancelationCode = 1; ClosePosition(foundPosition0, OrderType.Market); } } } //declare private variables below Transaction tBuy, tSell; } }
Your Response
Post
Edit Post
Login is required