Hello,
The code below simply tries to find first bar and market open of the day for use with intraday scales in SM. However, it only works correctly if activated after market open (9:30). Before open activations return wrong numbers. I have tried different scales (1 min & 5 min) and different data range (100 bars & 500 bars) but all return the same wrong results.
Is there anything missing here?
Thanks.
The code below simply tries to find first bar and market open of the day for use with intraday scales in SM. However, it only works correctly if activated after market open (9:30). Before open activations return wrong numbers. I have tried different scales (1 min & 5 min) and different data range (100 bars & 500 bars) but all return the same wrong results.
Is there anything missing here?
Thanks.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Drawing; using WealthLab.ChartWPF; using System.Collections.Generic; using System.IO; namespace WealthScript12 { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (bars.IsLastBarOfDay(idx)) lastBarYesterday = idx; if (bars.IsFirstBarOfDay(idx)) startBarToday = idx; //if (bars.IntradayBarNumber(idx) == 0) // startBarToday = idx; yesterdayClose = bars.Close[lastBarYesterday]; todayOpen = bars.Open[startBarToday]; Transaction t = PlaceTrade(bars, TransactionType.Sell, OrderType.Limit, todayOpen, String.Format("{0:f2} -", todayOpen)+bars.DateTimes[startBarToday].ToString()); } //declare private variables below int startBarToday; int lastBarYesterday; double todayOpen; double yesterdayClose; } }
Rename
As for my experience, this functions deal with market settings - open and close time. Sometimes it does not correlate to what you want - premarket, postmarket or something. I prefer to use manual checks, like if this bar date != previous bar date - this bar is the first bar of the date. The same for last bar, but you should compare current bar date and next bar date.
Mm, it's about Strategy Monitor, so comparing to the next bar will not work). But first bar of the day will still work.
You can use these functions on any bar in your strategy to return to determine if it's the last or first bar of that day - the day for the index.
If you need to know if it's the first bar of "today" as in DateTime.Now.Date, then you can condition it further, for example -
1. Time zones. If your computer is in another time zone far away from the market's time zone, you'll need to make another adjustment for the dates to match properly.
2. This will only work for "today" - you'll need to add more logic if you need your backtest to work for previous days.
3. You'll need to make another adjustment if you really need lastBarYesterday to be true for "today's last bar".
If you need to know if it's the first bar of "today" as in DateTime.Now.Date, then you can condition it further, for example -
CODE:Some things to keep in mind:
if (idx != bars.Count - 1) if (bars.IsLastBarOfDay(idx) && bars.DateTimes[idx + 1].Date == DateTime.Now.Date) lastBarYesterday = idx; if (bars.IsFirstBarOfDay(idx) && bars.DateTimes[idx].Date == DateTime.Now.Date) startBarToday = idx;
1. Time zones. If your computer is in another time zone far away from the market's time zone, you'll need to make another adjustment for the dates to match properly.
2. This will only work for "today" - you'll need to add more logic if you need your backtest to work for previous days.
3. You'll need to make another adjustment if you really need lastBarYesterday to be true for "today's last bar".
QUOTE:
If you need to know if it's the first bar of "today" as in DateTime.Now.Date, then you can condition it further, for example -
Why additional conditions are needed in order to find the first bar of today when only 100 one-minute bar has been loaded in SM? How many first bars of the day are supposed to exist in this small set which then requires further separation by using additional conditions? Furthermore, why no additional condition is needed if the SM is activated after market open?
So here is the scenario again:
Above code in SM with 100 bars of 1-min preloaded (no pre-market trading). If activated before open, say 9:20, returns wrong numbers after the first run @9:31. However, if activated after market open, say 9:32, it works correctly.
You can simply duplicate it and see...
QUOTE:
If activated before open, say 9:20, returns wrong numbers after the first run @9:31. However, if activated after market open, say 9:32, it works correctly.
Okay, thanks. That's much clearer now. We'll take a look.
I'll assume U.S. Market, but what data method and provider are you using? Streaming, Streaming Bars, or Polling? Data Provider?
Edit:
You said in the starter post, "but all return the same wrong results."
What results are you looking for with this script? There is only a sell signal without a signal to create a long position. Unless you actually assign a Quantity to the transaction, nothing will happen.
It is US market, TD, and Streaming Data.
By "wrong results" I mean incorrect data for the first bar of the day (Market Open). Transaction type can be changed to Buy, or as you suggested
By "wrong results" I mean incorrect data for the first bar of the day (Market Open). Transaction type can be changed to Buy, or as you suggested
CODE:can be added.
t.Quantity = 10;
Something that occurred to me while setting up for the test this morning. You need to make this selection in your Strategy for it to filter for regular market hours in the S. Monitor. Could that be the problem?
I modified the script slightly as follows - it will alert as long as the first bar's date doesn't equal yesterday's last bar date.
I got these alerts for the first bar at 0931. The dates are correct for first and yesterday's (last) bar.
IB streaming updates about every 250 msec, so it's not a tick-by-tick provider and small differences in opening and closing prices can be expected. However, I cannot reconcile many of these opening prices. For example, the highlighted DIS is showing 158.17, but the actual opening price(s) were solidly below 158 for the first several seconds and the High of the entire bar did not exceed 158.06. Unfortunately, I wasn't running streaming charts to bounce this result.
CODE:
public override void Execute(BarHistory bars, int idx) { if (bars.IsLastBarOfDay(idx)) lastBarYesterday = idx; if (bars.IsFirstBarOfDay(idx)) startBarToday = idx; yesterdayClose = bars.Close[lastBarYesterday]; todayOpen = bars.Open[startBarToday]; //if this is the last bar, "today" is now "yesterday", so don't alert if (bars.DateTimes[startBarToday].Date != bars.DateTimes[lastBarYesterday].Date) { string msg = String.Format("{0:f2} - {1:G}; last: {2:G}", todayOpen, bars.DateTimes[startBarToday], bars.DateTimes[lastBarYesterday]); Transaction t = PlaceTrade(bars, TransactionType.Sell, OrderType.Limit, todayOpen, msg); t.Quantity = 1; } }
I got these alerts for the first bar at 0931. The dates are correct for first and yesterday's (last) bar.
IB streaming updates about every 250 msec, so it's not a tick-by-tick provider and small differences in opening and closing prices can be expected. However, I cannot reconcile many of these opening prices. For example, the highlighted DIS is showing 158.17, but the actual opening price(s) were solidly below 158 for the first several seconds and the High of the entire bar did not exceed 158.06. Unfortunately, I wasn't running streaming charts to bounce this result.
I ran another modification to create the startBarToday on the first run in the middle of the day. Everything checked fine there. I'll take another look at the opening tomorrow to see if I can make some sense of those opening prices.
Thanks for the updates. Here is mine:
I ran your slightly modified code this morning as before on 1-min and 5-min data with 100 bars loaded. Again, after market open activations returned correct Opens, but before open activations problem still persist. (both 1-min and 5-min results are incorrect and different from each other)
Below are the snapshots showing the details:
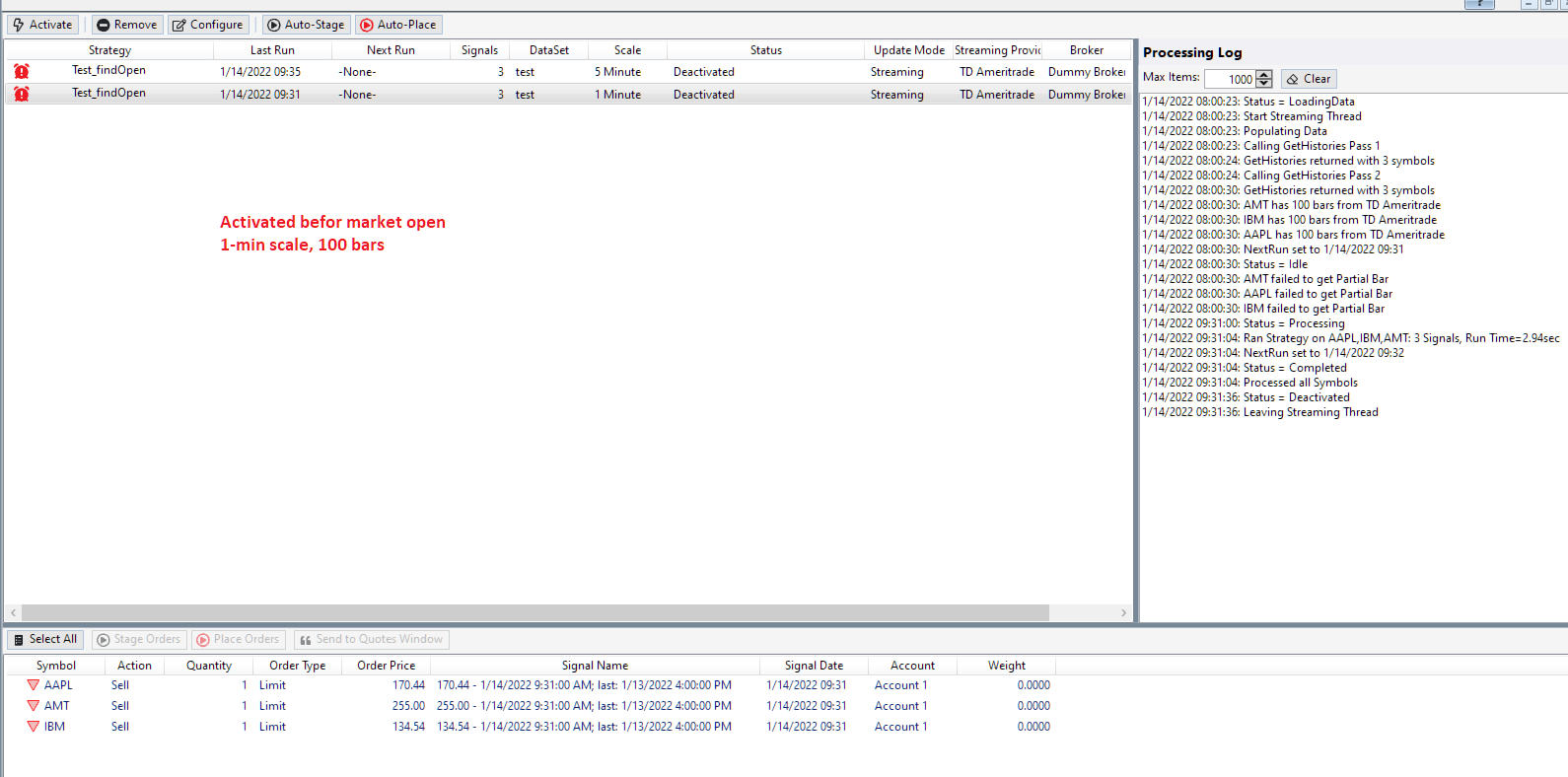
I ran your slightly modified code this morning as before on 1-min and 5-min data with 100 bars loaded. Again, after market open activations returned correct Opens, but before open activations problem still persist. (both 1-min and 5-min results are incorrect and different from each other)
Below are the snapshots showing the details:
Yes, I saw it today and backed it up with Streaming charts for comparison. I'm looking into it.
I think we've got this licked for Build 46, scheduled for release later today.
hello,
I checked this morning (using build 46) and it seems that the problem still persist for before 9:30 activation, and only one of the three symbols returned correct open.
I will check again tomorrow on a larger dataset.
I checked this morning (using build 46) and it seems that the problem still persist for before 9:30 activation, and only one of the three symbols returned correct open.
I will check again tomorrow on a larger dataset.
1. What do you mean by "before 09:30 activation"?
The first run for any strategy during U.S. market hours will be after 09:30. It will occur at 09:31 for 1-minute bars, 09:35 for 5-minute bars, etc.
2. Does the strategy have "Filter Pre/Post" checked?
Although I know it's not ideal, this setting must be saved with the Strategy to work in the S. Monitor.
3.. What data provider?
The first run for any strategy during U.S. market hours will be after 09:30. It will occur at 09:31 for 1-minute bars, 09:35 for 5-minute bars, etc.
2. Does the strategy have "Filter Pre/Post" checked?
Although I know it's not ideal, this setting must be saved with the Strategy to work in the S. Monitor.
3.. What data provider?
I thought the problem was clearly defined, discussed and shown in the previous posts and screenshots. Also, I thought you were able to duplicate it as you confirmed in your post on Jan, 14.
here are the answers again:
"before 9:30 activation" means activating the strategy in SM anytime before 9:30 (say 9:20).
data provide: TD
US market
the Filter is checked and saved with the strategy
Please let me know which part is not clear yet.
here are the answers again:
"before 9:30 activation" means activating the strategy in SM anytime before 9:30 (say 9:20).
data provide: TD
US market
the Filter is checked and saved with the strategy
Please let me know which part is not clear yet.
I'm not sure how you're doing the verifications, but it's likely that the problem you're seeing is due to using TDA Streaming. TDA (and IB) Streaming are conflated ticks, so these charts will NOT match a provider's historical charts, which are created tick-by-tick. Variations are expected in some of the OHLC values between TDA (and IB) streaming and their historical charts.
Aside:
IQFeed Streaming is tick-by-tick and this could be used to compare to historical charts.
Knowing about the conflated ticks issue, we worked tirelessly on integrating Streaming Bars for IB. It paid off. IB's Streaming Bars in the Strategy Monitor precisely match historical charts. I haven't looked to see if TDA has a similar facility, but if they do, we'll get to it.
Edit/Update: TD does have streaming bars. Took a quick look and it appears they come in consistently :04 secs after the minute mark, which is probably both good and bad. Good, because it probably indicates that we're getting the real tick-by-tick minute bar. Bad because it's a 4 second delay. Anyway, we'll get this incorporated into the provider for the S. Monitor.
Consequently, you can verify this fix in the following ways:
1. If you only have TD Streaming, run a Streaming chart along side the Strategy Monitor. The opening prices on the Streaming chart should match the open in the S. Monitor.
To compare to historical charts:
2. Use IQFeed Streaming or Streaming Bars.
3. Use IB Streaming Bars..
Aside:
IQFeed Streaming is tick-by-tick and this could be used to compare to historical charts.
Knowing about the conflated ticks issue, we worked tirelessly on integrating Streaming Bars for IB. It paid off. IB's Streaming Bars in the Strategy Monitor precisely match historical charts. I haven't looked to see if TDA has a similar facility, but if they do, we'll get to it.
Edit/Update: TD does have streaming bars. Took a quick look and it appears they come in consistently :04 secs after the minute mark, which is probably both good and bad. Good, because it probably indicates that we're getting the real tick-by-tick minute bar. Bad because it's a 4 second delay. Anyway, we'll get this incorporated into the provider for the S. Monitor.
Consequently, you can verify this fix in the following ways:
1. If you only have TD Streaming, run a Streaming chart along side the Strategy Monitor. The opening prices on the Streaming chart should match the open in the S. Monitor.
To compare to historical charts:
2. Use IQFeed Streaming or Streaming Bars.
3. Use IB Streaming Bars..
@Shaaker,
Give the new TDA Streaming Bars a try in TDA Build 16.
Streaming bars will replicate historical charts at the expense of a couple seconds more delay. (We build the Streaming bars by subscribing to 1-minute trading summary bars that arrive approximately 4.5 seconds after the end of interval.)
fyi, there is one minor error we detected today (fixed for TDA Build 17). If you subscribe to an illiquid symbol that doesn't update every minute, it's possible that a bar will be skipped in higher timeframe if there's no summary bar for the 1-minute period at the end-of-interval.
Give the new TDA Streaming Bars a try in TDA Build 16.
Streaming bars will replicate historical charts at the expense of a couple seconds more delay. (We build the Streaming bars by subscribing to 1-minute trading summary bars that arrive approximately 4.5 seconds after the end of interval.)
fyi, there is one minor error we detected today (fixed for TDA Build 17). If you subscribe to an illiquid symbol that doesn't update every minute, it's possible that a bar will be skipped in higher timeframe if there's no summary bar for the 1-minute period at the end-of-interval.
Thanks for the update and follow-up.
As suggested, I did run the streaming chart along side the S Monitor, and both were showing exactly the same, but wrong open.
That was build 14. I will upgrade to 15 and check again.
Also adding that it is run on a few very liquid symbols.
As suggested, I did run the streaming chart along side the S Monitor, and both were showing exactly the same, but wrong open.
That was build 14. I will upgrade to 15 and check again.
Also adding that it is run on a few very liquid symbols.
You cannot use TD Streaming to check the open for the reasons I stated before (conflated data).
Use Streaming Bars in the Strategy Monitor for TDA Build 16.
Just be mindful of the bug that could occur for illiquid symbols. For example, if you requested 5 minute bars and there was trading during the first 4 minutes, but not the last minute (i.e, :35, :40, :45, etc), this bar will be skipped. That won't happen when Build 17 becomes available.
Use Streaming Bars in the Strategy Monitor for TDA Build 16.
Just be mindful of the bug that could occur for illiquid symbols. For example, if you requested 5 minute bars and there was trading during the first 4 minutes, but not the last minute (i.e, :35, :40, :45, etc), this bar will be skipped. That won't happen when Build 17 becomes available.
Update:
I used Streaming Bars in the Strategy Monitor on a few very liquid symbols. Concurrently, opened a Streaming Chart for one of the symbols. As you can see in the screenshots below, both the Streaming Chart and Strategy Monitor still fail to get the correct open. I am using WL7 build 48, and TD extension build 17.
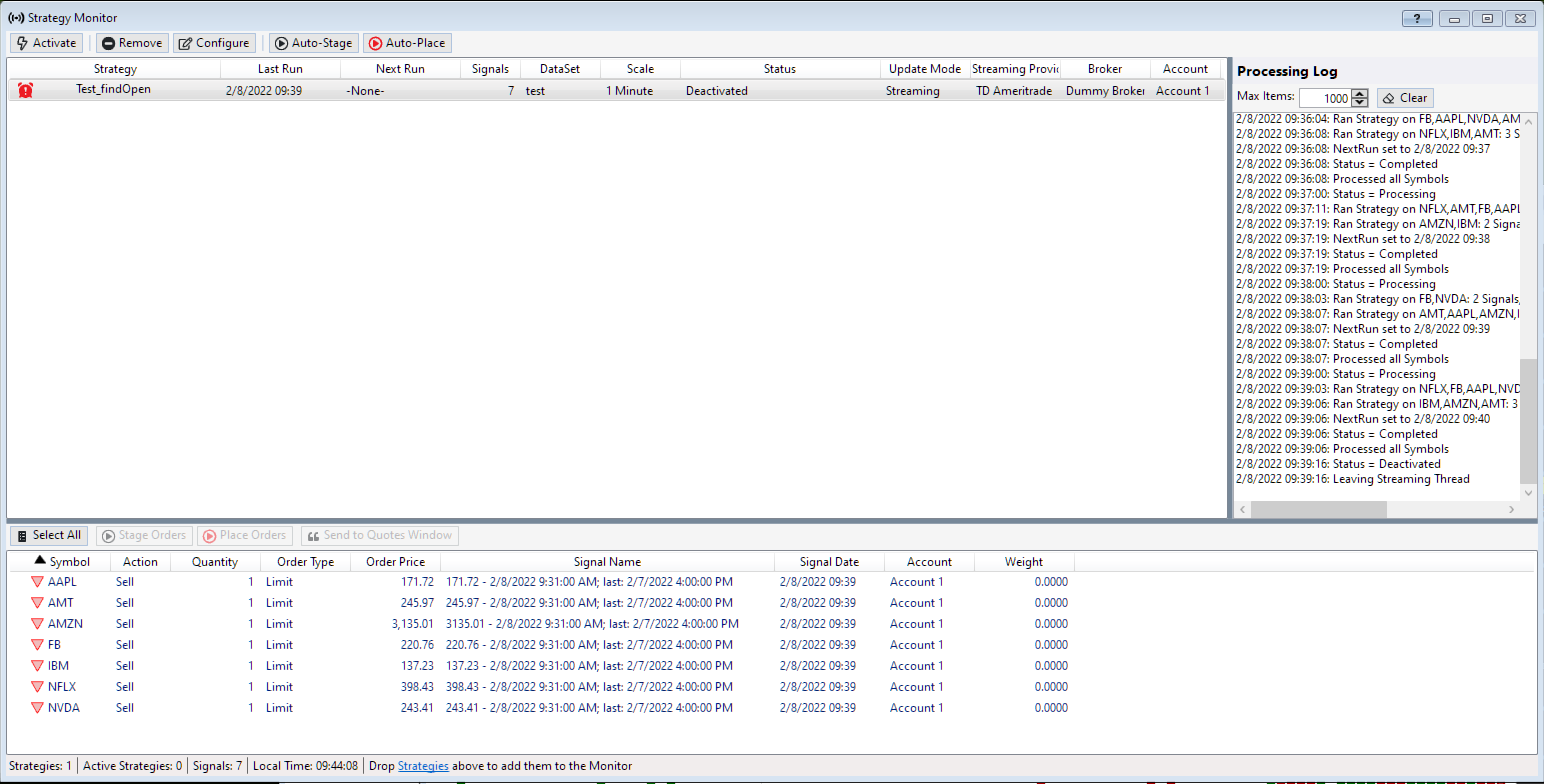
I used Streaming Bars in the Strategy Monitor on a few very liquid symbols. Concurrently, opened a Streaming Chart for one of the symbols. As you can see in the screenshots below, both the Streaming Chart and Strategy Monitor still fail to get the correct open. I am using WL7 build 48, and TD extension build 17.
Thanks for pictures, because it made it easy for me to see what you did wrong. Nonetheless, I'm going to apologize in advance for use of caps to drive the point home because I've said this repeatedly above.
You CANNOT use TDA STREAMING to test this. TDA Streaming provides conflated, not tick-by-tick data, the latter of which are used to build historical charts.
You MUST use Streaming Bars in the Strategy Monitor if you wish to precisely match historical chart data.
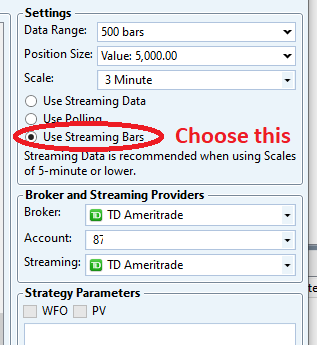
You CANNOT use TDA STREAMING to test this. TDA Streaming provides conflated, not tick-by-tick data, the latter of which are used to build historical charts.
You MUST use Streaming Bars in the Strategy Monitor if you wish to precisely match historical chart data.
Thanks for catching the mistake.
It is now working as expected, and SM returns correct opens when run on 5-min scale.
However, for 1-min scale there seems to be problem which may be from TD's end, but I am sharing here in case you have some insight.
As the screen shot below shows, the first run at 9:31 failed to complete and the second run at 9:32 failed to return opens (except for one symbol), and I have to mention that this happened in both days that I have tested so far.
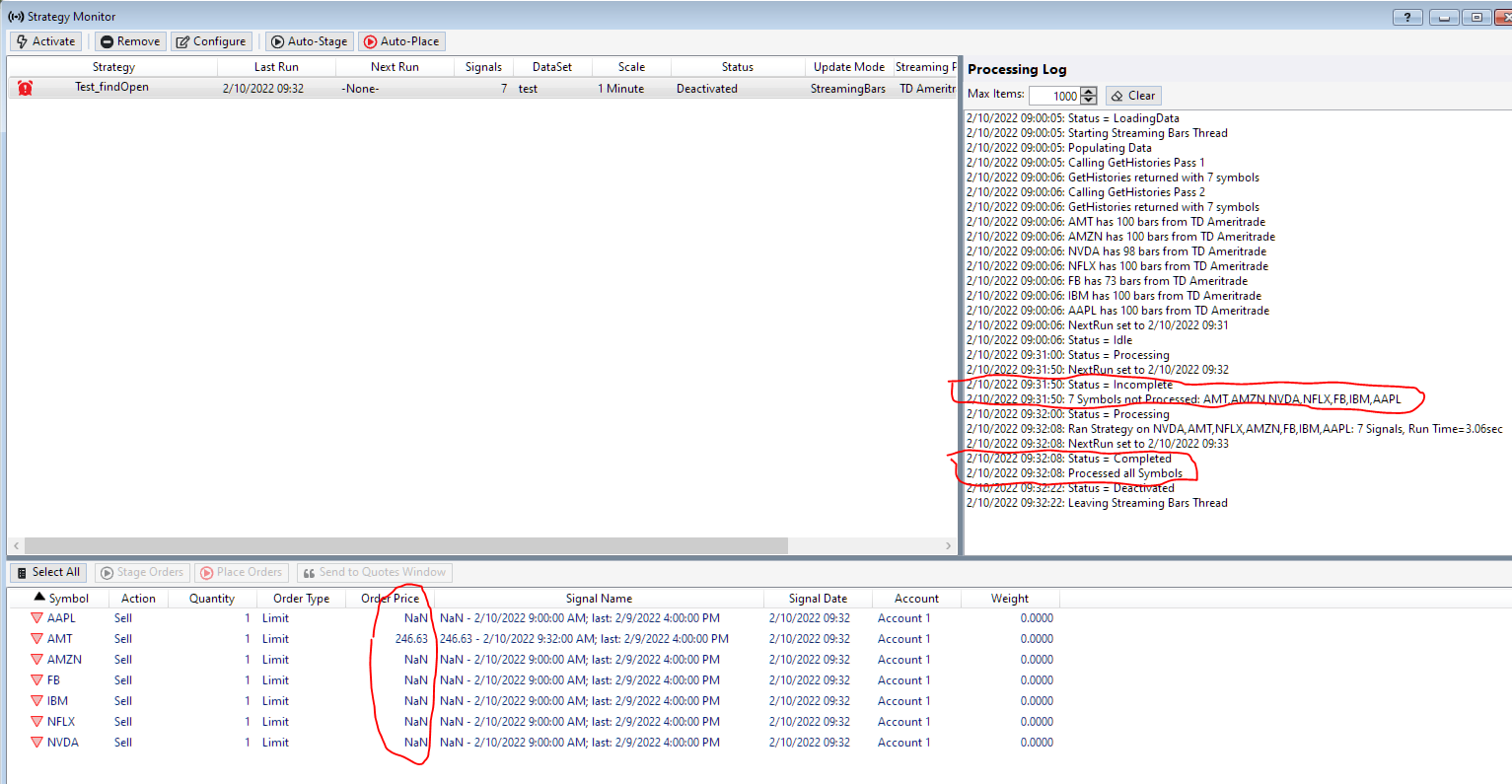
It is now working as expected, and SM returns correct opens when run on 5-min scale.
However, for 1-min scale there seems to be problem which may be from TD's end, but I am sharing here in case you have some insight.
As the screen shot below shows, the first run at 9:31 failed to complete and the second run at 9:32 failed to return opens (except for one symbol), and I have to mention that this happened in both days that I have tested so far.
Okay, thanks. It looks like I'll need to test initializing the 1-minute charts. Right now, I'm not sure why it would be different!
Thank you. Also, I just noticed following messages in the Exception Log which are related to SM run this morning, and thought might be helpful to share.

Thanks, I'll take care of that too.
I've got a handle on the 1-min case and will be testing it in 2 minutes!
I've got a handle on the 1-min case and will be testing it in 2 minutes!
Looks good!
2/11/2022 14:31:00: Status = Processing
2/11/2022 14:31:08: Ran Strategy on CSCO,IBM,GS,DOW,CAT,CVX,AAPL,BA,GE,CRM,HON,HD,DIS,AMGN,AXP: 0 Signals, Run Time=3.48sec
2/11/2022 14:31:08: NextRun set to 2/11/2022 14:32
2/11/2022 14:31:08: Status = Completed
2/11/2022 14:31:08: Processed all Symbols
2/11/2022 14:31:00: Status = Processing
2/11/2022 14:31:08: Ran Strategy on CSCO,IBM,GS,DOW,CAT,CVX,AAPL,BA,GE,CRM,HON,HD,DIS,AMGN,AXP: 0 Signals, Run Time=3.48sec
2/11/2022 14:31:08: NextRun set to 2/11/2022 14:32
2/11/2022 14:31:08: Status = Completed
2/11/2022 14:31:08: Processed all Symbols
Thanks for your continues effort for fixing the TD data issue and releasing the new built 18 for 1-min scale. Unfortunately, even with new updates I still haven't been able to get the opens with 1-min data. I keep testing again next week, but meanwhile wanted to report an exception error which might be a possible culprit:
Recently, I have been getting the following exception when starting WL7 (it only happens randomly, not all the times):
System.Exception: WealthLab.TDAmeritrade
at
.
.
.
(Boolean& )
at
.
.
.
()
at
.
.
[
](Int32 , Object , Object ,
& )
at
.
.
(Int32 , Object , Object )
at
.
.
(Object )
at
.
.
(Int32 )
at WealthLab.TDAmeritrade.TDHistorical.get_Name()
at InterpreterMapper.DefineHttpStruct(Object , InterpreterMapper )
at WealthLab.Data.DataProviderFactory.FindDataProvider(String name)
at WealthLab.Data.DataSetFactory.AssociateDataProviderWithDataSet(DataSet ds, Boolean addedByUser)
at WealthLab.Data.DataSetFactory.MapHttpQueue(Object , Boolean addedByUser)
at WealthLab.Data.DataSetFactory.Initialize()
at InvocationModel.ListState(InvocationModel )
at WealthLab7.MainController..ctor()
at WealthLab7.MainController.CreateInstance()
at WealthLab7.App.ResetRole()
at WealthLab7.App.OnStartup(StartupEventArgs e)
at System.Windows.Application.<.ctor>b__1_0(Object unused)
at System.Windows.Threading.ExceptionWrapper.InternalRealCall(Delegate callback, Object args, Int32 numArgs)
at System.Windows.Threading.ExceptionWrapper.TryCatchWhen(Object source, Delegate callback, Object args, Int32 numArgs, Delegate catchHandler)
Recently, I have been getting the following exception when starting WL7 (it only happens randomly, not all the times):
System.Exception: WealthLab.TDAmeritrade
at
.
.
.
(Boolean& )
at
.
.
.
()
at
.
.
[
](Int32 , Object , Object ,
& )
at
.
.
(Int32 , Object , Object )
at
.
.
(Object )
at
.
.
(Int32 )
at WealthLab.TDAmeritrade.TDHistorical.get_Name()
at InterpreterMapper.DefineHttpStruct(Object , InterpreterMapper )
at WealthLab.Data.DataProviderFactory.FindDataProvider(String name)
at WealthLab.Data.DataSetFactory.AssociateDataProviderWithDataSet(DataSet ds, Boolean addedByUser)
at WealthLab.Data.DataSetFactory.MapHttpQueue(Object , Boolean addedByUser)
at WealthLab.Data.DataSetFactory.Initialize()
at InvocationModel.ListState(InvocationModel )
at WealthLab7.MainController..ctor()
at WealthLab7.MainController.CreateInstance()
at WealthLab7.App.ResetRole()
at WealthLab7.App.OnStartup(StartupEventArgs e)
at System.Windows.Application.<.ctor>b__1_0(Object unused)
at System.Windows.Threading.ExceptionWrapper.InternalRealCall(Delegate callback, Object args, Int32 numArgs)
at System.Windows.Threading.ExceptionWrapper.TryCatchWhen(Object source, Delegate callback, Object args, Int32 numArgs, Delegate catchHandler)
I'm not sure how that exception could occur, but I'll keep an eye out for it.
I'll check the 1-min streaming bars again, but I did test it several times to ensure the 1-minute bars were returning all the data. Here are the results for a test with AAPL more than a week ago. The test strategy just saves the OHLC/V to a text file.
In any case, we've got another installment for the historical and streaming providers ready to go that supports futures (probably will get Forex working too).
I'll check the 1-min streaming bars again, but I did test it several times to ensure the 1-minute bars were returning all the data. Here are the results for a test with AAPL more than a week ago. The test strategy just saves the OHLC/V to a text file.
CODE:
20220211 0931 172.33 172.75 172.27 172.5925 977315.0 20220211 0932 172.60 173.08 172.59 172.76 370999.0 20220211 0933 172.75 172.87 172.4401 172.565 323359.0 20220211 0934 172.56 172.5701 172.3107 172.49 227130.0 20220211 0935 172.48 172.48 172.17 172.2271 234067.0 20220211 0936 172.21 172.26 171.88 172.01 381165.0
In any case, we've got another installment for the historical and streaming providers ready to go that supports futures (probably will get Forex working too).
Looks good for me today too. How about for you?
Here are the first few bars for -
Here are the first few bars for -
CODE:Remember, the strategy outputs these OHLC/V data for the bar timestamp indicated.
//BA 20220222 0931 205.00 206.90 204.2601 204.84 216863.0 20220222 0932 204.82 205.0999 203.85 204.155 43736.0 20220222 0933 204.02 204.0807 202.88 203.82 72185.0 //CRM 20220222 0931 195.53 196.74 195.00 196.72 244983.0 20220222 0932 196.65 197.37 196.42 196.9251 30309.0 20220222 0933 197.16 197.16 196.50 196.87 10444.0 //GS 20220222 0931 342.46 343.43 341.56 343.43 48899.0 20220222 0932 343.43 343.69 342.73 343.005 7048.0 20220222 0933 343.04 343.60 342.43 342.555 5730.0
Thanks for following up. Unfortunately, I was not able to run it today, but hopefully will test and provide an update in the coming days.
I was just looking at your original code and couldn't really figure out how you were getting a Signal without assigning a Quantity for the transaction. In any case, I'd recommend you change that line of code to the following to make it easy to copy the result from the Log and not unnecessarily execute PlaceTrade except for the last bar when it counts.
CODE:
if (idx == bars.Count - 1) { string msg = String.Format("{0}: startBarToday: {1:yyyyMMdd HHmm}; Open: {2:N2} ", bars.Symbol, bars.DateTimes[startBarToday], todayOpen); WLHost.Instance.AddLogItemOrders("Opening Bar Check", msg, Color.Black); Transaction t = PlaceTrade(bars, TransactionType.Sell, OrderType.Limit, todayOpen, String.Format("{0:f2} -", todayOpen) + bars.DateTimes[startBarToday].ToString()); t.Quantity = 1; }
I have been using the modified script posted on Jan 13 (above) which specifies the Quantity.
Also, today's run returned the same results: it works for 5-min, but not 1-min.
For the next run I will use the code you just posted and provide update.
Also, today's run returned the same results: it works for 5-min, but not 1-min.
For the next run I will use the code you just posted and provide update.
Maybe I missed something in the Release build 18 that I had changed locally while working on something else.
I'll check it today with the Release build 18, and in any case, I'll see if the team can push out TD Build 19 today or Monday.
I'll check it today with the Release build 18, and in any case, I'll see if the team can push out TD Build 19 today or Monday.
I ran full release mode today with Build 18. Worked perfectly. If it didn't for you, snap a picture of your set up, please.
In any case, TD Build 19 is ready to go out with WL Build 50, probably later today.
In any case, TD Build 19 is ready to go out with WL Build 50, probably later today.
Here are the screenshots from this morning run (using the latest scrip that generates the log) which still cannot get the correct opens. Currently I have WL build 48, and TD build 18.
Also, occasionally I still get the TD exception error that I mentioned before, and I'd like to believe that somehow this is related to this problem.
Another thing I noticed and as you can see in the second screenshot, the symbol AMT does not show up in the Log Viewer
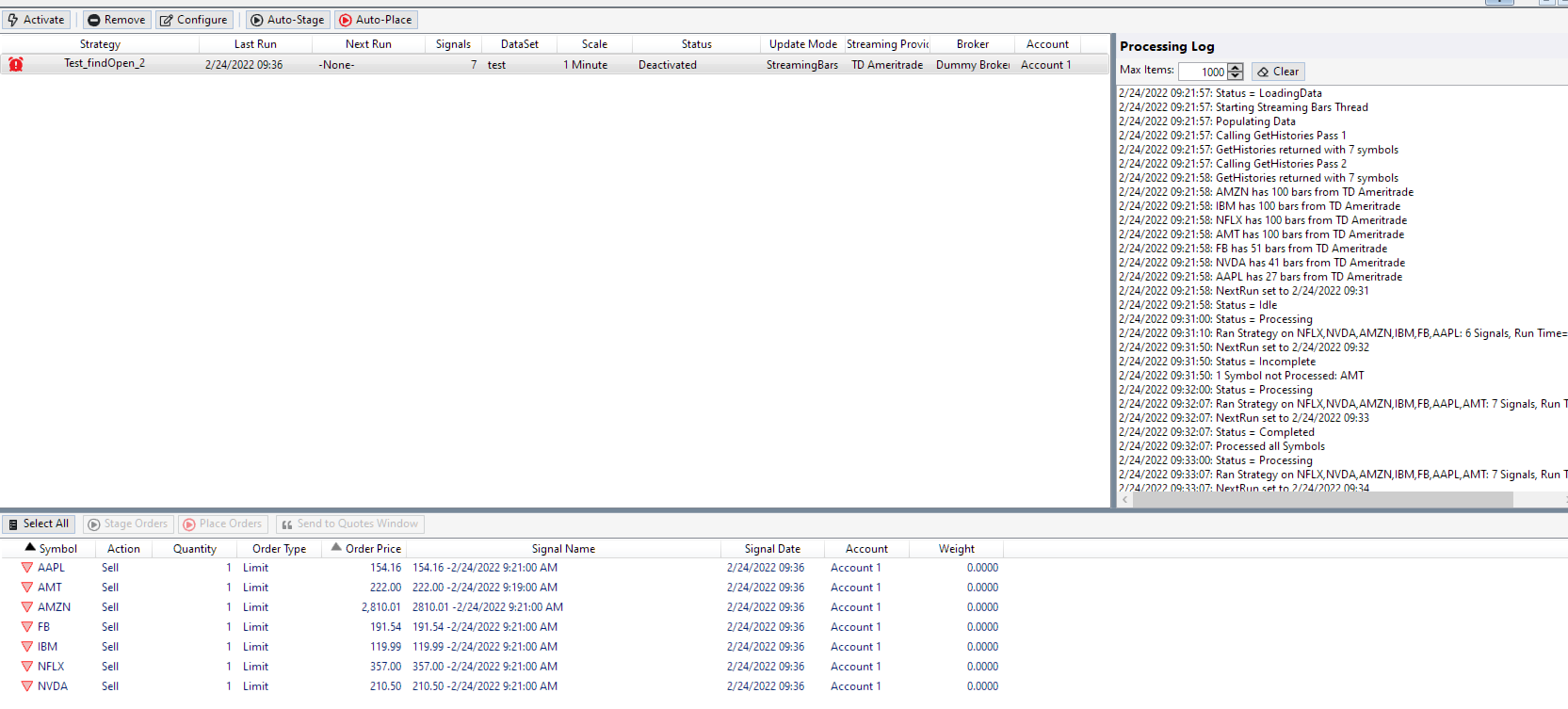
Also, occasionally I still get the TD exception error that I mentioned before, and I'd like to believe that somehow this is related to this problem.
Another thing I noticed and as you can see in the second screenshot, the symbol AMT does not show up in the Log Viewer
I've never seen that exception and without it actually occurring, I don't see how it could even occur.
Why does it look like you're getting 15-minute delayed data? The alert that occurred at 09:36 is showing first bar data for 09:21 - VERY odd! At a minimum, this indicates that you're not using the market filter. See Post #7.
Deactivate the Strategy, and close the S. Monitor. Open and save that Strategy with the Market filter enabled for the next test on Monday. install Wealth-Lab Build 50 and TD Build 19 for next time.
Edit:
I switched time zones while traveling.. there's time for another try today!
Why does it look like you're getting 15-minute delayed data? The alert that occurred at 09:36 is showing first bar data for 09:21 - VERY odd! At a minimum, this indicates that you're not using the market filter. See Post #7.
Deactivate the Strategy, and close the S. Monitor. Open and save that Strategy with the Market filter enabled for the next test on Monday. install Wealth-Lab Build 50 and TD Build 19 for next time.
Edit:
I switched time zones while traveling.. there's time for another try today!
Robert, here's another case of the exception from Post #28:
https://www.wealth-lab.com/Discussion/7658
Sometimes it turns into a startup failure.
https://www.wealth-lab.com/Discussion/7658
Sometimes it turns into a startup failure.
First I should say that the Filter has been always selected since the beginning of our conversation in these posts.
On the seemingly 15 minutes delay, I should point out that 9:21 is exactly the time that the strategy was activated in SM (can be seen in the Processing Log section), and by checking the data I could confirm that the Opens reported are in fact the open of that bar (9:21).
Now, even if we assume that the Filter is not working for some reason, then why does the SM take the activation time as the first bar of the day and not the first bar of the 100 preloaded bars? Another important question would be why the the same strategy run on 5-minute scale does not generate any of these problem and works correctly.
On the seemingly 15 minutes delay, I should point out that 9:21 is exactly the time that the strategy was activated in SM (can be seen in the Processing Log section), and by checking the data I could confirm that the Opens reported are in fact the open of that bar (9:21).
Now, even if we assume that the Filter is not working for some reason, then why does the SM take the activation time as the first bar of the day and not the first bar of the 100 preloaded bars? Another important question would be why the the same strategy run on 5-minute scale does not generate any of these problem and works correctly.
Hello,
I am re-opening this post to report that the problem discussed here still persists in WL7 and also in WL8.
In summary, I am using the following code on 1-min data in SM (with 100 bars as Data Range) to determine the market open. The historical and streaming data provider is TD. The "Filter Pre/Post Market Data" is checked and saved with the strategy. As shown in the snapshots below both the "Streaming Bars" and "Streaming Data" have been tested where the first one cannot generate any signal, and the second one cannot get the correct market opens (as expected since it is not the right setting selection)
It is important to note that this issue exist when the strategy in SM is Activated before 9:30 EST.


I am re-opening this post to report that the problem discussed here still persists in WL7 and also in WL8.
In summary, I am using the following code on 1-min data in SM (with 100 bars as Data Range) to determine the market open. The historical and streaming data provider is TD. The "Filter Pre/Post Market Data" is checked and saved with the strategy. As shown in the snapshots below both the "Streaming Bars" and "Streaming Data" have been tested where the first one cannot generate any signal, and the second one cannot get the correct market opens (as expected since it is not the right setting selection)
It is important to note that this issue exist when the strategy in SM is Activated before 9:30 EST.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Drawing; using WealthLab.ChartWPF; using System.Collections.Generic; using System.IO; namespace WealthScript2 { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { lastBarYesterday = startBarToday = 0; // StartIndex = bars.Count - 400; } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (bars.IsLastBarOfDay(idx)) { lastBarYesterday = idx; DrawBarAnnotation("L", idx, true, WLColor.Black, 14); } if (bars.IsFirstBarOfDay(idx)) { startBarToday = idx; DrawBarAnnotation("O", idx, true, WLColor.Black, 14); } yesterdayClose = bars.Close[lastBarYesterday]; todayOpen = bars.Open[startBarToday]; if (idx == bars.Count - 1) { string msg = String.Format("{0}: startBarToday: {1:yyyyMMdd HHmm}; Open: {2:N2} ", bars.Symbol, bars.DateTimes[startBarToday], todayOpen); WLHost.Instance.AddLogItemOrders("Opening Bar Check", msg, WLColor.Black); Transaction t = PlaceTrade(bars, TransactionType.Sell, OrderType.Limit, todayOpen, String.Format("{0:f2} -", todayOpen) + bars.DateTimes[startBarToday].ToString()); t.Quantity = 1; } } //declare private variables below int startBarToday; int lastBarYesterday; double todayOpen; double yesterdayClose; } }
Are you saying the "today's open" values are incorrect? Let me check.
OK, I see some slight differences in the open price in your signals and the reported open price in the TD one minute chart. I'll need to run this tomorrow at market open and see what's going on, it looks like the first streaming tick received from TD doesn't exactly correspond to the eventual historical data one minute bar for the first bar of the day.
OK, I see some slight differences in the open price in your signals and the reported open price in the TD one minute chart. I'll need to run this tomorrow at market open and see what's going on, it looks like the first streaming tick received from TD doesn't exactly correspond to the eventual historical data one minute bar for the first bar of the day.
Yes, and also:
1. they are not the same between WL7 and WL8.
2. using "Streaming Bars" option in the Settings did not generate any signals
Just reminding that for duplicating the issue the strategy in SM must be Activated before 9:30
1. they are not the same between WL7 and WL8.
2. using "Streaming Bars" option in the Settings did not generate any signals
Just reminding that for duplicating the issue the strategy in SM must be Activated before 9:30
TD uses conflated data in their stream, so it’s not surprising actually. It would probably be slightly different each instance. You could try polling but it might introduce too much lag on a 1 minute scale. IQFeed streaming would be much more reliable.
This point was discussed in the earlier posts of this thread, and using the "Streaming Bars" was supposed to be the solution but apparently it is not.
Additionally, if the conflated data is the culprit then how come there are other scenarios under which the issue does not occur and correct Opens are returned:
1. if the strategy is Activated in SM any time after 9:31am EST
2. if the strategy is Activated in SM before 9:30am EST but on 5-min data
Additionally, if the conflated data is the culprit then how come there are other scenarios under which the issue does not occur and correct Opens are returned:
1. if the strategy is Activated in SM any time after 9:31am EST
2. if the strategy is Activated in SM before 9:30am EST but on 5-min data
QUOTE:Because if you activate after the interval, the historical data is loaded for that bar.
1. if the strategy is Activated in SM any time after 9:31am EST
QUOTE:This reminds me that we discovered a problem in our Streaming Bar Builder with "completing" 1-minute intervals during the premarket session. That was corrected in Wealth-Lab Build 14. I'm pretty sure this only applied to pre-market, but just to sure, are you using WL Build 14?
2. if the strategy is Activated in SM before 9:30am EST but on 5-min data
Maybe there's still an initialization issue with 1-minute bars we need to nail down. We'll check it today.
My test this morning showed that every Dow 30 symbol that I checked (6 of them) streaming bar open in the S. Monitor precisely matched the open of the first 1-minute bar on the chart, i.e., the 0931 bar.
If you're seeing something different, you'll have to show me precisely how you're capturing the data.
If you're seeing something different, you'll have to show me precisely how you're capturing the data.
QUOTE:
are you using WL Build 14?
Yes. Both WL7 & 8, and also the extensions are the latest builds.
I will run it again tomorrow using "Streaming Bars" on a small dataset of highly liquid symbols (instead of DOW - only for simplifying further) and will share the Log Viewer and the S.M. Processing Log.
Is there is anything else that you would need to see?
I don't need the Logs. Let me just give you the script I use to verify the data entering the S. Monitor - it creates a Data\StrategyMonitorTesting\n-Min folder in the User Data Folder and writes the last bar's information to a file for each instrument. You can use these files to compare with chart data later.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using System.IO; namespace WealthScript123 { public class LastBarToFile : UserStrategyBase { public override void Initialize(BarHistory bars) { const string tab = "\t"; const string fmt = "0.00####"; // bars.Scale.Interval is 1 for Daily+ string subfolder = bars.Scale.IsIntraday ? bars.Scale.Interval + "-MIN" : "Daily"; string path = Path.Combine(WLHost.Instance.DataFolder, "Data\\StrategyMonitorTesting\\" + subfolder); if (!Directory.Exists(path)) { Directory.CreateDirectory(path); } string file = bars.Symbol.Replace('/', '_') + ".txt"; string filename = Path.Combine(path, file); System.IO.StreamWriter csv = new System.IO.StreamWriter(filename, true); /* write the BarCount, local time, bar timestamp, and OHLC/V of the last bar to the file */ int bar = bars.Count - 1; string line = bars.Count.ToString() + tab + DateTime.Now.ToString("HH:mm:ss.fff") + tab + bars.DateTimes[bar].ToString("yyyyMMdd HHmm") + tab + bars.Open[bar].ToString(fmt) + tab + bars.High[bar].ToString(fmt) + tab + bars.Low[bar].ToString(fmt) + tab + bars.Close[bar].ToString(fmt) + tab + bars.Volume[bar].ToString("0.0#######"); csv.WriteLine(line); csv.Close(); csv = null; } public override void Execute(BarHistory bars, int idx) { } } }
Thank you.
I tested the script and the generated files had correct data including the opens. I have to further investigate as to why the other script doesn't work (it might be due to a intermittent connection problem with TD from my computer). I will provide update on any progress.
I tested the script and the generated files had correct data including the opens. I have to further investigate as to why the other script doesn't work (it might be due to a intermittent connection problem with TD from my computer). I will provide update on any progress.
Sounds good.
btw, TDA streaming bars are 1-minute bars, so they go straight into the chart without manipulation. n-minute intervals are compiled from the 1-minute streaming bars, so if there's an interruption/error in receiving one of the sets of 1-minute data, it could affect the data of an n-minute bar.
btw, TDA streaming bars are 1-minute bars, so they go straight into the chart without manipulation. n-minute intervals are compiled from the 1-minute streaming bars, so if there's an interruption/error in receiving one of the sets of 1-minute data, it could affect the data of an n-minute bar.
Update:
I was able to find the culprit for the intermittent connection problem with TD. Apparently it was related to running S.M. simultaneously in both WL7 and 8. Running WL8 alone this morning worked without any problem.
More importantly I wanted to share my finding about the script (Post #39) which does not return correct Market Opens - despite having confirmation from the text files (Post #47 & 48) that the data is correct. It seems that in this instance the method bars.IsFirstBarOfDay is not working properly; because replacing the:
with
solves the problem.
I was able to find the culprit for the intermittent connection problem with TD. Apparently it was related to running S.M. simultaneously in both WL7 and 8. Running WL8 alone this morning worked without any problem.
More importantly I wanted to share my finding about the script (Post #39) which does not return correct Market Opens - despite having confirmation from the text files (Post #47 & 48) that the data is correct. It seems that in this instance the method bars.IsFirstBarOfDay is not working properly; because replacing the:
CODE:
if (bars.IsFirstBarOfDay(idx)) startBarToday = idx;
with
CODE:
if((bars.DateTimes[idx].Hour == 9) && (bars.DateTimes[idx].Minute == 31)) startBarToday = idx;
solves the problem.
QUOTE:
...intermittent connection problem with TD. Apparently it was related to running S.M. simultaneously in both WL7 and 8.
Thanks for the update. Who would have thought of such scenario in action.
QUOTE:The problem is that you're not using the market hours data filter. For the Strategy Monitor, make sure that you save the Strategy with the Strategy Settings > Filter Pre/Post (checked) if that's what you want.
It seems that in this instance the method bars.IsFirstBarOfDay is not working properly because replacing the:
I assure you that the Filter has been always checked and saved with the strategy (as I have pointed out in previous posts), but seemingly did not help fixing the problem. I have even made sure that the similar filter in Preferences>Data>Data in Charts has been always selected.
Okay, we'll take a closer look at that then. Thanks.
Thanks for the TD extension update with the fix.
Your Response
Post
Edit Post
Login is required