I have a strategy which plots the summary of the dataset built in PreExecute() in the top pane. In WL7 the pane shows the plotted lines, but WL8 does not. Is this the appropriate way to plot values calculated n PreExecute()?
Dataset contents: XLB XLC XLE XLF XLI XLK XLP XLRE XLU XLV XLY
WL7 Chart:
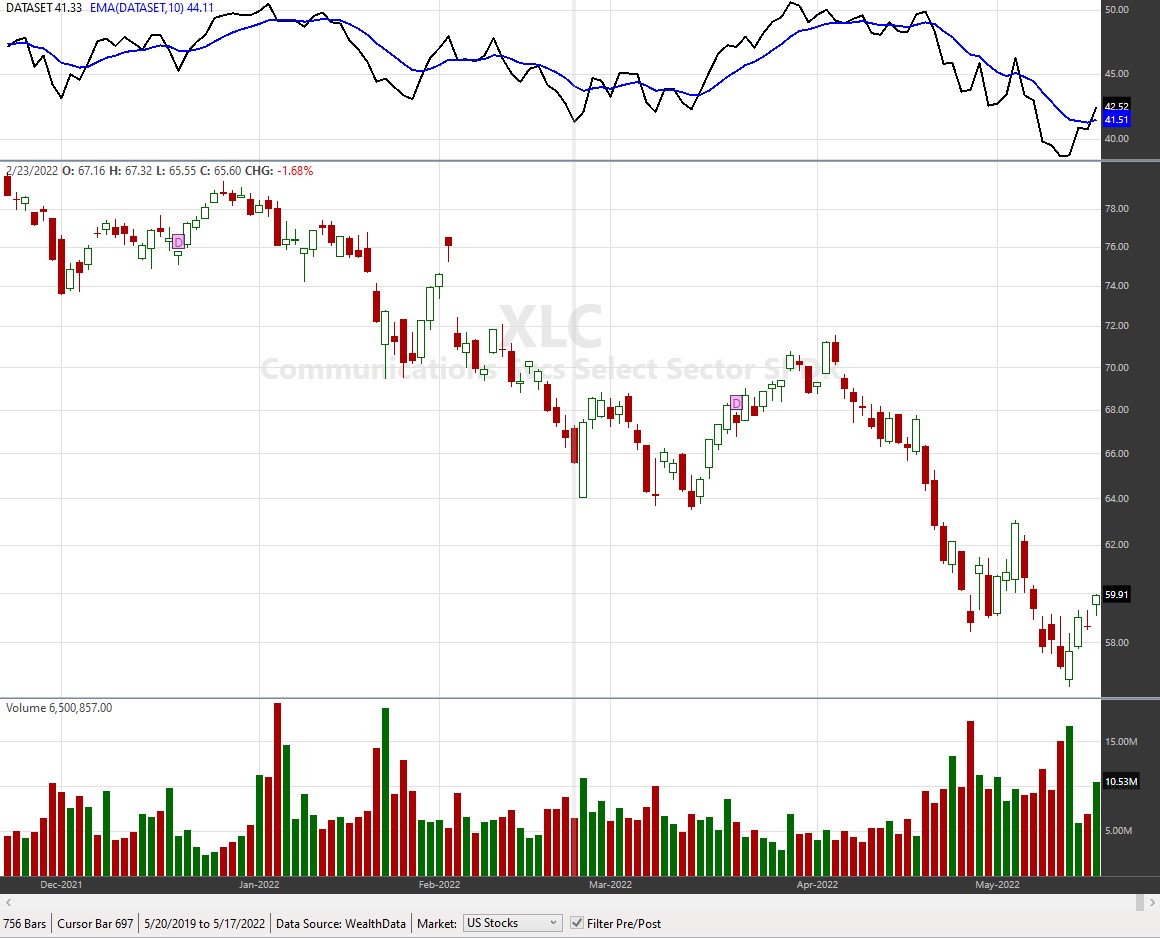
WL8 Chart:
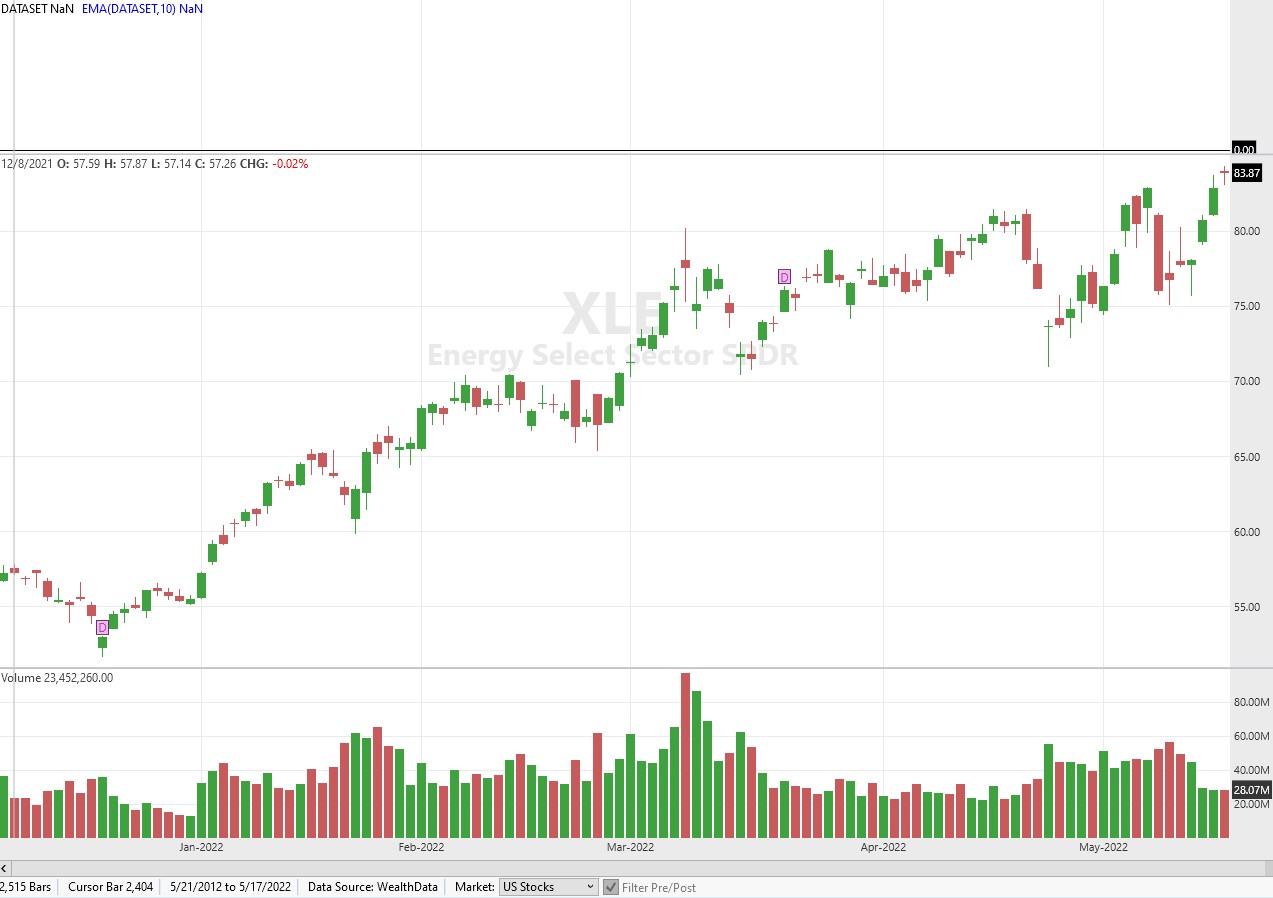
WL8 Code:
Dataset contents: XLB XLC XLE XLF XLI XLK XLP XLRE XLU XLV XLY
WL7 Chart:
WL8 Chart:
WL8 Code:
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Drawing; using System.Collections.Generic; namespace WealthScript2 { public class MyStrategy : UserStrategyBase { private TimeSeries _tsSymDataset; private IndicatorBase _tsSymDatasetMa; private bool IsFirstPreExecute = true; public MyStrategy() { } //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { TimeSeries tsSymBarChg = GetCachedSymbolBarChange(bars); _tsSymDataset = new TimeSeries(bars.DateTimes); // Will be updated in Execute using PreExecute values _tsSymDataset.Description = "DATASET"; _tsSymDatasetMa = EMA.Series(_tsSymDataset, 10); SetPaneDrawingOptions("DATASET", 30, -1); DrawHorzLine(0.0, WLColor.Black, 1, LineStyle.Solid, "DATASET"); PlotTimeSeriesLine(_tsSymDataset, _tsSymDataset.Description, "DATASET", WLColor.Black, 2, LineStyle.Solid); PlotTimeSeriesLine(_tsSymDatasetMa, _tsSymDatasetMa.Description, "DATASET", WLColor.Blue, 2, LineStyle.Solid); WriteToDebugLog(string.Concat("First historical date: ", bars.DateTimes[0].ToShortDateString())); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (idx == StartIndex) { WriteToDebugLog(string.Concat("Execute start date: ", bars.DateTimes[idx].ToShortDateString(), " (", idx.ToString(), ")")); } int bidx = _tsDataset.IndexOf(bars.DateTimes[idx]); _tsSymDataset[idx] = _tsDataset[bidx]; _tsSymDatasetMa.Populate(); if (!HasOpenPosition(bars, PositionType.Long)) { //code your buy conditions here } else { //code your sell conditions here } } #region Basket build logic private static TimeSeries _tsDataset; // For Basket logic public override void BacktestBegin() { _tsDataset = new TimeSeries(); _tsDataset.Description = "Dataset"; _tsDataset.FirstValidIndex = 1; } // called once per bar passed list of all symbols public override void PreExecute(DateTime dt, List<BarHistory> participants) { if (IsFirstPreExecute) { WriteToDebugLog(string.Concat("PreExecute first date: ", dt.ToShortDateString())); IsFirstPreExecute = false; } AddBasketValue(dt, participants); } private void AddBasketValue(DateTime dt, List<BarHistory> participants) { double BasketBarChg = 0.0; double SymCnt = 0.0; foreach (BarHistory bh in participants) { int idx = GetCurrentIndex(bh); TimeSeries tsSymBarChg = GetCachedSymbolBarChange(bh); if (idx < tsSymBarChg.FirstValidIndex) continue; BasketBarChg += tsSymBarChg[idx]; SymCnt += 1.0; } if (SymCnt > 0) { BasketBarChg /= SymCnt; if (_tsDataset.Count > 0) BasketBarChg += _tsDataset[_tsDataset.Count - 1]; } _tsDataset.Add(BasketBarChg, dt); } private TimeSeries GetCachedSymbolBarChange(BarHistory bh) { const string KEY_SYMBARCHG = "SymBarChg"; TimeSeries tsSymBarChg = null; if (bh.Cache.TryGetValue(KEY_SYMBARCHG, out object value)) { tsSymBarChg = (TimeSeries)value; } else { tsSymBarChg = ROC.Series(bh.Close, 1); bh.Cache[KEY_SYMBARCHG] = tsSymBarChg; } return tsSymBarChg; } public override void BacktestComplete() { _tsDataset = null; } #endregion } }
Rename
The code must have changed between WL7 and WL8. It was never possible to plot a series in Initialize with the values you insert during Execute. Put your plotting in the BacktestComplete method -
CODE:
public override void BacktestComplete() { SetPaneDrawingOptions("DATASET", 30, -1); DrawHorzLine(0.0, WLColor.Black, 1, LineStyle.Solid, "DATASET"); PlotTimeSeriesLine(_tsSymDataset, _tsSymDataset.Description, "DATASET", WLColor.Black, 2, LineStyle.Solid); PlotTimeSeriesLine(_tsSymDatasetMa, _tsSymDatasetMa.Description, "DATASET", WLColor.Blue, 2, LineStyle.Solid); _tsDataset = null; }
When I moved it to BacktestComplete() the pane disappeared as well as the plotted series. I was able to get the pane and lines to appear by overriding the Cleanup() method.
CODE:
public override void Cleanup(BarHistory bars) { SetPaneDrawingOptions("DATASET", 30, -1); DrawHorzLine(0.0, WLColor.Black, 1, LineStyle.Solid, "DATASET"); PlotTimeSeriesLine(_tsSymDataset, _tsSymDataset.Description, "DATASET", WLColor.Black, 2, LineStyle.Solid); PlotTimeSeriesLine(_tsSymDatasetMa, _tsSymDatasetMa.Description, "DATASET", WLColor.Blue, 2, LineStyle.Solid); }
Your Response
Post
Edit Post
Login is required