I need to know how to have a different target for each position that i open in this strategy, i want a target of 10% for each one of them, how can i do this ?
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; namespace BnHWP { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { kUp = new KeltnerUpper(bars, 10); kLower = new KeltnerLower(bars, 10); PlotIndicatorLine(kUp, WLColor.Red); PlotIndicatorLine(kLower, WLColor.Green); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (HasOpenPosition(bars, PositionType.Long)) { } if (bars.Low[idx] <= kLower[idx]) { PlaceTrade(bars, TransactionType.Buy, OrderType.Market, signalName: "Buy"); } } //declare private variables below IndicatorBase kUp, kLower; double targetPrice; //Transaction t; } }
Rename
Please edit and enclose your code in a code-description block so it gets formatted accordingly. Readers can't follow your code unless it's formatted appropriately.
By "target" are we talking about symbols (i.e. target symbols) or profit (i.e. a profit target that will sell the position)? Or some other target?
By 10%, I assume we are talking about capital allocation, right?
By "target" are we talking about symbols (i.e. target symbols) or profit (i.e. a profit target that will sell the position)? Or some other target?
By 10%, I assume we are talking about capital allocation, right?
Your topic title ("Buy and have a diferrent target for each position") sounded misleading, giving an impression that you'd like to open multiple positions based on different conditions which I presume is not true. Further, the profit target is the SAME (10%) and so isn't different. Let's rename the topic as a "Profit target 10% for each position" then.
For a single position you could use something like this. For multiple positions, you should provide additional info as neither the code nor the question itself contained any insight into their rules.
For a single position you could use something like this. For multiple positions, you should provide additional info as neither the code nor the question itself contained any insight into their rules.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; namespace BnHWP { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { kUp = new KeltnerUpper(bars, 10); kLower = new KeltnerLower(bars, 10); PlotIndicatorLine(kUp, WLColor.Red); PlotIndicatorLine(kLower, WLColor.Green); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { Position foundPosition0 = FindOpenPosition(0); if (foundPosition0 == null) { if (bars.Low[idx] <= kLower[idx]) PlaceTrade(bars, TransactionType.Buy, OrderType.Market, signalName: "Buy"); } else { ClosePosition(foundPosition0, OrderType.Limit, foundPosition0.EntryPrice * 1.10, "Sell at 10% profit tgt"); } } //declare private variables below IndicatorBase kUp, kLower; double targetPrice; //Transaction t; } }
You'll want to include some kind of criteria for exiting loosing positions as well; otherwise, those loosing positions will continue to drag your portfolio down.
I apologize for expressing myself wrong, I wanted to know how to make each position have a target of 10% profit in a system that has multiple positions.
It's no problem, but you have to manage each position in your code. A block strategy with the "Multiple Positions" radio button check will do that for you. Just create it and If you want to see how it's done, click the Open as C# Code button
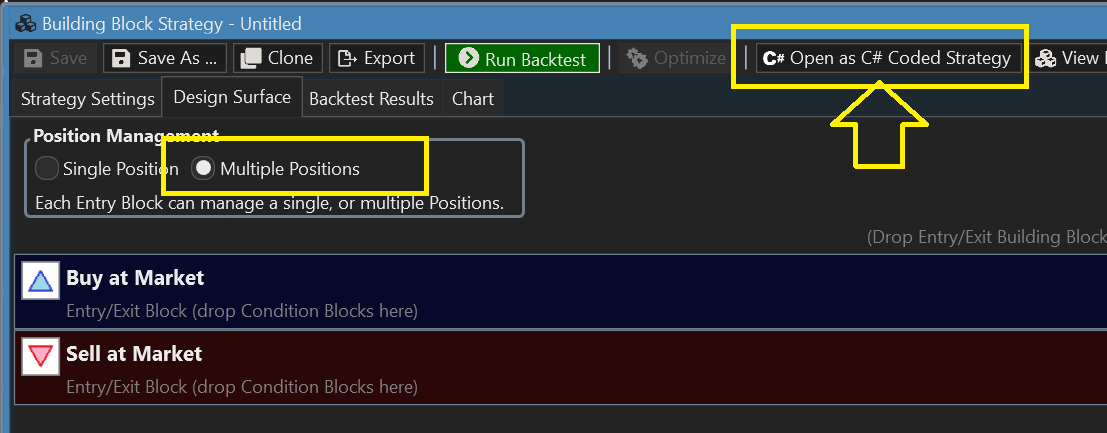
Your Response
Post
Edit Post
Login is required