Suppose I want to exit of on close if whatever condition happens at the close.
How would I do that ?
Thank you.
How would I do that ?
Thank you.
Rename
Building Blocks or C# Code?
C Code.
I want to peek and trade.
Thanks
Thanks
Here is a minimal example. To learn how to trade such a system on daily data in the Strategy Monitor see the Build 99 video on our Youtube channel.
https://youtu.be/Z39A55OEudM
https://youtu.be/Z39A55OEudM
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { rsi4 = RSI.Series(bars.Close, 4); PlotIndicator(rsi4); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (idx == bars.Count - 1) return; if (!HasOpenPosition(bars, PositionType.Long)) { if (rsi4[idx + 1] < 20) PlaceTrade(bars, TransactionType.Buy, OrderType.MarketClose); } else { if (rsi4[idx + 1] > 70) PlaceTrade(bars, TransactionType.Sell, OrderType.MarketClose); } } //declare private variables below private RSI rsi4; } }
Thanks VM!
How do I close on same day close.
So peek on open
and close on close of idx not idx+ 1
Thanks.
So peek on open
and close on close of idx not idx+ 1
Thanks.
The code I shared above already peeks 1 bar ahead and exits at the close.
I have run your code and I have been unable to find an instance where a trade closes on the same day that the opening trade went on. There are a lot of trades, but I have scrolled down lets say 2 or 3 pages.
Thanks. Sorry if did not ask the question correctly.
Buy or short on open. sell or cover on close.
Thanks again.
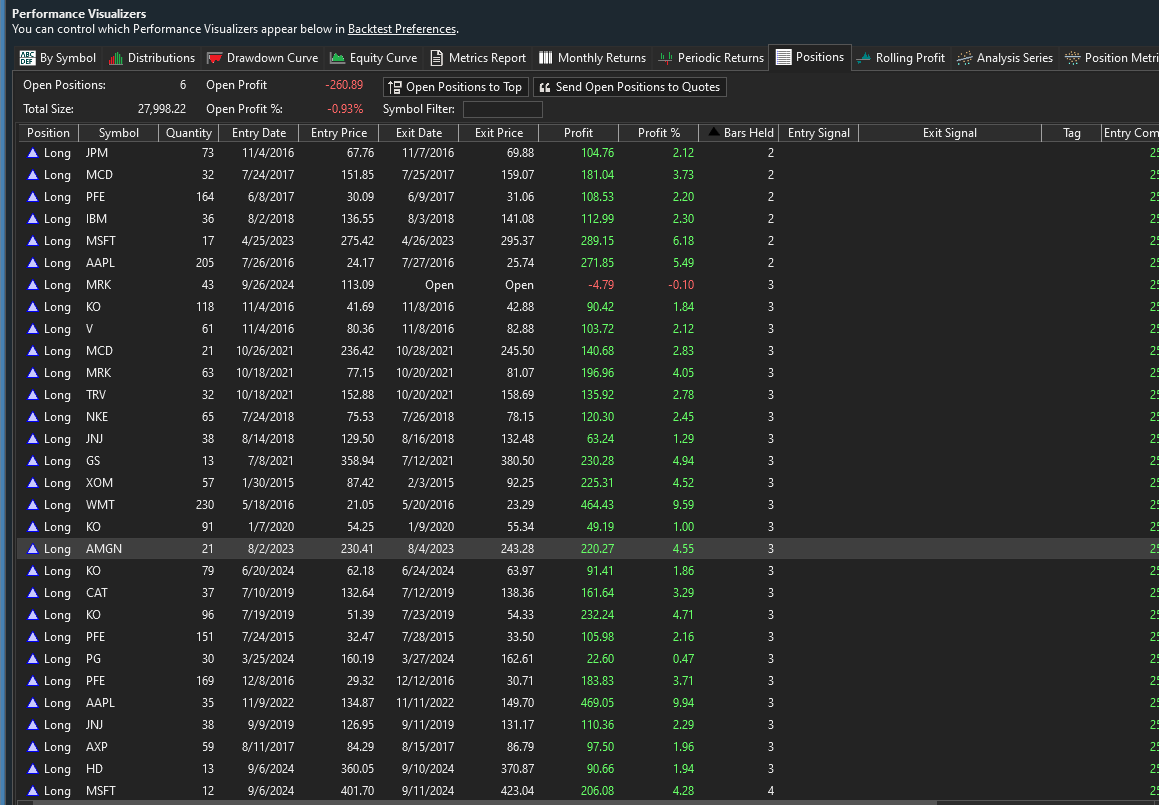
Thanks. Sorry if did not ask the question correctly.
Buy or short on open. sell or cover on close.
Thanks again.
The RSI4 will never close above 70 AND below 20 on the same day, so it will never happen. If you want to learn about WL8’s support for same bar exits you can watch this video.
I’ll try and mock up your revised rules tomorrow 👍
https://www.youtube.com/watch?v=eLHjUpUFGf8
I’ll try and mock up your revised rules tomorrow 👍
https://www.youtube.com/watch?v=eLHjUpUFGf8
Here's an implementation of the rules:
- Buy next bar at market open if RSI4 < 20
- Sell next bar at market close if that bar's RSI > 70 or the trade's profit is 1% or more.
Running with TQQQ here is a cluster of trades that exited on the same bar as entry.
- Buy next bar at market open if RSI4 < 20
- Sell next bar at market close if that bar's RSI > 70 or the trade's profit is 1% or more.
Running with TQQQ here is a cluster of trades that exited on the same bar as entry.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; using ScottPlot.Plottable; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { rsi4 = RSI.Series(bars.Close, 4); PlotIndicator(rsi4); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { if (idx == bars.Count - 1) return; if (!HasOpenPosition(bars, PositionType.Long)) { //code your buy conditions here if (rsi4[idx] < 20) { entryPrice = bars.Open[idx + 1]; PlaceTrade(bars, TransactionType.Buy, OrderType.Market); PlaceExits(bars, idx); } } else { //code your sell conditions here PlaceExits(bars, idx); } } //place exit orders, peek one bar ahead private void PlaceExits(BarHistory bars, int idx) { //exit at close when trade has 1% profit, even on entry bar, or RSI4 closes above 70 double profit = (bars.Close[idx + 1] - entryPrice) * 100 / entryPrice; if (profit > 1 || rsi4[idx + 1] > 70) PlaceTrade(bars, TransactionType.Sell, OrderType.MarketClose); } //declare private variables below private RSI rsi4; private double entryPrice; } }
Thanks.
I don't want a closing condition. I want the trade to CLOSE on the Close of the bar that I Opened it in. Not subject to conditions.
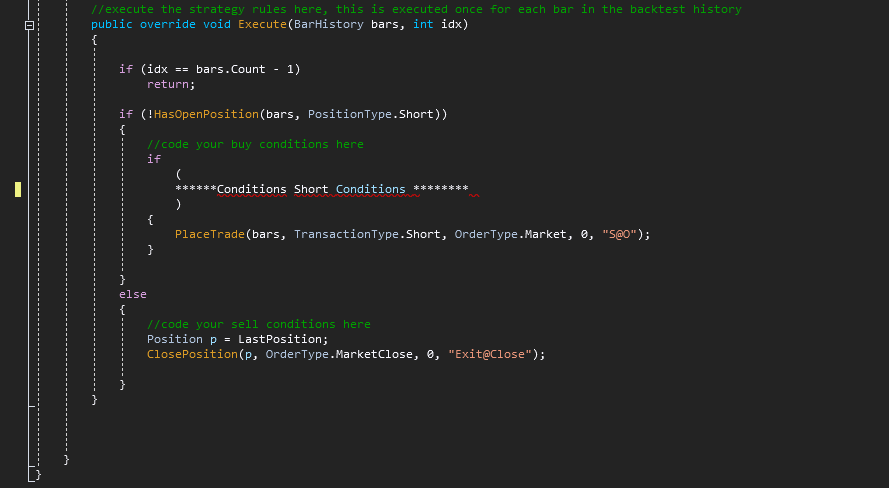
I don't want a closing condition. I want the trade to CLOSE on the Close of the bar that I Opened it in. Not subject to conditions.
I think I gave you enough to go on, if you want a lot more custom coding we offer a Concierge Support service where we can code your complete strategy based on your specs.
Here’s a hint though, you just need to place your exit trade at close on the line right after your entry.
Here’s a hint though, you just need to place your exit trade at close on the line right after your entry.
Your code does not close on the date of entry.
But that is ok to go on.
Ok...
Thanks for your effort.
But that is ok to go on.
Ok...
Thanks for your effort.
For all of you that want to trade on same bar, don't put anything on the else side of the HasOpenPosition
CODE:
{ PlaceTrade(bars, TransactionType.Short, OrderType.Market, 0, "S@O"); } { PlaceTrade(bars, TransactionType.Cover, OrderType.MarketClose, 0, "Having a Glitch is your code is Cool"); }
>> Your code does not close on the date of entry.<<
Umm, take a look at the chart image I posted and the area surrounded in red. It most certainly does exit on the date of entry 🤷🏼♂️
Umm, take a look at the chart image I posted and the area surrounded in red. It most certainly does exit on the date of entry 🤷🏼♂️
Your Response
Post
Edit Post
Login is required