The new Option Chain feature in Tradier extension B24 is FANTASTIC!!!!!
I whipped up sample code (included at end of post) that shows the power of this new capability for trading options with WL. This demo selects a Call option for an underlying with a monthly expiration at least 31 days out. It requires that the Greek Delta be between .4 and .6 (close to the money) and that the price of the option does not exceed its allocation. It then selects from candidate options that meet these criteria the one that maximizes transaction value. These are just some examples of the types of complex rules you can apply to option selection, using the power of Linc statements in C#.
The sample code replaces 336 lines of code in my working strategy and eliminates 90+ % of calls made to the broker for option data!
I did come across a couple of glitches, which could be related to each other:
1. There is some strange caching going on either in WL or at Tradier that keeps populating an option chain fetch with results from previous fetches on other symbols.
2. Option chain requests return stale chain data from Tradier as time goes on, after the initial fetch that followed a WL restart.
Caching
A snap of the debug log on a three-symbol dataset shows how, after clearing the Option List, the next symbol fetch returns data from previous symbol fetches and includes it with the new.
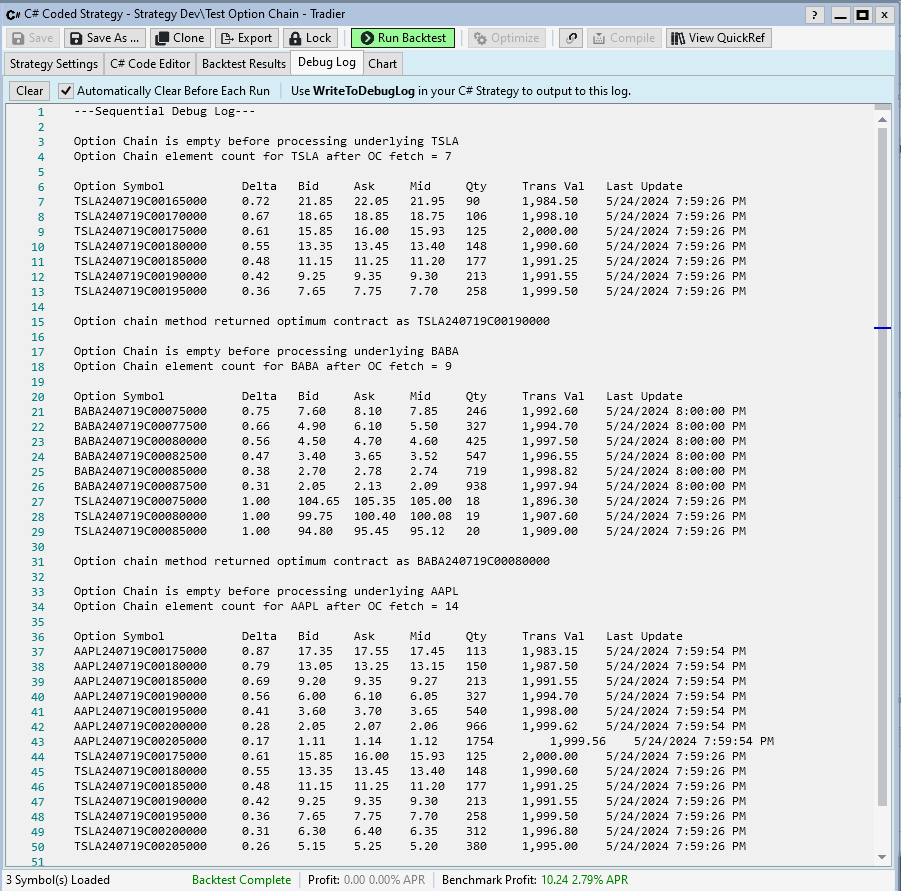
Stale Chain Data
Here's a thread I had with Tradier tech support:
Sample Code
I whipped up sample code (included at end of post) that shows the power of this new capability for trading options with WL. This demo selects a Call option for an underlying with a monthly expiration at least 31 days out. It requires that the Greek Delta be between .4 and .6 (close to the money) and that the price of the option does not exceed its allocation. It then selects from candidate options that meet these criteria the one that maximizes transaction value. These are just some examples of the types of complex rules you can apply to option selection, using the power of Linc statements in C#.
The sample code replaces 336 lines of code in my working strategy and eliminates 90+ % of calls made to the broker for option data!
I did come across a couple of glitches, which could be related to each other:
1. There is some strange caching going on either in WL or at Tradier that keeps populating an option chain fetch with results from previous fetches on other symbols.
2. Option chain requests return stale chain data from Tradier as time goes on, after the initial fetch that followed a WL restart.
Caching
A snap of the debug log on a three-symbol dataset shows how, after clearing the Option List, the next symbol fetch returns data from previous symbol fetches and includes it with the new.
Stale Chain Data
Here's a thread I had with Tradier tech support:
QUOTE:
Tech. replied
May 23, 1:29pm
I just pulled the chain to confirm these details and here is what I got from our data provider:
https://documentation.tradier.com/brokerage-api/markets/get-options-chains
{'symbol': 'PLUG240719C00003000', 'description': 'PLUG Jul 19 2024 $3.00 Call', 'exch': 'Z', 'type': 'option', 'last': 0.53, 'change': -0.13, 'volume': 38, 'open': 0.59, 'high': 0.59, 'low': 0.52, 'close': None, 'bid': 0.5, 'ask': 0.54, 'underlying': 'PLUG', 'strike': 3.0, 'greeks': {'delta': 0.6479942833699219, 'gamma': 0.3366493682351791, 'theta': -0.0035140679977920266, 'vega': 0.004686660601744747, 'rho': 0.0021265099103928813, 'phi': -0.0027956345185813397, 'bid_iv': 0.830937, 'mid_iv': 0.841645, 'ask_iv': 0.852352, 'smv_vol': 0.857, 'updated_at': '2024-05-23 16:59:06'}, 'change_percentage': -19.7, 'average_volume': 0, 'last_volume': 3, 'trade_date': 1716483729345, 'prevclose': 0.66, 'week_52_high': 0.0, 'week_52_low': 0.0, 'bidsize': 4141, 'bidexch': 'D', 'bid_date': 1716484690000, 'asksize': 152, 'askexch': 'D', 'ask_date': 1716484688000, 'open_interest': 416, 'contract_size': 100, 'expiration_date': '2024-07-19', 'expiration_type': 'standard', 'option_type': 'call', 'root_symbol': 'PLUG'}
It shows the update as being in the last hour (time is in UTC so 16:59 is about 13:00 EDT). Sometimes when volume is very very low on particular options they can go without an update for a bit but in this case, it looks like it has been updated.
All the best,
Tradier Support
techsupport@tradier.com
© Tradier Inc. All rights reserved.
Tradier Brokerage Inc. Member FINRA/SIPC
Original Sent message
May 23, 12:57pm
Hello.
Using the API to get an option chain for symbol plug at 12:50 Eastern time today 5/23 returns the following:
PLUG240719C00003000 0.71 0.55 0.59 0.57 5/22/2024 7:59:50 PM
This is a $3.00 strike call option with a delta of 71. The other numbers are (in order) bid, ask, midpoint, and last updated. Note last updated is from 7:59 PM yesterday (not sure what time zone that is). I understand that option chains for the API are only updated once an hour at Tradier, but why not since yesterday? The information is so out-of-date as to be useless.
Is there a way to get real-time bid, ask, midpoint, or last price?
Sample Code
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; using System.Linq; using WealthLab.Tradier; using System.Text; using System.Runtime.InteropServices; using WealthLab.ChartWPF; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { public override void BacktestBegin() { _minDelta = .4; _maxDelta = .6; _minDaysToExpire = 31; } //create indicators and other objects here, this is executed prior to the main trading loop public override void Initialize(BarHistory bars) { _indexSym = bars.Symbol; _optAllocAmt = 2000; } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { bool lastBar = idx == bars.Count - 1; if (lastBar) { _contractSymbol = GetContractSymbolFromChain(_optAllocAmt, _minDelta, _maxDelta, _minDaysToExpire, bars.Symbol, bars.Close[idx]); if (_contractSymbol != null && _contractSymbol != "") { WriteToDebugLog("\nOption chain method returned optimum contract as " + _contractSymbol, false); } else WriteToDebugLog(bars.DateTimes[idx].ToShortDateString() + " " + _indexSym + " did not return valid contract that meets all criteria"); string GetContractSymbolFromChain(double maxTransAmt, double deltaMin, double deltaMax, int expDays, string uSym, double uPrc) { double lStrike = uPrc * .9; double hStrike = uPrc * 1.1; string optimumOptionSymbol = ""; OptionGreek optimumOption = null; List<OptionGreek> optChain = new List<OptionGreek>(); DateTime expDate = bars.DateTimes[idx].AddDays(_minDaysToExpire).NextOptionExpiryDate(bars); if(optChain.Any()) WriteToDebugLog("\nOption Chain has data for underlying " + uSym, false); else WriteToDebugLog("\nOption Chain is empty before processing underlying " + uSym, false); optChain = TradierHistorical.Instance.GetOptionChainSnapshot(uSym, expDate, OptionType.Call, lStrike, hStrike, 10); if (optChain.Any()) { WriteToDebugLog("Option Chain element count for " + uSym + " after OC fetch = " + optChain.Count(), false); WriteToDebugLog("\nOption Symbol\t\t\tDelta\tBid\t\tAsk\t\tMid\t\tQty\t\tTrans Val\tLast Update", false); foreach (OptionGreek g in optChain) { WriteToDebugLog(g.Symbol + "\t\t" + g.Delta.ToString("N2") + "\t" + g.Bid.ToString("N2") + "\t" + g.Ask.ToString("N2") + "\t" + g.Midpoint.ToString("N2") + "\t" + (int)(maxTransAmt / g.Ask) + "\t\t" + ((int)(maxTransAmt / g.Ask) * g.Ask).ToString("N2") + "\t" + g.UpdatedAt, false); } } else WriteToDebugLog(uSym + " Option Chain is empty", false); optimumOption = optChain.Where(o => OptionsHelper.SymbolUnderlier(o.Symbol) == uSym && o.Delta >= deltaMin && o.Delta <= deltaMax && o.Ask * 100 < maxTransAmt) //must be <= than allocation .OrderByDescending(t => (int)(maxTransAmt / (t.Ask * 100)) * t.Ask) //sort hi-lo by price * qty .FirstOrDefault(); //select first (highest transaction value within allocation amt) if (optimumOption != null) { optimumOptionSymbol = optimumOption.Symbol; } else WriteToDebugLog("\nQualifying contract not found", false); return optimumOptionSymbol; } } } //declare private variables below private static int _minDaysToExpire; private static double _minDelta; private static double _maxDelta; private string _indexSym; private string _contractSymbol; private double _optAllocAmt; } }
Rename
CODE:Yes, thank you. I think I see the problem and will fix!
I did come across a couple of glitches, which could be related to each other:
A 1-liner fix. Too bad I missed that detail the first time through, but thanks for reporting it.
Here's a run on the Dow 30 after the fix for Build 26.
Here's a run on the Dow 30 after the fix for Build 26.
CODE:
---Sequential Debug Log--- Option Chain is empty before processing underlying DOW Option Chain element count for DOW after OC fetch = 5 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update DOW240719C00052500 0.93 5.20 6.30 5.75 317 1,997.10 5/24/2024 7:59:58 PM DOW240719C00055000 0.76 2.90 3.20 3.05 625 2,000.00 5/24/2024 7:59:58 PM DOW240719C00057500 0.52 1.43 1.54 1.48 1298 1,998.92 5/24/2024 7:59:58 PM DOW240719C00060000 0.26 0.54 0.59 0.56 3389 1,999.51 5/24/2024 7:59:58 PM DOW240719C00062500 0.10 0.14 0.17 0.16 11764 1,999.88 5/24/2024 7:59:58 PM Option chain method returned optimum contract as DOW240719C00057500 Option Chain is empty before processing underlying UNH Option Chain element count for UNH after OC fetch = 10 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update UNH240719C00460000 0.88 52.30 53.55 52.92 37 1,981.35 5/24/2024 7:59:49 PM UNH240719C00470000 0.83 43.70 44.80 44.25 44 1,971.20 5/24/2024 7:59:49 PM UNH240719C00480000 0.77 35.55 36.80 36.17 54 1,987.20 5/24/2024 7:59:49 PM UNH240719C00490000 0.70 28.25 29.80 29.02 67 1,996.60 5/24/2024 7:59:49 PM UNH240719C00500000 0.61 22.25 22.65 22.45 88 1,993.20 5/24/2024 7:59:49 PM UNH240719C00510000 0.52 16.65 17.00 16.82 117 1,989.00 5/24/2024 7:59:49 PM UNH240719C00520000 0.42 12.10 12.35 12.22 161 1,988.35 5/24/2024 7:59:49 PM UNH240719C00530000 0.33 8.45 9.10 8.77 219 1,992.90 5/24/2024 7:59:49 PM UNH240719C00540000 0.25 5.65 6.40 6.03 312 1,996.80 5/24/2024 7:59:49 PM UNH240719C00550000 0.19 3.75 4.35 4.05 459 1,996.65 5/24/2024 7:59:49 PM Option chain method returned optimum contract as UNH240719C00510000 Option Chain is empty before processing underlying V Option Chain element count for V after OC fetch = 11 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update V240719C00250000 0.92 26.80 27.60 27.20 72 1,987.20 5/24/2024 8:00:00 PM V240719C00255000 0.89 21.90 23.15 22.52 86 1,990.90 5/24/2024 8:00:00 PM V240719C00260000 0.84 17.80 18.45 18.12 108 1,992.60 5/24/2024 8:00:00 PM V240719C00265000 0.77 13.65 14.15 13.90 141 1,995.15 5/24/2024 8:00:00 PM V240719C00270000 0.68 10.05 10.35 10.20 193 1,997.55 5/24/2024 8:00:00 PM V240719C00275000 0.56 6.95 7.05 7.00 283 1,995.15 5/24/2024 8:00:00 PM V240719C00280000 0.42 4.45 4.60 4.53 434 1,996.40 5/24/2024 8:00:00 PM V240719C00285000 0.30 2.64 2.75 2.70 727 1,999.25 5/24/2024 8:00:00 PM V240719C00290000 0.19 1.49 1.56 1.52 1282 1,999.92 5/24/2024 8:00:00 PM V240719C00295000 0.12 0.70 0.86 0.78 2325 1,999.50 5/24/2024 8:00:00 PM V240719C00300000 0.07 0.35 0.59 0.47 3389 1,999.51 5/24/2024 8:00:00 PM Option chain method returned optimum contract as V240719C00280000 Option Chain is empty before processing underlying AMGN Option Chain element count for AMGN after OC fetch = 12 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update AMGN240719C00280000 0.89 28.90 30.05 29.48 66 1,983.30 5/24/2024 7:59:52 PM AMGN240719C00285000 0.85 24.55 25.50 25.02 78 1,989.00 5/24/2024 7:59:52 PM AMGN240719C00290000 0.79 20.85 21.40 21.12 93 1,990.20 5/24/2024 7:59:52 PM AMGN240719C00295000 0.73 17.05 19.10 18.08 104 1,986.40 5/24/2024 7:59:52 PM AMGN240719C00300000 0.66 13.70 13.95 13.82 143 1,994.85 5/24/2024 7:59:52 PM AMGN240719C00305000 0.57 10.65 10.90 10.78 183 1,994.70 5/24/2024 7:59:52 PM AMGN240719C00310000 0.49 8.05 8.30 8.18 240 1,992.00 5/24/2024 7:59:52 PM AMGN240719C00315000 0.40 5.95 6.15 6.05 325 1,998.75 5/24/2024 7:59:52 PM AMGN240719C00320000 0.32 4.25 4.45 4.35 449 1,998.05 5/24/2024 7:59:52 PM AMGN240719C00325000 0.25 2.98 3.15 3.06 634 1,997.10 5/24/2024 7:59:52 PM AMGN240719C00330000 0.19 2.07 2.19 2.13 913 1,999.47 5/24/2024 7:59:52 PM AMGN240719C00335000 0.14 1.41 1.53 1.47 1307 1,999.71 5/24/2024 7:59:52 PM Option chain method returned optimum contract as AMGN240719C00310000 Option Chain is empty before processing underlying HON Option Chain element count for HON after OC fetch = 6 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update HON240719C00180000 0.93 21.10 22.00 21.55 90 1,980.00 5/24/2024 8:00:04 PM HON240719C00185000 0.88 16.70 18.10 17.40 110 1,991.00 5/24/2024 8:00:04 PM HON240719C00190000 0.81 12.30 12.70 12.50 157 1,993.90 5/24/2024 8:00:04 PM HON240719C00195000 0.70 8.40 8.70 8.55 229 1,992.30 5/24/2024 8:00:04 PM HON240719C00200000 0.56 5.10 5.30 5.20 377 1,998.10 5/24/2024 8:00:04 PM HON240719C00210000 0.21 1.15 1.30 1.23 1538 1,999.40 5/24/2024 8:00:04 PM Option chain method returned optimum contract as HON240719C00200000 Option Chain is empty before processing underlying BA Option Chain element count for BA after OC fetch = 7 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update BA240719C00160000 0.79 18.55 19.25 18.90 103 1,982.75 5/24/2024 8:00:01 PM BA240719C00165000 0.72 14.70 15.55 15.12 128 1,990.40 5/24/2024 8:00:01 PM BA240719C00170000 0.64 11.60 11.90 11.75 168 1,999.20 5/24/2024 8:00:01 PM BA240719C00175000 0.55 8.85 9.00 8.93 222 1,998.00 5/24/2024 8:00:01 PM BA240719C00180000 0.45 6.55 6.75 6.65 296 1,998.00 5/24/2024 8:00:01 PM BA240719C00185000 0.36 4.70 4.85 4.78 412 1,998.20 5/24/2024 8:00:01 PM BA240719C00190000 0.28 3.30 3.45 3.38 579 1,997.55 5/24/2024 8:00:01 PM Option chain method returned optimum contract as BA240719C00175000 Option Chain is empty before processing underlying CRM Option Chain element count for CRM after OC fetch = 5 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update CRM240719C00250000 0.78 28.25 28.75 28.50 69 1,983.75 5/24/2024 8:00:00 PM CRM240719C00260000 0.69 21.35 21.60 21.48 92 1,987.20 5/24/2024 8:00:00 PM CRM240719C00270000 0.57 15.40 15.65 15.53 127 1,987.55 5/24/2024 8:00:00 PM CRM240719C00280000 0.45 10.65 10.85 10.75 184 1,996.40 5/24/2024 8:00:00 PM CRM240719C00290000 0.34 7.00 7.20 7.10 277 1,994.40 5/24/2024 8:00:00 PM Option chain method returned optimum contract as CRM240719C00270000 Option Chain is empty before processing underlying VZ Option Chain element count for VZ after OC fetch = 8 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update VZ240719C00036000 0.92 3.20 4.20 3.70 476 1,999.20 5/24/2024 7:59:55 PM VZ240719C00037000 0.84 2.98 3.15 3.06 634 1,997.10 5/24/2024 7:59:55 PM VZ240719C00038000 0.73 1.99 2.42 2.21 826 1,998.92 5/24/2024 7:59:55 PM VZ240719C00039000 0.60 1.45 1.49 1.47 1342 1,999.58 5/24/2024 7:59:55 PM VZ240719C00040000 0.46 0.85 0.89 0.87 2247 1,999.83 5/24/2024 7:59:55 PM VZ240719C00041000 0.31 0.45 0.46 0.46 4347 1,999.62 5/24/2024 7:59:55 PM VZ240719C00042000 0.19 0.20 0.25 0.23 8000 2,000.00 5/24/2024 7:59:55 PM VZ240719C00043000 0.10 0.09 0.12 0.10 16666 1,999.92 5/24/2024 7:59:55 PM Option chain method returned optimum contract as VZ240719C00040000 Option Chain is empty before processing underlying TRV Option Chain element count for TRV after OC fetch = 5 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update TRV240719C00195000 0.87 18.60 20.20 19.40 99 1,999.80 5/24/2024 8:00:04 PM TRV240719C00200000 0.81 15.30 15.80 15.55 126 1,990.80 5/24/2024 8:00:04 PM TRV240719C00210000 0.61 8.00 8.40 8.20 238 1,999.20 5/24/2024 8:00:04 PM TRV240719C00220000 0.35 3.10 3.40 3.25 588 1,999.20 5/24/2024 8:00:04 PM TRV240719C00230000 0.14 0.90 1.10 1.00 1818 1,999.80 5/24/2024 8:00:04 PM Qualifying contract not found Option Chain is empty before processing underlying GS Option Chain element count for GS after OC fetch = 18 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update GS240719C00420000 0.87 43.85 46.10 44.98 43 1,982.30 5/24/2024 7:59:53 PM GS240719C00425000 0.84 40.05 41.20 40.62 48 1,977.60 5/24/2024 7:59:53 PM GS240719C00430000 0.81 35.55 36.85 36.20 54 1,989.90 5/24/2024 7:59:53 PM GS240719C00435000 0.77 32.00 32.90 32.45 60 1,974.00 5/24/2024 7:59:53 PM GS240719C00440000 0.73 27.85 29.30 28.58 68 1,992.40 5/24/2024 7:59:53 PM GS240719C00445000 0.69 24.65 26.00 25.32 76 1,976.00 5/24/2024 7:59:53 PM GS240719C00450000 0.64 21.25 22.10 21.68 90 1,989.00 5/24/2024 7:59:53 PM GS240719C00455000 0.59 18.65 19.20 18.92 104 1,996.80 5/24/2024 7:59:53 PM GS240719C00460000 0.54 15.95 16.25 16.10 123 1,998.75 5/24/2024 7:59:53 PM GS240719C00465000 0.48 13.40 13.80 13.60 144 1,987.20 5/24/2024 7:59:53 PM GS240719C00470000 0.43 11.20 11.45 11.32 174 1,992.30 5/24/2024 7:59:53 PM GS240719C00475000 0.38 9.20 9.60 9.40 208 1,996.80 5/24/2024 7:59:53 PM GS240719C00480000 0.33 7.45 7.80 7.62 256 1,996.80 5/24/2024 7:59:53 PM GS240719C00485000 0.28 6.05 6.30 6.17 317 1,997.10 5/24/2024 7:59:53 PM GS240719C00490000 0.24 4.85 5.10 4.97 392 1,999.20 5/24/2024 7:59:53 PM GS240719C00495000 0.20 3.85 4.15 4.00 481 1,996.15 5/24/2024 7:59:53 PM GS240719C00500000 0.17 3.05 3.25 3.15 615 1,998.75 5/24/2024 7:59:53 PM GS240719C00505000 0.14 2.39 2.57 2.48 778 1,999.46 5/24/2024 7:59:53 PM Option chain method returned optimum contract as GS240719C00455000 Option Chain is empty before processing underlying AAPL Option Chain element count for AAPL after OC fetch = 7 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update AAPL240719C00175000 0.87 17.35 17.55 17.45 113 1,983.15 5/24/2024 7:59:54 PM AAPL240719C00180000 0.79 13.05 13.25 13.15 150 1,987.50 5/24/2024 7:59:54 PM AAPL240719C00185000 0.69 9.20 9.35 9.27 213 1,991.55 5/24/2024 7:59:54 PM AAPL240719C00190000 0.56 6.00 6.10 6.05 327 1,994.70 5/24/2024 7:59:54 PM AAPL240719C00195000 0.41 3.60 3.70 3.65 540 1,998.00 5/24/2024 7:59:54 PM AAPL240719C00200000 0.28 2.05 2.07 2.06 966 1,999.62 5/24/2024 7:59:54 PM AAPL240719C00205000 0.17 1.11 1.14 1.12 1754 1,999.56 5/24/2024 7:59:54 PM Option chain method returned optimum contract as AAPL240719C00195000 Option Chain is empty before processing underlying HD Option Chain element count for HD after OC fetch = 13 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update HD240719C00295000 0.88 31.25 33.50 32.38 59 1,976.50 5/24/2024 8:00:01 PM HD240719C00300000 0.85 26.80 27.55 27.18 72 1,983.60 5/24/2024 8:00:01 PM HD240719C00305000 0.80 22.55 24.20 23.38 82 1,984.40 5/24/2024 8:00:01 PM HD240719C00310000 0.74 18.65 19.65 19.15 101 1,984.65 5/24/2024 8:00:01 PM HD240719C00315000 0.68 15.10 17.30 16.20 115 1,989.50 5/24/2024 8:00:01 PM HD240719C00320000 0.60 11.75 12.45 12.10 160 1,992.00 5/24/2024 8:00:01 PM HD240719C00325000 0.52 8.85 9.20 9.02 217 1,996.40 5/24/2024 8:00:01 PM HD240719C00330000 0.43 6.50 6.75 6.62 296 1,998.00 5/24/2024 8:00:01 PM HD240719C00335000 0.34 4.65 4.80 4.72 416 1,996.80 5/24/2024 8:00:01 PM HD240719C00340000 0.25 3.20 3.45 3.33 579 1,997.55 5/24/2024 8:00:01 PM HD240719C00345000 0.18 2.17 2.37 2.27 843 1,997.91 5/24/2024 8:00:01 PM HD240719C00350000 0.13 1.46 1.67 1.56 1197 1,998.99 5/24/2024 8:00:01 PM HD240719C00355000 0.10 0.86 1.16 1.01 1724 1,999.84 5/24/2024 8:00:01 PM Option chain method returned optimum contract as HD240719C00325000 Option Chain is empty before processing underlying WBA Option Chain element count for WBA after OC fetch = 2 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update WBA240719C00015000 0.69 1.74 1.79 1.77 1117 1,999.43 5/24/2024 7:59:53 PM WBA240719C00017500 0.35 0.60 0.61 0.60 3278 1,999.58 5/24/2024 7:59:53 PM Qualifying contract not found Option Chain is empty before processing underlying CAT Option Chain element count for CAT after OC fetch = 7 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update CAT240719C00320000 0.85 33.20 34.75 33.98 57 1,980.75 5/24/2024 7:59:56 PM CAT240719C00330000 0.76 25.15 27.65 26.40 72 1,990.80 5/24/2024 7:59:56 PM CAT240719C00340000 0.66 18.45 19.00 18.73 105 1,995.00 5/24/2024 7:59:56 PM CAT240719C00350000 0.53 12.55 12.95 12.75 154 1,994.30 5/24/2024 7:59:56 PM CAT240719C00360000 0.40 7.95 8.30 8.12 240 1,992.00 5/24/2024 7:59:56 PM CAT240719C00370000 0.28 4.70 5.15 4.93 388 1,998.20 5/24/2024 7:59:56 PM CAT240719C00380000 0.18 2.70 3.45 3.08 579 1,997.55 5/24/2024 7:59:56 PM Option chain method returned optimum contract as CAT240719C00360000 Option Chain is empty before processing underlying IBM Option Chain element count for IBM after OC fetch = 7 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update IBM240719C00155000 0.89 17.40 18.10 17.75 110 1,991.00 5/24/2024 7:59:51 PM IBM240719C00160000 0.84 12.80 13.25 13.03 150 1,987.50 5/24/2024 7:59:51 PM IBM240719C00165000 0.75 8.80 9.05 8.93 220 1,991.00 5/24/2024 7:59:51 PM IBM240719C00170000 0.60 5.50 5.60 5.55 357 1,999.20 5/24/2024 7:59:51 PM IBM240719C00175000 0.41 3.05 3.15 3.10 634 1,997.10 5/24/2024 7:59:51 PM IBM240719C00180000 0.25 1.51 1.62 1.56 1234 1,999.08 5/24/2024 7:59:51 PM IBM240719C00185000 0.15 0.64 0.83 0.73 2409 1,999.47 5/24/2024 7:59:51 PM Option chain method returned optimum contract as IBM240719C00175000 Option Chain is empty before processing underlying AXP Option Chain element count for AXP after OC fetch = 5 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update AXP240719C00220000 0.83 20.55 21.55 21.05 92 1,982.60 5/24/2024 7:59:51 PM AXP240719C00230000 0.70 13.55 13.75 13.65 145 1,993.75 5/24/2024 7:59:51 PM AXP240719C00240000 0.51 7.60 7.80 7.70 256 1,996.80 5/24/2024 7:59:51 PM AXP240719C00250000 0.32 3.70 3.85 3.78 519 1,998.15 5/24/2024 7:59:51 PM AXP240719C00260000 0.17 1.60 1.77 1.69 1129 1,998.33 5/24/2024 7:59:51 PM Option chain method returned optimum contract as AXP240719C00240000 Option Chain is empty before processing underlying CVX Option Chain element count for CVX after OC fetch = 6 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update CVX240719C00145000 0.89 14.45 14.80 14.62 135 1,998.00 5/24/2024 7:59:53 PM CVX240719C00150000 0.80 10.20 10.35 10.27 193 1,997.55 5/24/2024 7:59:53 PM CVX240719C00155000 0.66 6.50 6.65 6.58 300 1,995.00 5/24/2024 7:59:53 PM CVX240719C00160000 0.48 3.65 3.70 3.67 540 1,998.00 5/24/2024 7:59:53 PM CVX240719C00165000 0.29 1.80 1.84 1.82 1086 1,998.24 5/24/2024 7:59:53 PM CVX240719C00170000 0.16 0.79 0.82 0.80 2439 1,999.98 5/24/2024 7:59:53 PM Option chain method returned optimum contract as CVX240719C00160000 Option Chain is empty before processing underlying JNJ Option Chain element count for JNJ after OC fetch = 6 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update JNJ240719C00135000 0.89 13.20 14.25 13.72 140 1,995.00 5/24/2024 7:59:59 PM JNJ240719C00140000 0.80 9.05 9.30 9.18 215 1,999.50 5/24/2024 7:59:59 PM JNJ240719C00145000 0.66 5.30 5.45 5.38 366 1,994.70 5/24/2024 7:59:59 PM JNJ240719C00150000 0.43 2.57 2.65 2.61 754 1,998.10 5/24/2024 7:59:59 PM JNJ240719C00155000 0.22 1.02 1.11 1.06 1801 1,999.11 5/24/2024 7:59:59 PM JNJ240719C00160000 0.11 0.36 0.49 0.42 4081 1,999.69 5/24/2024 7:59:59 PM Option chain method returned optimum contract as JNJ240719C00150000 Option Chain is empty before processing underlying CSCO Option Chain element count for CSCO after OC fetch = 4 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update CSCO240719C00042500 0.90 4.20 4.50 4.35 444 1,998.00 5/24/2024 8:00:07 PM CSCO240719C00045000 0.71 2.05 2.14 2.09 934 1,998.76 5/24/2024 8:00:07 PM CSCO240719C00047500 0.35 0.71 0.73 0.72 2739 1,999.47 5/24/2024 8:00:07 PM CSCO240719C00050000 0.11 0.17 0.19 0.18 10526 1,999.94 5/24/2024 8:00:07 PM Qualifying contract not found Option Chain is empty before processing underlying MSFT Option Chain element count for MSFT after OC fetch = 17 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update MSFT240719C00390000 0.90 44.45 45.25 44.85 44 1,991.00 5/24/2024 7:59:54 PM MSFT240719C00395000 0.87 40.15 40.90 40.52 48 1,963.20 5/24/2024 7:59:54 PM MSFT240719C00400000 0.85 35.70 36.45 36.08 54 1,968.30 5/24/2024 7:59:54 PM MSFT240719C00405000 0.81 31.35 32.00 31.68 62 1,984.00 5/24/2024 7:59:54 PM MSFT240719C00410000 0.78 27.30 27.90 27.60 71 1,980.90 5/24/2024 7:59:54 PM MSFT240719C00415000 0.74 23.40 23.95 23.67 83 1,987.85 5/24/2024 7:59:54 PM MSFT240719C00420000 0.69 18.85 20.45 19.65 97 1,983.65 5/24/2024 7:59:54 PM MSFT240719C00425000 0.63 16.60 16.85 16.73 118 1,988.30 5/24/2024 7:59:54 PM MSFT240719C00430000 0.57 13.55 13.80 13.68 144 1,987.20 5/24/2024 7:59:54 PM MSFT240719C00435000 0.50 10.90 11.10 11.00 180 1,998.00 5/24/2024 7:59:54 PM MSFT240719C00440000 0.43 8.60 8.85 8.72 225 1,991.25 5/24/2024 7:59:54 PM MSFT240719C00445000 0.36 6.70 6.90 6.80 289 1,994.10 5/24/2024 7:59:54 PM MSFT240719C00450000 0.29 5.10 5.30 5.20 377 1,998.10 5/24/2024 7:59:54 PM MSFT240719C00455000 0.24 3.80 4.00 3.90 500 2,000.00 5/24/2024 7:59:54 PM MSFT240719C00460000 0.19 2.60 2.94 2.77 680 1,999.20 5/24/2024 7:59:54 PM MSFT240719C00465000 0.14 1.82 2.37 2.10 843 1,997.91 5/24/2024 7:59:54 PM MSFT240719C00470000 0.11 1.47 1.53 1.50 1307 1,999.71 5/24/2024 7:59:54 PM Option chain method returned optimum contract as MSFT240719C00440000 Option Chain is empty before processing underlying NKE Option Chain element count for NKE after OC fetch = 7 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update NKE240719C00085000 0.77 8.60 8.75 8.68 228 1,995.00 5/24/2024 8:00:03 PM NKE240719C00087500 0.69 6.90 7.00 6.95 285 1,995.00 5/24/2024 8:00:03 PM NKE240719C00090000 0.60 5.35 5.50 5.42 363 1,996.50 5/24/2024 8:00:03 PM NKE240719C00092500 0.51 4.05 4.15 4.10 481 1,996.15 5/24/2024 8:00:03 PM NKE240719C00095000 0.42 3.00 3.05 3.02 655 1,997.75 5/24/2024 8:00:03 PM NKE240719C00097500 0.33 2.11 2.21 2.16 904 1,997.84 5/24/2024 8:00:03 PM NKE240719C00100000 0.25 1.46 1.55 1.50 1290 1,999.50 5/24/2024 8:00:03 PM Option chain method returned optimum contract as NKE240719C00095000 Option Chain is empty before processing underlying MCD Option Chain element count for MCD after OC fetch = 10 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update MCD240719C00235000 0.89 22.85 24.60 23.73 81 1,992.60 5/24/2024 7:59:34 PM MCD240719C00240000 0.85 19.20 20.40 19.80 98 1,999.20 5/24/2024 7:59:34 PM MCD240719C00245000 0.80 14.85 16.05 15.45 124 1,990.20 5/24/2024 7:59:34 PM MCD240719C00250000 0.72 11.00 11.50 11.25 173 1,989.50 5/24/2024 7:59:34 PM MCD240719C00255000 0.60 7.65 7.85 7.75 254 1,993.90 5/24/2024 7:59:34 PM MCD240719C00260000 0.46 4.95 5.10 5.03 392 1,999.20 5/24/2024 7:59:34 PM MCD240719C00265000 0.32 2.96 3.10 3.03 645 1,999.50 5/24/2024 7:59:34 PM MCD240719C00270000 0.21 1.69 1.89 1.79 1058 1,999.62 5/24/2024 7:59:34 PM MCD240719C00275000 0.14 0.89 1.00 0.95 2000 2,000.00 5/24/2024 7:59:34 PM MCD240719C00280000 0.09 0.52 0.60 0.56 3333 1,999.80 5/24/2024 7:59:34 PM Option chain method returned optimum contract as MCD240719C00260000 Option Chain is empty before processing underlying DIS Option Chain element count for DIS after OC fetch = 4 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update DIS240719C00095000 0.83 7.90 8.00 7.95 250 2,000.00 5/24/2024 7:59:58 PM DIS240719C00100000 0.63 4.15 4.25 4.20 470 1,997.50 5/24/2024 7:59:58 PM DIS240719C00105000 0.36 1.79 1.85 1.82 1081 1,999.85 5/24/2024 7:59:58 PM DIS240719C00110000 0.18 0.75 0.79 0.77 2531 1,999.49 5/24/2024 7:59:58 PM Qualifying contract not found Option Chain is empty before processing underlying INTC Option Chain element count for INTC after OC fetch = 6 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update INTC240719C00028000 0.81 3.35 3.45 3.40 579 1,997.55 5/24/2024 8:00:04 PM INTC240719C00029000 0.73 2.42 2.67 2.54 749 1,999.83 5/24/2024 8:00:04 PM INTC240719C00030000 0.63 1.99 2.03 2.01 985 1,999.55 5/24/2024 8:00:04 PM INTC240719C00031000 0.52 1.46 1.50 1.48 1333 1,999.50 5/24/2024 8:00:04 PM INTC240719C00032000 0.42 1.05 1.06 1.06 1886 1,999.16 5/24/2024 8:00:04 PM INTC240719C00033000 0.32 0.73 0.74 0.73 2702 1,999.48 5/24/2024 8:00:04 PM Option chain method returned optimum contract as INTC240719C00031000 Option Chain is empty before processing underlying JPM Option Chain element count for JPM after OC fetch = 8 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update JPM240719C00185000 0.84 17.60 18.20 17.90 109 1,983.80 5/24/2024 7:59:58 PM JPM240719C00190000 0.77 13.45 13.80 13.62 144 1,987.20 5/24/2024 7:59:58 PM JPM240719C00195000 0.67 9.75 9.90 9.82 202 1,999.80 5/24/2024 7:59:58 PM JPM240719C00200000 0.55 6.65 6.75 6.70 296 1,998.00 5/24/2024 7:59:58 PM JPM240719C00205000 0.42 4.20 4.35 4.28 459 1,996.65 5/24/2024 7:59:58 PM JPM240719C00210000 0.29 2.51 2.59 2.55 772 1,999.48 5/24/2024 7:59:58 PM JPM240719C00215000 0.19 1.38 1.48 1.43 1351 1,999.48 5/24/2024 7:59:58 PM JPM240719C00220000 0.11 0.70 0.98 0.84 2040 1,999.20 5/24/2024 7:59:58 PM Option chain method returned optimum contract as JPM240719C00205000 Option Chain is empty before processing underlying PG Option Chain element count for PG after OC fetch = 7 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update PG240719C00150000 0.94 16.15 18.40 17.27 108 1,987.20 5/24/2024 7:59:51 PM PG240719C00155000 0.89 11.60 12.20 11.90 163 1,988.60 5/24/2024 7:59:51 PM PG240719C00160000 0.78 7.20 7.35 7.28 272 1,999.20 5/24/2024 7:59:51 PM PG240719C00165000 0.57 3.55 3.65 3.60 547 1,996.55 5/24/2024 7:59:51 PM PG240719C00170000 0.29 1.22 1.29 1.25 1550 1,999.50 5/24/2024 7:59:51 PM PG240719C00175000 0.10 0.28 0.44 0.36 4545 1,999.80 5/24/2024 7:59:51 PM PG240719C00180000 0.02 0.06 0.12 0.09 16666 1,999.92 5/24/2024 7:59:51 PM Option chain method returned optimum contract as PG240719C00165000 Option Chain is empty before processing underlying MMM Option Chain element count for MMM after OC fetch = 4 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update MMM240719C00090000 0.91 10.50 11.35 10.93 176 1,997.60 5/24/2024 7:59:51 PM MMM240719C00095000 0.77 6.55 6.75 6.65 296 1,998.00 5/24/2024 7:59:51 PM MMM240719C00100000 0.54 3.25 3.40 3.33 588 1,999.20 5/24/2024 7:59:51 PM MMM240719C00105000 0.30 1.33 1.43 1.38 1398 1,999.14 5/24/2024 7:59:51 PM Option chain method returned optimum contract as MMM240719C00100000 Option Chain is empty before processing underlying WMT Option Chain element count for WMT after OC fetch = 6 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update WMT240719C00060000 0.90 5.95 6.30 6.12 317 1,997.10 5/24/2024 7:59:52 PM WMT240719C00061670 0.85 3.80 4.75 4.28 421 1,999.75 5/24/2024 7:59:52 PM WMT240719C00063330 0.77 3.00 3.10 3.05 645 1,999.50 5/24/2024 7:59:52 PM WMT240719C00065000 0.62 1.82 1.84 1.83 1086 1,998.24 5/24/2024 7:59:52 PM WMT240719C00066670 0.41 0.95 0.96 0.95 2083 1,999.68 5/24/2024 7:59:52 PM WMT240719C00070000 0.15 0.20 0.22 0.21 9090 1,999.80 5/24/2024 7:59:52 PM Option chain method returned optimum contract as WMT240719C00066670 Option Chain is empty before processing underlying MRK Option Chain element count for MRK after OC fetch = 5 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update MRK240719C00120000 0.84 10.25 10.65 10.45 187 1,991.55 5/24/2024 7:59:53 PM MRK240719C00125000 0.72 6.15 6.35 6.25 314 1,993.90 5/24/2024 7:59:53 PM MRK240719C00130000 0.49 3.05 3.20 3.12 625 2,000.00 5/24/2024 7:59:53 PM MRK240719C00135000 0.25 1.19 1.25 1.22 1600 2,000.00 5/24/2024 7:59:53 PM MRK240719C00140000 0.12 0.39 0.52 0.46 3846 1,999.92 5/24/2024 7:59:53 PM Option chain method returned optimum contract as MRK240719C00130000 Option Chain is empty before processing underlying KO Option Chain element count for KO after OC fetch = 5 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update KO240719C00057500 0.92 4.65 4.90 4.78 408 1,999.20 5/24/2024 8:00:03 PM KO240719C00060000 0.75 2.40 2.61 2.50 766 1,999.26 5/24/2024 8:00:03 PM KO240719C00062500 0.43 0.84 0.89 0.86 2247 1,999.83 5/24/2024 8:00:03 PM KO240719C00065000 0.13 0.15 0.18 0.16 11111 1,999.98 5/24/2024 8:00:03 PM KO240719C00067500 0.03 0.03 0.05 0.04 40000 2,000.00 5/24/2024 8:00:03 PM Option chain method returned optimum contract as KO240719C00062500 ---Symbol by Symbol Debug Logs--- ---TRV--- 5/24/2024 TRV did not return valid contract that meets all criteria ---WBA--- 5/24/2024 WBA did not return valid contract that meets all criteria ---CSCO--- 5/24/2024 CSCO did not return valid contract that meets all criteria ---DIS--- 5/24/2024 DIS did not return valid contract that meets all criteria
@Cone Nice! Do you think this had anything to do with not fetching the latest chain data? Is that fixed as well?
What other broker extensions does the Options Chain fetch work for?
(after modifying lines 8 and 58 of the excellent example code accordingly)
I tried using (without any yield):
8 using WealthLab.IQFeed;
58 optChain = IQFeedHistorical.Instance.GetOptionChainSnapshot(uSym, expDate, OptionType.Call, lStrike, hStrike, 10);
[unfortunately, I can't get a Tradier account]. Does it work for the Medved extension (with Schwab or Fidelity as data source)?
(after modifying lines 8 and 58 of the excellent example code accordingly)
I tried using (without any yield):
8 using WealthLab.IQFeed;
58 optChain = IQFeedHistorical.Instance.GetOptionChainSnapshot(uSym, expDate, OptionType.Call, lStrike, hStrike, 10);
[unfortunately, I can't get a Tradier account]. Does it work for the Medved extension (with Schwab or Fidelity as data source)?
Re: innertrader
I'm not sure about the latest data. As long as successive requests are 3 seconds apart, we'll request a refresh. I'll make sure the refresh is working next week.
I'll convert the UpdatedAt time from UTC to EST since we're generally supporting only U.S. options.
Re: bcdxxx
You can get the full OptionChain from IQFeed but they don't return greeks. For their apps, they calculate the greeks to display them. GetOptionChainSnapshot (used for a single expiration) is currently only available for Tradier, for IB with the next build (51), and probably Schwab when that happens. (We just added the function to the API, so Medved would have to implement it.)
I'm not sure about the latest data. As long as successive requests are 3 seconds apart, we'll request a refresh. I'll make sure the refresh is working next week.
I'll convert the UpdatedAt time from UTC to EST since we're generally supporting only U.S. options.
Re: bcdxxx
You can get the full OptionChain from IQFeed but they don't return greeks. For their apps, they calculate the greeks to display them. GetOptionChainSnapshot (used for a single expiration) is currently only available for Tradier, for IB with the next build (51), and probably Schwab when that happens. (We just added the function to the API, so Medved would have to implement it.)
QUOTE:Found the bug here too. We'll get these corrrections out later this week in Trader build 26.
As long as successive requests are 3 seconds apart, we'll request a refresh. I'll make sure the refresh is working next week.
fyi, (Tradier greeks)
Although this is fixed (in dev) from our side, it looks like the greeks don't really update until at least 10 minutes after the hour.
This request was performed about 12 minutes after the hour and still several symbols still returned greek data from the previous hour. It wasn't until about 18 minutes into the next hour that all symbols returned the "latest" data.
For the latest data, you'll be able to use IB the same way, but the same request take several seconds longer for every symbol. The IBHistorical method has to use subscribe to every symbol in the chain (within the strike contraints) and wait for a streaming update to get the greeks. There are tradeoffs for either provider.
Although this is fixed (in dev) from our side, it looks like the greeks don't really update until at least 10 minutes after the hour.
This request was performed about 12 minutes after the hour and still several symbols still returned greek data from the previous hour. It wasn't until about 18 minutes into the next hour that all symbols returned the "latest" data.
CODE:
---Sequential Debug Log--- Option Chain is empty before processing underlying DOW Option Chain element count for DOW after OC fetch = 5 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update DOW240719C00052500 0.91 4.50 4.95 4.72 404 1,999.80 5/29/2024 1:59:07 PM DOW240719C00055000 0.73 2.57 2.82 2.69 709 1,999.38 5/29/2024 1:59:07 PM DOW240719C00057500 0.47 1.25 1.29 1.27 1550 1,999.50 5/29/2024 1:59:07 PM DOW240719C00060000 0.21 0.42 0.45 0.43 4444 1,999.80 5/29/2024 1:59:07 PM DOW240719C00062500 0.08 0.12 0.13 0.12 15384 1,999.92 5/29/2024 1:59:07 PM Option chain method returned optimum contract as DOW240719C00057500 Option Chain is empty before processing underlying V Option Chain element count for V after OC fetch = 11 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update V240719C00245000 0.92 28.05 28.65 28.35 69 1,976.85 5/29/2024 12:59:05 PM V240719C00250000 0.89 23.40 23.75 23.57 84 1,995.00 5/29/2024 12:59:05 PM V240719C00255000 0.85 18.95 19.25 19.10 103 1,982.75 5/29/2024 12:59:05 PM V240719C00260000 0.78 14.75 15.05 14.90 132 1,986.60 5/29/2024 12:59:05 PM V240719C00265000 0.70 10.95 11.25 11.10 177 1,991.25 5/29/2024 12:59:05 PM V240719C00270000 0.58 7.70 7.90 7.80 253 1,998.70 5/29/2024 12:59:05 PM V240719C00275000 0.45 5.05 5.20 5.12 384 1,996.80 5/29/2024 12:59:05 PM V240719C00280000 0.32 3.05 3.25 3.15 615 1,998.75 5/29/2024 12:59:05 PM V240719C00285000 0.21 1.77 1.87 1.82 1069 1,999.03 5/29/2024 12:59:05 PM V240719C00290000 0.13 0.97 1.04 1.00 1923 1,999.92 5/29/2024 12:59:05 PM V240719C00295000 0.08 0.51 0.56 0.54 3571 1,999.76 5/29/2024 12:59:05 PM Option chain method returned optimum contract as V240719C00270000 Option Chain is empty before processing underlying JNJ Option Chain element count for JNJ after OC fetch = 6 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update JNJ240719C00130000 0.94 15.65 15.95 15.80 125 1,993.75 5/29/2024 12:58:52 PM JNJ240719C00135000 0.87 11.15 11.35 11.25 176 1,997.60 5/29/2024 12:58:52 PM JNJ240719C00140000 0.74 7.00 7.25 7.12 275 1,993.75 5/29/2024 12:58:52 PM JNJ240719C00145000 0.55 3.80 3.95 3.88 506 1,998.70 5/29/2024 12:58:52 PM JNJ240719C00150000 0.32 1.67 1.70 1.69 1176 1,999.20 5/29/2024 12:58:52 PM JNJ240719C00155000 0.15 0.58 0.64 0.61 3125 2,000.00 5/29/2024 12:58:52 PM Option chain method returned optimum contract as JNJ240719C00145000 Option Chain is empty before processing underlying HD Option Chain element count for HD after OC fetch = 13 Option Symbol Delta Bid Ask Mid Qty Trans Val Last Update HD240719C00300000 0.86 27.05 27.90 27.48 71 1,980.90 5/29/2024 1:58:52 PM HD240719C00305000 0.81 22.70 23.40 23.05 85 1,989.00 5/29/2024 1:58:52 PM HD240719C00310000 0.75 18.85 19.50 19.18 102 1,989.00 5/29/2024 1:58:52 PM HD240719C00315000 0.68 15.20 15.50 15.35 129 1,999.50 5/29/2024 1:58:52 PM HD240719C00320000 0.60 11.90 12.20 12.05 163 1,988.60 5/29/2024 1:58:52 PM HD240719C00325000 0.52 9.10 9.30 9.20 215 1,999.50 5/29/2024 1:58:52 PM HD240719C00330000 0.43 6.70 7.00 6.85 285 1,995.00 5/29/2024 1:58:52 PM HD240719C00335000 0.35 4.90 5.10 5.00 392 1,999.20 5/29/2024 1:58:52 PM HD240719C00340000 0.27 3.45 3.65 3.55 547 1,996.55 5/29/2024 1:58:52 PM HD240719C00345000 0.20 2.40 2.52 2.46 793 1,998.36 5/29/2024 1:58:52 PM HD240719C00350000 0.14 1.63 1.76 1.69 1136 1,999.36 5/29/2024 1:58:52 PM HD240719C00355000 0.11 1.13 1.23 1.18 1626 1,999.98 5/29/2024 1:58:52 PM HD240719C00360000 0.08 0.79 0.87 0.83 2298 1,999.26 5/29/2024 1:58:52 PM
For the latest data, you'll be able to use IB the same way, but the same request take several seconds longer for every symbol. The IBHistorical method has to use subscribe to every symbol in the chain (within the strike contraints) and wait for a streaming update to get the greeks. There are tradeoffs for either provider.
QUOTE:
it looks like the greeks don't really update until at least 10 minutes after the hour.
Yes, I noticed that too.
The issues raised in this post were tested and resolved in Tradier B27. Thank you.
Your Response
Post
Edit Post
Login is required