What is the correct syntax for using indicators as parameter in the following script:
When dragging the indicator on a chart the window looks like screenshot 1 (and "WGHT" pane on chart remains empty). What is the correct syntax that it works like screenshot 2?
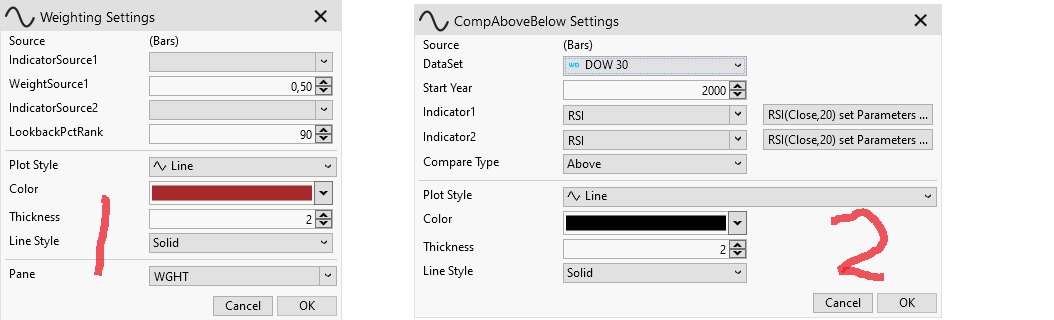
CODE:
using WealthLab.Core; using WealthLab.Indicators; namespace WealthLabCustom { public class Weighting : IndicatorBase { public Weighting() : base() { LibraryName = "RiMarBo"; } //for code based construction public Weighting(BarHistory barHistory, TimeSeries indicatorSource1, double weightSource1, TimeSeries indicatorSource2, int lookbackPctRank) : base() { LibraryName = "RiMarBo"; Parameters[0].Value = barHistory; Parameters[1].Value = indicatorSource1; Parameters[2].Value = weightSource1; Parameters[3].Value = indicatorSource2; Parameters[4].Value = lookbackPctRank; Populate(); } //abbreviation - Abkürzung im Suchbaum public override string Abbreviation => "Weighting"; //description public override string HelpDescription => "Mix 2 indicators with an individual weighting factor. Each indicator is scaled from 0-100% before by 'PctRank'"; //price pane public override string PaneTag => "WGHT"; //name - Name im Pane wo Indikator beschrieben ist public override string Name => "Weighting Indicators"; //default color public override WLColor DefaultColor => WLColor.Brown; //default plot style public override PlotStyle DefaultPlotStyle => PlotStyle.Line; //generate parameters protected override void GenerateParameters() { AddParameter("Source", ParameterType.BarHistory, null); AddParameter("IndicatorSource1", ParameterType.IndicatorTSSource, ParameterType.IndicatorTSSource); AddParameter("WeightSource1", ParameterType.Double, 0.5); AddParameter("IndicatorSource2", ParameterType.IndicatorTSSource, ParameterType.IndicatorTSSource); AddParameter("LookbackPctRank", ParameterType.Int32, 90); } //populate public override void Populate() { BarHistory source = Parameters[0].AsBarHistory; TimeSeries indicatorSource1 = Parameters[1].AsTimeSeries; double weightSource1 = Parameters[2].AsDouble; TimeSeries indicatorSource2 = Parameters[3].AsTimeSeries; double weightSource2 = 1 - weightSource1; Int32 lookbackPctRank = Parameters[4].AsInt; DateTimes = source.DateTimes; //calculate prct rank TimeSeries prctRankIndicator1 = new PctRank(indicatorSource1, lookbackPctRank); TimeSeries prctRankIndicator2 = new PctRank(indicatorSource2, lookbackPctRank); //calculate combined weight for (int idx = lookbackPctRank; idx < source.Count; idx++) { Values[idx] = weightSource1 * prctRankIndicator1[idx] + weightSource2 * prctRankIndicator2[idx]; } } } }
When dragging the indicator on a chart the window looks like screenshot 1 (and "WGHT" pane on chart remains empty). What is the correct syntax that it works like screenshot 2?
Rename
Before anything else, it looks like you could use MathIndOpInd with "Add" as operation, taking MathIndOpValue for both Indicator1 and Indicator2? Where the Indicator1 and Indicator2 would be another (nested) MathIndOpValue, multiplying the PctRank by a weight source.
Hi Eugene, thanks for response. Basically you are right, but this is not the solution for my problem. I want to add an indicator as parameter. For later investigations with e.g. "Analysis Series" I want to change the indicators.
So what is the correct method for adding indicators as parameter and how to access the timeseries of the parameter?
So what is the correct method for adding indicators as parameter and how to access the timeseries of the parameter?
CODE:
//generate parameters protected override void GenerateParameters() { //which method? AddIndicatorParameter("SMA"); AddParameter("IndicatorSource1", ParameterType.Indicator, ???); AddParameter("IndicatorSource1", ParameterType.IndicatorSource, ???); AddParameter("IndicatorSource1", ParameterType.IndicatorTSSource, ???); } //populate public override void Populate() { //how to access timeseries of selected indicator? TimeSeries indicatorSource1 = Parameters[0].AsTimeSeries; }
Here is the correct syntax.
There are a few things to note,
1) Add the assignments to IndicatorAbbreviation, IndicatorParameters, and IndicatorInstance in the constructor.
2) Use the helper method AddIndicatorParameter in the GenerateParameters.
3) In Populate, extract the IndicatorAbbreviation and IndicatorParameters, then call IndicatorFactory.Instance.CreateIndicator to create the indicator's instance.
There are a few things to note,
1) Add the assignments to IndicatorAbbreviation, IndicatorParameters, and IndicatorInstance in the constructor.
2) Use the helper method AddIndicatorParameter in the GenerateParameters.
3) In Populate, extract the IndicatorAbbreviation and IndicatorParameters, then call IndicatorFactory.Instance.CreateIndicator to create the indicator's instance.
CODE:
using WealthLab.Core; using WealthLab.Indicators; using System; namespace WealthLabCustom { public class Weighting : IndicatorBase { public Weighting() : base() { LibraryName = "RiMarBo"; } //for code based construction public Weighting(BarHistory barHistory, IndicatorBase indicatorSource1, double weightSource1, IndicatorBase indicatorSource2, int lookbackPctRank) : base() { LibraryName = "RiMarBo"; Parameters[0].Value = barHistory; Parameters[1].Value = indicatorSource1; Parameters[1].IndicatorInstance = indicatorSource1; Parameters[1].IndicatorAbbreviation = indicatorSource1.Abbreviation; Parameters[1].IndicatorParameters = indicatorSource1.Parameters; Parameters[2].Value = weightSource1; Parameters[3].Value = indicatorSource2; Parameters[3].IndicatorInstance = indicatorSource2; Parameters[3].IndicatorAbbreviation = indicatorSource2.Abbreviation; Parameters[3].IndicatorParameters = indicatorSource2.Parameters; Parameters[4].Value = lookbackPctRank; Populate(); } //abbreviation - Abkürzung im Suchbaum public override string Abbreviation => "Weighting"; //description public override string HelpDescription => "Mix 2 indicators with an individual weighting factor. Each indicator is scaled from 0-100% before by 'PctRank'"; //price pane public override string PaneTag => "WGHT"; //name - Name im Pane wo Indikator beschrieben ist public override string Name => "Weighting Indicators"; //default color public override WLColor DefaultColor => WLColor.Brown; //default plot style public override PlotStyle DefaultPlotStyle => PlotStyle.Line; //generate parameters protected override void GenerateParameters() { AddParameter("Source", ParameterType.BarHistory, null); AddIndicatorParameter("IndicatorSource1"); AddParameter("WeightSource1", ParameterType.Double, 0.5); AddIndicatorParameter("IndicatorSource2"); AddParameter("LookbackPctRank", ParameterType.Int32, 90); } //populate public override void Populate() { BarHistory source = Parameters[0].AsBarHistory; string indName = Parameters[1].IndicatorAbbreviation; ParameterList indParams = Parameters[1].IndicatorParameters; IndicatorBase ind1 = IndicatorFactory.Instance.CreateIndicator(indName, indParams, source); double weightSource1 = Parameters[2].AsDouble; indName = Parameters[3].IndicatorAbbreviation; indParams = Parameters[3].IndicatorParameters; IndicatorBase ind2 = IndicatorFactory.Instance.CreateIndicator(indName, indParams, source); double weightSource2 = 1 - weightSource1; Int32 lookbackPctRank = Parameters[4].AsInt; DateTimes = source.DateTimes; //calculate prct rank TimeSeries prctRankIndicator1 = new PctRank(ind1, lookbackPctRank); TimeSeries prctRankIndicator2 = new PctRank(ind2, lookbackPctRank); //calculate combined weight for (int idx = lookbackPctRank; idx < source.Count; idx++) { Values[idx] = weightSource1 * prctRankIndicator1[idx] + weightSource2 * prctRankIndicator2[idx]; } } } }
Yes, that works! Thank you very much Glitch!
You’re welcome! 🫡
I came across this post while searching for an example of how to use IndicatorSource. There are now several ways to use nested indicators. Are there certain scenarios when I should prefer one approach over another? For example, when is it better to derive a custom indicator from TransformerBase or to write the code as in this example. Or am I confusing two different things here?
Your Response
Post
Edit Post
Login is required