I have a customized Entry Building Block. Running the block with strategy evolver works fine. Evolver is collecting the apex strategies. After stopping the process I can open the strategies in evolver tab. But when opening an apex strategy, the parameters of entry block are missing. Can you give me a hint without the customized code of the block?
Open strategy from evolver tab:
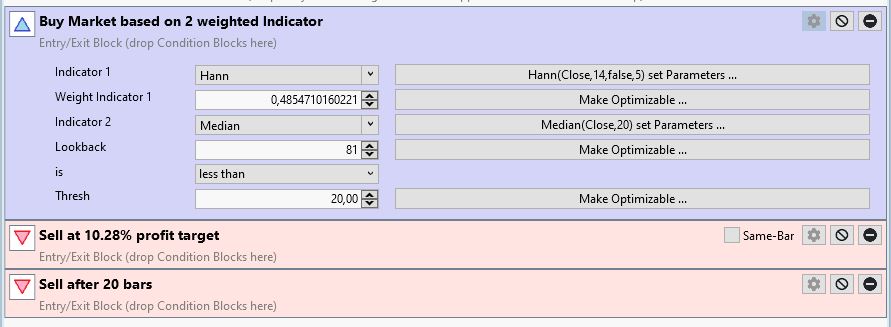
Open strategy from apex strategies:
Open strategy from evolver tab:
Open strategy from apex strategies:
Rename
They look like two different strategies. They have different exit blocks even.
it I’ll take a look and see if there’s any work to be done in apex strategies.
it I’ll take a look and see if there’s any work to be done in apex strategies.
I have made another test, same bad result: Opened Apex strategy has lost parameters. - I hope you can reproduce it.
Code for Entry Building Block:
Gene Code:
In Gene Roster following 2 items are checked: Entry block and "Sell after N bars". Test data are WD DOW 30, last 5 years. Position size 7% of equity, starting capital 100 000.
RESULTS:
Evolver (opening strategy from Evolver works fine):
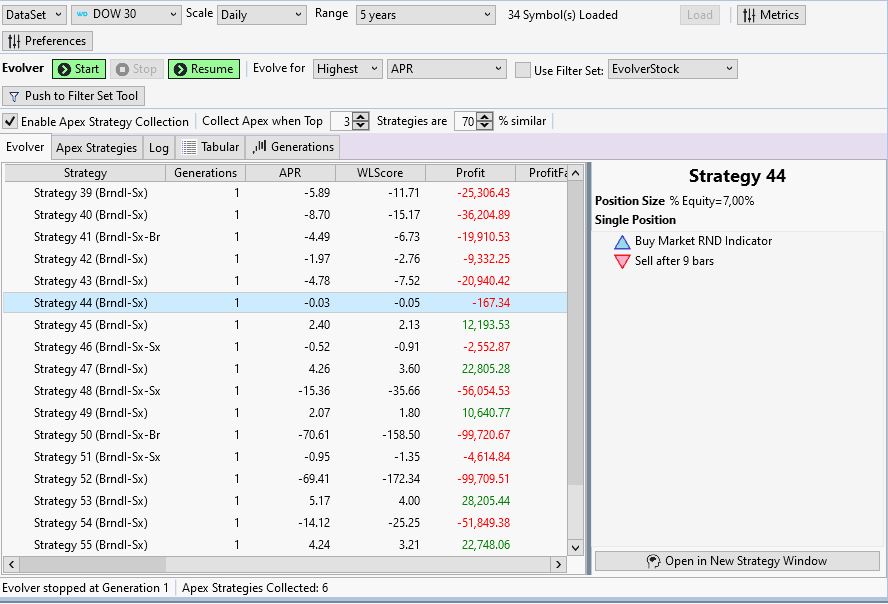
Opened Evolver strategy:
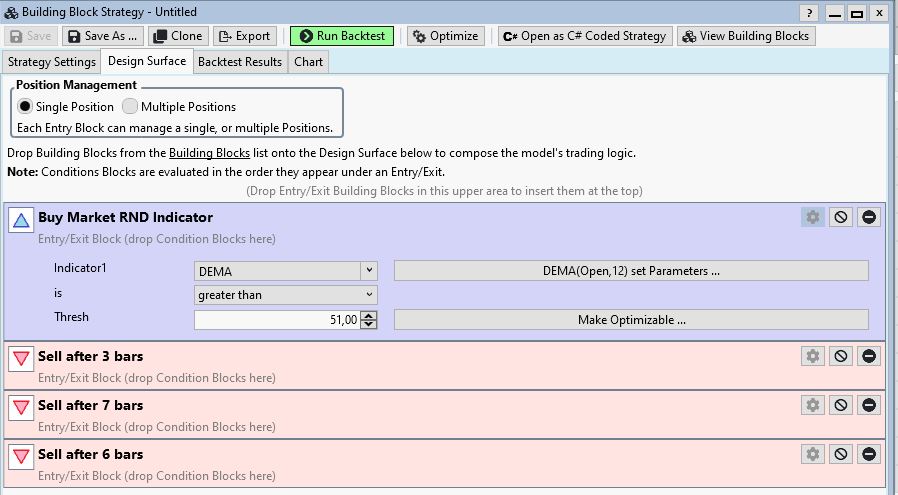
APEX strategies (opening strategies here, parameters are lost):
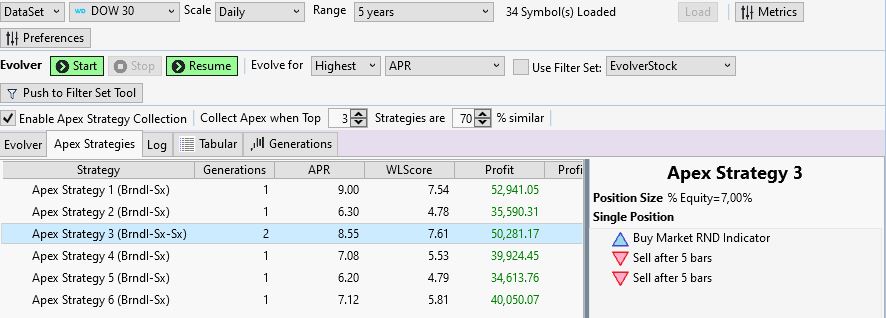
Opened Apex strategy:
Code for Entry Building Block:
CODE:
using WealthLab.Backtest; using WealthLab.Core; namespace WealthLabCustom { public class BuyMarketOneIndicator : EntryExitBuildingBlock { public override string Name => "Buy Market RND Indicator"; public override bool IsEntry => true; public override bool IsExit => false; public override PositionType? PositionType => WealthLab.Backtest.PositionType.Long; //parameters public override void GenerateParameters() { AddIndicatorParameter("Indicator1", "SMA");//0 AddValueCompareParameter("is", "greater than");//1 AddParameter("Thresh", ParameterType.Double, 10);//2 } //indicators public override void Initialize() { //register variable RegisterVariable("indicator1", "IndicatorBase"); RegisterVariable("indicatorRatio", "TimeSeries"); } //generate init code public override void GenerateInitCode() { GenerateIndicatorInitCode(0, "indicator1"); InitCode.Add("\t<indicatorRatio> = <indicator1> / bars.Close - 1;"); } //code in execute section public override void GenerateMainCode() { string compareOp = Parameters[1].AsCompareOperation; string thres = Convert.ToString(Parameters[2].AsDouble / 10000); thres = thres.Replace(',', '.'); MainCode.Add("if (<indicatorRatio>[idx] " + compareOp + " " + thres + ")"); MainCode.Add("{"); MainCode.Add("\t_transaction = PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0," + GroupCode + ");"); MainCode.Add("}"); base.GenerateMainCode(); } //associate building block with strategy gene public override StrategyGeneBase GetGene() { BuyMarketOneIndicatorGene buyMarketOneIndicatorGene = new BuyMarketOneIndicatorGene(); buyMarketOneIndicatorGene.IndicatorAbbreviation1 = Parameters[0].IndicatorAbbreviation; buyMarketOneIndicatorGene.IndicatorParameters1 = Parameters[0].IndicatorParameters; buyMarketOneIndicatorGene.AboveOrBelow = (AboveBelow)Parameters[1].Value; buyMarketOneIndicatorGene.Thresh = (double)Parameters[3].Value; return buyMarketOneIndicatorGene; } } }
Gene Code:
CODE:
using WealthLab.Backtest; using WealthLab.Core; using WealthLab.Indicators; using WealthLabCustom.StrategyGenes; namespace WealthLabCustom { public class BuyMarketOneIndicatorGene : StrategyGeneBase { //gene name public override string Name => "Buy Market when Close < RND indicator"; //buy simple public override string ShortCode => "BrndI"; public override bool IsEntry => true; public override bool IsExit => false; //Genes to randomize public string IndicatorAbbreviation1 { get; set; } = ""; public ParameterList IndicatorParameters1 { get; set; } = new ParameterList(); public AboveBelow AboveOrBelow { get; set; } public double Thresh { get; set; } //randomize public override void Randomize() { IndicatorBase ind1 = IndicatorFactory.Instance.Smoothers.RandomValue; IndicatorAbbreviation1 = ind1.Abbreviation; IndicatorParameters1 = ind1.Parameters.Clone(); RandomizeParameters(IndicatorParameters1); AboveOrBelow = RNG.NextDouble() > 0.5 ? AboveBelow.Above : AboveBelow.Below; Thresh = Convert.ToDouble(RNG.Next(120)); } //No Generation 2 possible? /*public override void Mutate(GeneticStrategy gs) { if (RNG.NextDouble() > 0.25) { AboveOrBelow = AboveOrBelow.Opposite(); } }*/ //create building block public override BuildingBlockBase GetBuildingBlock() { BuyMarketOneIndicator buyMarketOneIndicator = new BuyMarketOneIndicator(); buyMarketOneIndicator.Parameters[0].IndicatorAbbreviation = IndicatorAbbreviation1; buyMarketOneIndicator.Parameters[0].IndicatorParameters = IndicatorParameters1; buyMarketOneIndicator.Parameters[1].Value = AboveOrBelow.AsLessThanGreaterThan(); buyMarketOneIndicator.Parameters[2].Value = Thresh; return buyMarketOneIndicator; } } }
In Gene Roster following 2 items are checked: Entry block and "Sell after N bars". Test data are WD DOW 30, last 5 years. Position size 7% of equity, starting capital 100 000.
RESULTS:
Evolver (opening strategy from Evolver works fine):
Opened Evolver strategy:
APEX strategies (opening strategies here, parameters are lost):
Opened Apex strategy:
Opening an Apex strategy isn't different from regular strategies. You're using a custom indicator/strategy library. Perhaps there's an oversight in the Random indicator value code?
It looks like your customized buiklding blocks might not be coded correctly or completely.
Look at the Sell after N Bars and Sell at XX% Profit Target Blocks that you opened from the Apex, those parameters did carry over.
Look at the Sell after N Bars and Sell at XX% Profit Target Blocks that you opened from the Apex, those parameters did carry over.
You are right, but "Sell after N bars" is here a standard WL-block. I don't know, but for me there is obviously a difference in opening Evolver/Apex strategies.
Randomizing the indicator:
then calling the method for building the block:
Calling it from Apex it looks like, that indicator's abbrevation is empty. But why is it different when calling it by Evolver?
Randomizing the indicator:
CODE:
IndicatorBase ib = IndicatorFactory.Instance.Smoothers.RandomValue; while (ib.Abbreviation.Equals("")) { ib = IndicatorFactory.Instance.Smoothers.RandomValue; } IndicatorAbbreviation1 = ib.Abbreviation; IndicatorParameters1 = ib.Parameters.Clone(); RandomizeParameters(IndicatorParameters1);
then calling the method for building the block:
CODE:
public override BuildingBlockBase GetBuildingBlock() { BuyMarketOneIndicator buyMarketOneIndicator = new BuyMarketOneIndicator(); if (IndicatorAbbreviation1 != "") { buyMarketOneIndicator.Parameters[0].IndicatorAbbreviation = IndicatorAbbreviation1; buyMarketOneIndicator.Parameters[0].IndicatorParameters = IndicatorParameters1; buyMarketOneIndicator.Parameters[1].Value = AboveOrBelow.AsLessThanGreaterThan(); buyMarketOneIndicator.Parameters[2].Value = 0815; } else { buyMarketOneIndicator.Parameters[0].IndicatorAbbreviation = PriceComponent.AveragePriceHL.ToString(); buyMarketOneIndicator.Parameters[1].Value = AboveOrBelow.AsLessThanGreaterThan(); buyMarketOneIndicator.Parameters[2].Value = 1015; } return buyMarketOneIndicator; }
Calling it from Apex it looks like, that indicator's abbrevation is empty. But why is it different when calling it by Evolver?
>>You are right, but "Sell after N bars" is here a standard WL-block.<<
That's my point. It's operating correctly for all of the standard Blocks that we created.
If I had to guess I would say your custom Genes don't implement (or implement incorrectly) the GeneData get/set methods.
That's my point. It's operating correctly for all of the standard Blocks that we created.
If I had to guess I would say your custom Genes don't implement (or implement incorrectly) the GeneData get/set methods.
Ok, now I have got it. For getting the parameters in Apex strategies they have to be stored in class GeneData-property.
Thanks for the hint!
CODE:
public override string GeneData
Thanks for the hint!
Your Response
Post
Edit Post
Login is required