Hello guys
I am trying to place an order every time the candle closure is 0.5% higher than my last open position, but I'm strugling with an error.
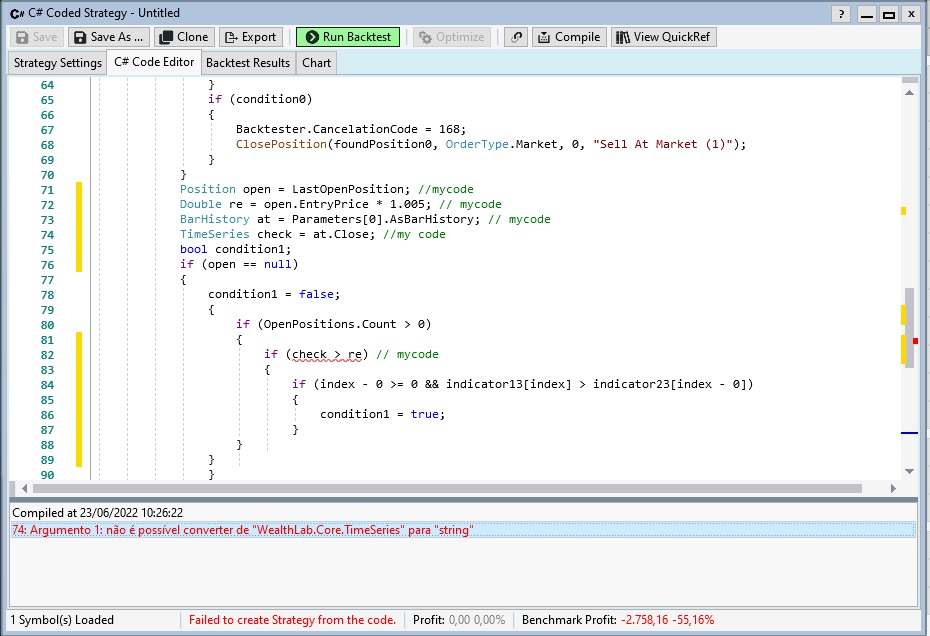
I am trying to place an order every time the candle closure is 0.5% higher than my last open position, but I'm strugling with an error.
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Indicators; using System.Collections.Generic; using WealthLab.MyIndicators; namespace WealthScript4 { public class MyStrategy : UserStrategyBase { public MyStrategy() : base() { } public override void Initialize(BarHistory bars) { indicator1 = new CustomSMA(bars.Close,7); PlotIndicator(indicator1,new WLColor(0,0,0)); indicator1.MassageColors = true; indicator2 = new CustomSMA(bars.Close,14); PlotIndicator(indicator2,new WLColor(0,0,255)); indicator2.MassageColors = true; indicator12 = new CustomSMA(bars.Close,7); indicator22 = new CustomSMA(bars.Close,14); indicator13 = new CustomSMA(bars.Close,7); indicator23 = new CustomSMA(bars.Close,14); StartIndex = 14; } public override void Execute(BarHistory bars, int idx) { int index = idx; Position foundPosition0 = FindOpenPosition(0); bool condition0; if (foundPosition0 == null) { condition0 = false; { if (index - 0 >= 0 && indicator1[index] > indicator2[index - 0]) { if (OpenPositions.Count == 0) { condition0 = true; } } } if (condition0) { _transaction = PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0, 0, "Buy At Market (1)"); } } else { condition0 = false; { if (index - 0 >= 0 && indicator12[index] < indicator22[index - 0]) { condition0 = true; } } if (condition0) { Backtester.CancelationCode = 168; ClosePosition(foundPosition0, OrderType.Market, 0, "Sell At Market (1)"); } } Position open = LastOpenPosition; //mycode Double re = open.EntryPrice * 1.005; // mycode BarHistory at = Parameters[0].AsBarHistory; // mycode TimeSeries check = at.Close; //my code bool condition1; if (open == null) { condition1 = false; { if (OpenPositions.Count > 0) { if (check > re) // mycode { if (index - 0 >= 0 && indicator13[index] > indicator23[index - 0]) { condition1 = true; } } } } if (condition1) { _transaction = PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0, 1, "Buy At Market (2)"); } } else { } } public override void NewWFOInterval(BarHistory bars) { indicator1 = new CustomSMA(bars.Close,7); indicator2 = new CustomSMA(bars.Close,14); indicator12 = new CustomSMA(bars.Close,7); indicator22 = new CustomSMA(bars.Close,14); indicator13 = new CustomSMA(bars.Close,7); indicator23 = new CustomSMA(bars.Close,14); } private IndicatorBase indicator1; private IndicatorBase indicator2; private IndicatorBase indicator12; private IndicatorBase indicator22; private IndicatorBase indicator13; private IndicatorBase indicator23; private Transaction _transaction; } }
Rename
check is a TimeSeries. To get the value of a TimeSeries (or Indicator) at the current bar, you have to use an indexer like this -
idx is the current bar, idx - 1 is the previous bar, idx - 2 is 2 bars ago, etc.
CODE:
if (check[idx] > re) // mycode
idx is the current bar, idx - 1 is the previous bar, idx - 2 is 2 bars ago, etc.
Thank, I think this part of the code is working, as well I finally discovered what idx stands for...
But it seem that I have new errors to deal with:
But it seem that I have new errors to deal with:
These type of questions will go on many more times unless you understand what you're programming. You've added code "outside" the "if/else" block for foundPosition0 .. why, exactly? I cannot tell.
But to answer this specific error, objects, like a Position object that's returned from LastOpenPosition, can have a null value. When they do, you can't use them to access their properties or methods, like you did on line 72. If there's a chance of a null, you have to check for it ...
But to answer this specific error, objects, like a Position object that's returned from LastOpenPosition, can have a null value. When they do, you can't use them to access their properties or methods, like you did on line 72. If there's a chance of a null, you have to check for it ...
CODE:
Position open = LastOpenPosition; if (open == null) return; // nothing you can do with open if it's null double re - open.EntryPrice * 1.005;
I can not tell either... that came from the strategy constructor..
If there's a problem with auto-generated block code, let us know before you modify it ;)
I can see there was an open == null check already, but you added code above that statement - and below it (the other error with check).
I can see there was an open == null check already, but you added code above that statement - and below it (the other error with check).
I managed to make it work!!!
Thak you very much for the help ;)
Thak you very much for the help ;)
Your Response
Post
Edit Post
Login is required