I am trying to get wealthlab to get entry dates and stocks from an excel sheet to then try out different sell strategies.
The problem is that I am clearly not understanding how bars.DateTimes[idx].Date works because the strategy buys whenever it wants rather than when bars.DateTimes[idx].Date is within 10 days of the date on the excel (screen shot below)
So here is a screen shot of the csv file filtered for the ticker 'ABR'
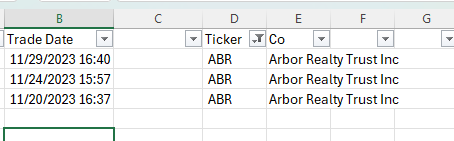
And here are the resulting trades for ticker "ABR'
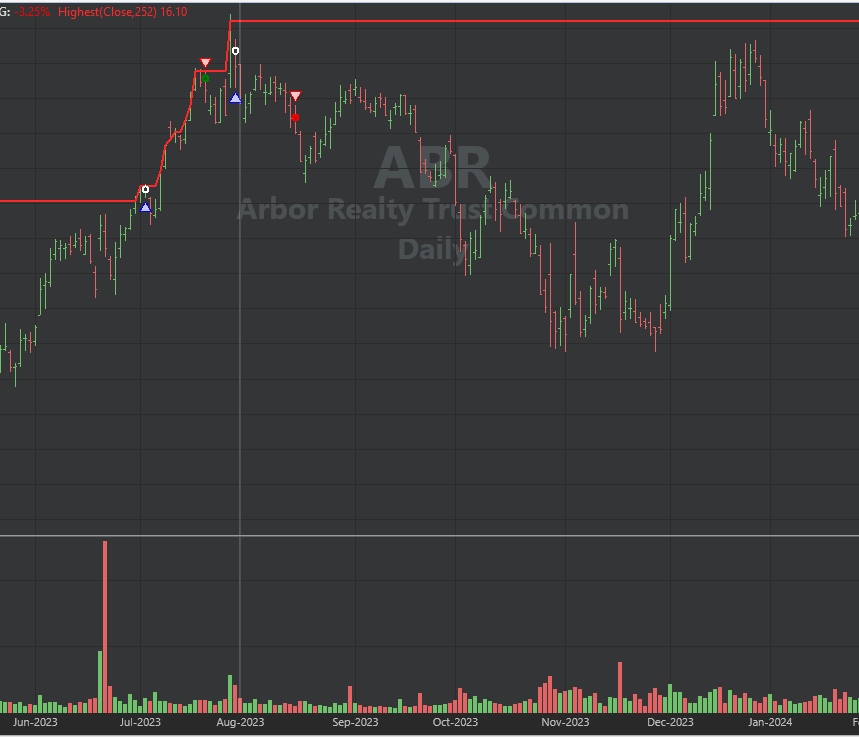
The trades are ocurring at the 52 week high but NOT within 10 days of the other signal.
Help with what I am doing wrong would be much appreciated.
Thank you in advance.
The problem is that I am clearly not understanding how bars.DateTimes[idx].Date works because the strategy buys whenever it wants rather than when bars.DateTimes[idx].Date is within 10 days of the date on the excel (screen shot below)
CODE:
using WealthLab.Backtest; using System; using System.IO; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; using System.Linq; using Steema.TeeChart.Functions; using SixLabors.ImageSharp; using Alternet.Editor.Wpf; //using DocumentFormat.OpenXml.Drawing.Diagrams; //using DocumentFormat.OpenXml.Wordprocessing; namespace WealthScript1 { public class MyStrategy : UserStrategyBase { //create indicators and other objects here, this is executed prior to the main trading loop private Dictionary<int, Tuple<string, DateTime>> data; Highest highest_s; public override void Initialize(BarHistory bars) { StartIndex = 60; data = func_openfile_var(); highest_s = new Highest(bars.Close, 252); PlotIndicator(highest_s, WLColor.Red, PlotStyle.Line); } //execute the strategy rules here, this is executed once for each bar in the backtest history public override void Execute(BarHistory bars, int idx) { // Get data from spreadsheet if (!HasOpenPosition(bars, PositionType.Long)) { //code your buy conditions here if ( func_purchase_date_bool(data, bars.Symbol, bars.DateTimes[idx].Date) && bars.Close[idx] > highest_s[idx - 1] ) { PlaceTrade(bars, TransactionType.Buy, OrderType.Market, 0, "Buy"); } } else { //code your sell conditions here Position p = LastPosition; if (idx - p.EntryBar > 10) { ClosePosition(p, OrderType.Market, 0, "TimeOut"); } } } //declare private variables below public static Dictionary<int, Tuple<string, DateTime>> func_openfile_var() { string file_loc_str = @"C:\Users\gasto\Sync\Nanodegree\Finance\Data"; string file_name_str = "purchases.csv"; string full_path_str = Path.Combine(file_loc_str, file_name_str); var data = File.ReadLines(full_path_str).Skip(1) .Select((line, index) => new { Line = line.Split(','), LineNumber = index }) .ToDictionary(x => x.LineNumber, x => Tuple.Create(x.Line[3].Replace(" ",""), DateTime.Parse(x.Line[1]).Date)); return data; } static bool func_purchase_date_bool(Dictionary<int, Tuple<string, DateTime>> data, string searchString, DateTime currentDate) { return data.Any(item => item.Value.Item1.Equals(searchString) && (currentDate - item.Value.Item2.Date).TotalDays <= 10); } } }
So here is a screen shot of the csv file filtered for the ticker 'ABR'
And here are the resulting trades for ticker "ABR'
The trades are ocurring at the 52 week high but NOT within 10 days of the other signal.
Help with what I am doing wrong would be much appreciated.
Thank you in advance.
Rename
You pass bars.DateTimes[idx].Date into the func_purchase_date_bool method. bars.DateTimes[idx].Date is the date at bar number idx. Without seeing the content of your Dictionary, I'm going to guess that dictionary's tuples contains data for a full range of dates both before and after practically any of the dates in bars.DateTimes.
Hence you have:
But currentDate may be less than item.Value.Item2.Date assuming what I stated above. Hence, currentDate - item.Value.Item2.Date can be negative in that case.
Try this...
Hence you have:
CODE:
static bool func_purchase_date_bool(Dictionary<int, Tuple<string, DateTime>> data, string searchString, DateTime currentDate) { return data.Any(item => item.Value.Item1.Equals(searchString) && (currentDate - item.Value.Item2.Date).TotalDays <= 10); }
But currentDate may be less than item.Value.Item2.Date assuming what I stated above. Hence, currentDate - item.Value.Item2.Date can be negative in that case.
Try this...
CODE:
static bool func_purchase_date_bool(Dictionary<int, Tuple<string, DateTime>> data, string searchString, DateTime currentDate) { return data.Any(item => item.Value.Item1.Equals(searchString) && (currentDate - item.Value.Item2.Date).TotalDays <= 10 && (currentDate - item.Value.Item2.Date).TotalDays >= 0); }
Thank you very much!!!..
Your Response
Post
Edit Post
Login is required