Hello there,
EDIT: for TP I noticed you have normal limit orders, just checked the code - and the numbers are always fixed value so that's good, just need help with Stop orders now - how do I allow for some slippage if I choose StopLimit orders?
So I have backtested a simple strategy which turns out to be profitable (image 1), and it includes take profit targets, and stop loss order - at a fixed price% - for example, 10% below the price it got bought at.
However, I know that Binance spot API trading doesn't really allow either of them. Is there any way in C# to mimic these types of orders with limit orders (stop limit and stop buy)? But they have to be based on fixed price target, not relative to candlestick changes - if ETHUSDT got bought at 2500 and SL is 2250, it should stay 2250 ten candlesticks later.
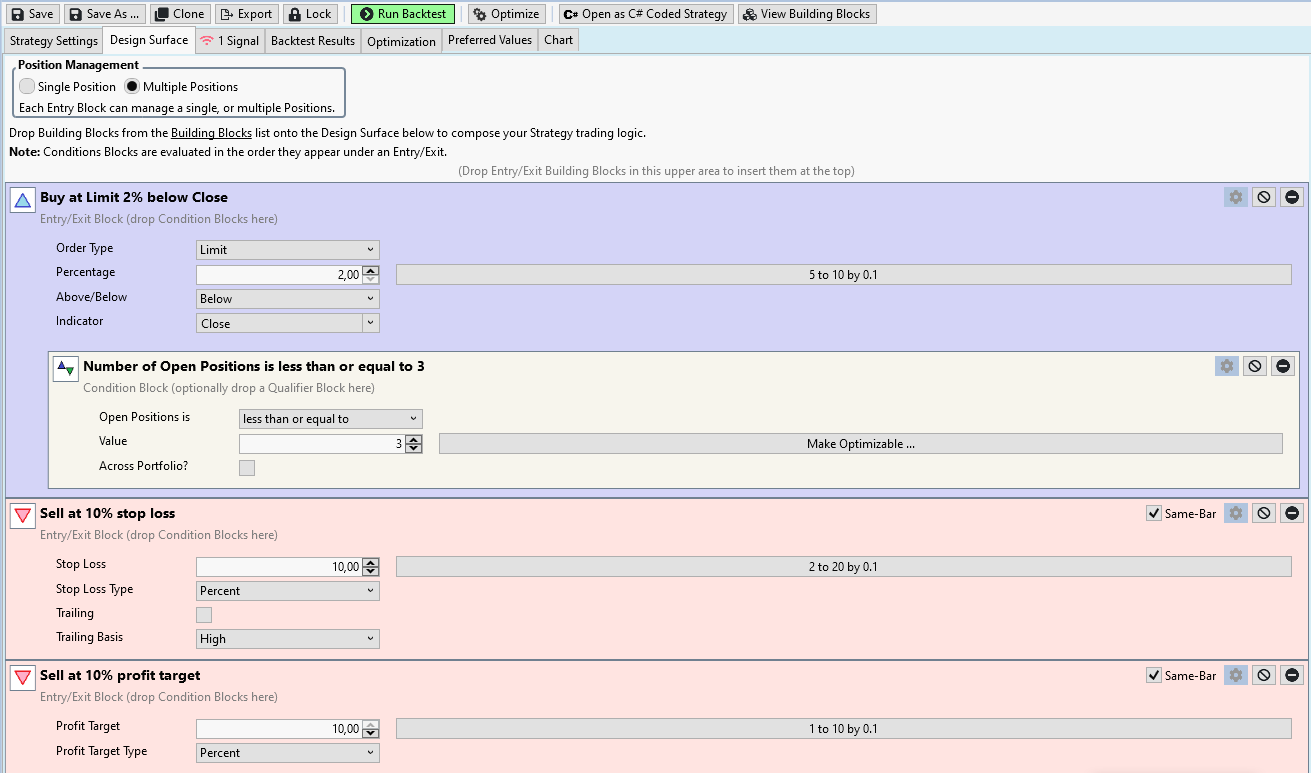
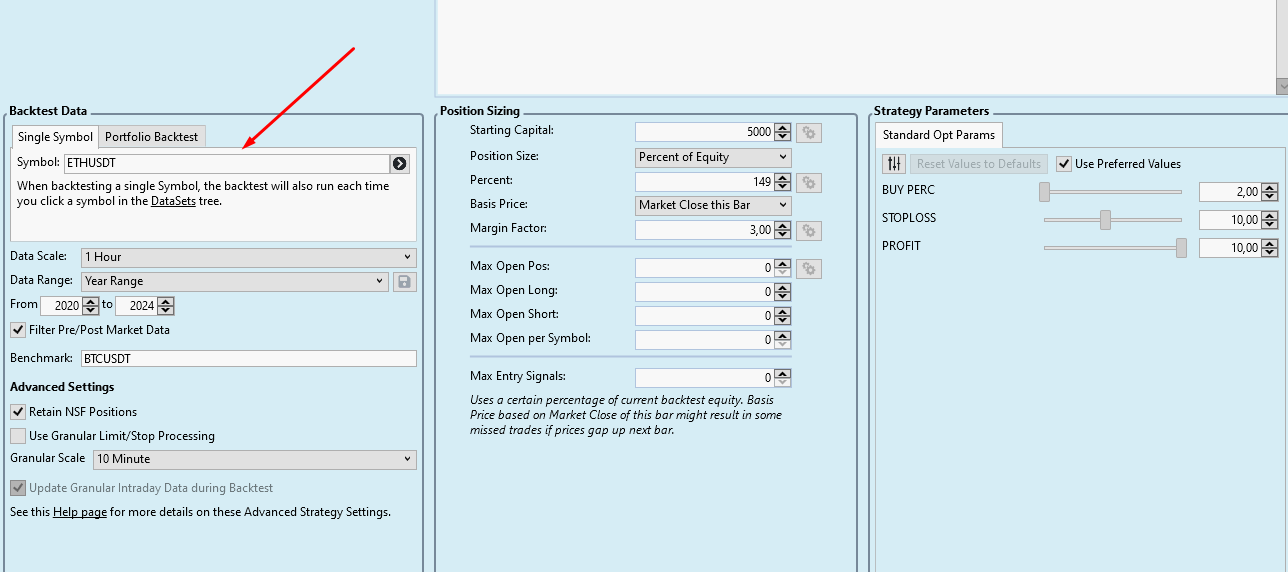
Is it possible to provide an example on how to code this in C#? In next post I'll give the code of the strategy.
EDIT: for TP I noticed you have normal limit orders, just checked the code - and the numbers are always fixed value so that's good, just need help with Stop orders now - how do I allow for some slippage if I choose StopLimit orders?
So I have backtested a simple strategy which turns out to be profitable (image 1), and it includes take profit targets, and stop loss order - at a fixed price% - for example, 10% below the price it got bought at.
However, I know that Binance spot API trading doesn't really allow either of them. Is there any way in C# to mimic these types of orders with limit orders (stop limit and stop buy)? But they have to be based on fixed price target, not relative to candlestick changes - if ETHUSDT got bought at 2500 and SL is 2250, it should stay 2250 ten candlesticks later.
Is it possible to provide an example on how to code this in C#? In next post I'll give the code of the strategy.
Rename
CODE:
using WealthLab.Backtest; using System; using WealthLab.Core; using WealthLab.Data; using WealthLab.Indicators; using System.Collections.Generic; namespace WealthScript2 { public class MyStrategy : UserStrategyBase { public MyStrategy() : base() { AddParameter("BUY PERC", ParameterType.Double, 2, 5, 10, 0.1); AddParameter("STOPLOSS", ParameterType.Double, 10, 2, 20, 0.1); AddParameter("PROFIT", ParameterType.Double, 10, 1, 10, 0.1); StartIndex = 0; } public override void Initialize(BarHistory bars) { source = bars.Close; pct = Parameters[0].AsDouble; pct = (100.0 - pct) / 100.0; multSource = source * pct; PlotStopsAndLimits(3); trailing = false; PlotStopsAndLimits(3); PlotStopsAndLimits(3); foreach(IndicatorBase ib in _startIndexList) if (ib.FirstValidIndex > StartIndex) StartIndex = ib.FirstValidIndex; } public override void Execute(BarHistory bars, int idx) { int index = idx; bool condition0; condition0 = false; { if (OpenPositions.Count <= 3) { condition0 = true; } } if (condition0) { Backtester.CancelationCode = 1; val = multSource[idx]; _transaction = PlaceTrade(bars, TransactionType.Buy, OrderType.Limit, val, 0, "Buy " + Parameters[0].AsDouble + "% below " + source.Description); } { foreach(Position foundPosition0 in OpenPositions) { if (foundPosition0.PositionTag == 0) { Backtester.CancelationCode = 1; value = 1.0 - (Parameters[1].AsDouble / 100.0); ClosePosition(foundPosition0, OrderType.Stop, foundPosition0.EntryPrice * value, "Sell at " + Parameters[1].AsDouble + "% stop loss"); } } } { foreach(Position foundPosition0 in OpenPositions) { if (foundPosition0.PositionTag == 0) { Backtester.CancelationCode = 1; value2 = (Parameters[2].AsDouble / 100.0) + 1.0; ClosePosition(foundPosition0, OrderType.Limit, foundPosition0.EntryPrice * value2, "Sell at " + Parameters[2].AsDouble + "% profit tgt"); } } } } public override void NewWFOInterval(BarHistory bars) { source = bars.Close; pct = Parameters[0].AsDouble; pct = (100.0 - pct) / 100.0; multSource = source * pct; } public override void AssignAutoStopTargetPrices(Transaction t, double basisPrice, double executionPrice) { double price = 0; if (t.PositionTag == 0) { price = executionPrice * (1.0 - Parameters[1].AsDouble / 100.0); t.AssignAutoStopLossPrice(price); } if (t.PositionTag == 0) { price = executionPrice * (Parameters[2].AsDouble / 100.0 + 1.0); t.AssignAutoProfitTargetPrice(price); } } private double pct; private double val; private TimeSeries source; private TimeSeries multSource; private double value; private bool trailing; private double value2; private Transaction _transaction; private List<IndicatorBase> _startIndexList = new List<IndicatorBase>(); } }
Your Response
Post
Edit Post
Login is required